How to Use the Ruby Date.parse Method
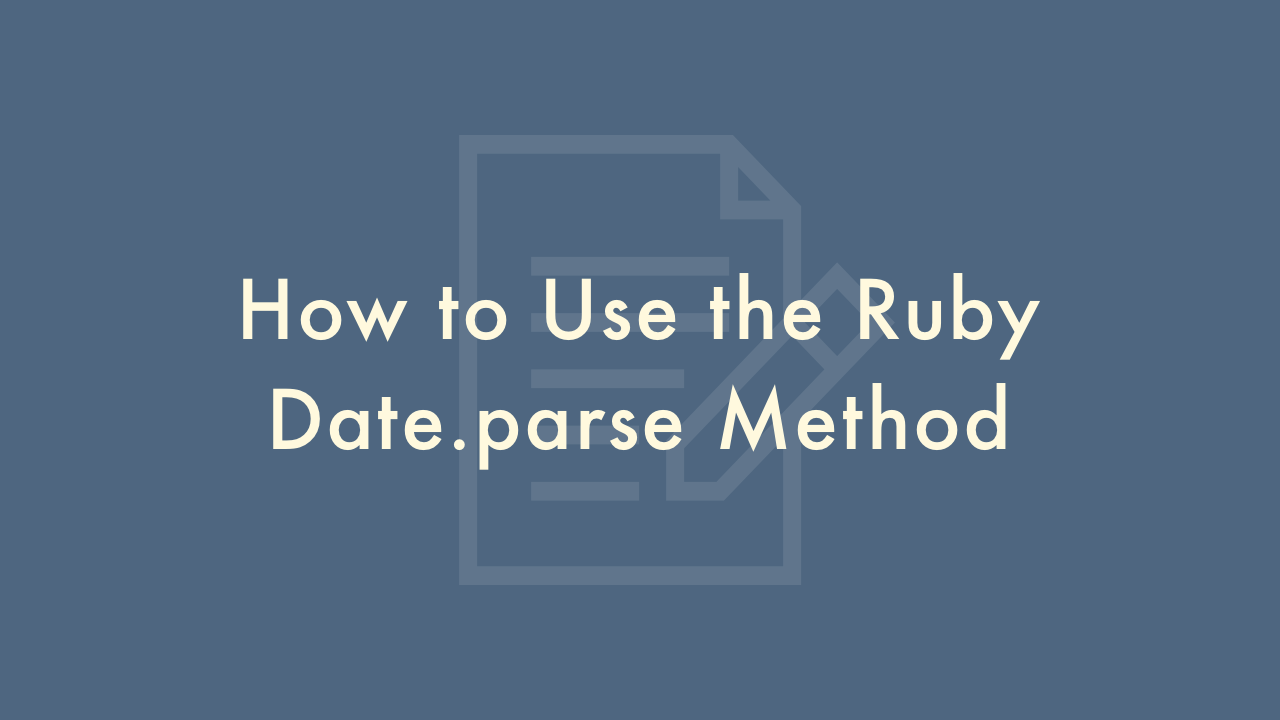
Contents
In this article, you will learn how to use the Ruby Date.parse method.
Using the Date.parse method
Ruby’s Date.parse method is a convenient way to convert date strings into Date objects. The Date.parse method is part of the Ruby standard library, so you don’t need to install any additional gems to use it. Here are some steps to help you use Date.parse effectively:
Require the ‘date’ library
Before using Date.parse, you need to require the ‘date’ library. You can do this by adding the following line at the beginning of your Ruby script:
require 'date'
Pass the date string to Date.parse
Once you’ve required the ‘date’ library, you can use the Date.parse method to convert date strings into Date objects. To use Date.parse, simply pass a date string to the method. The date string can be in any of the following formats:
- YYYY-MM-DD
- YYYY/MM/DD
- MM/DD/YYYY
- DD/MM/YYYY
- Month DD, YYYY (e.g. “January 1, 2022”)
- Month DD YYYY (e.g. “January 1 2022”)
- DD Month YYYY (e.g. “1 January 2022”)
Here’s an example of how to use Date.parse to convert a date string into a Date object:
date_string = "2022-01-01"
date_object = Date.parse(date_string)
Use the Date object
Once you’ve converted the date string into a Date object, you can use it in your Ruby code. Here are some examples of how to use a Date object:
- Get the year: date_object.year
- Get the month: date_object.month
- Get the day: date_object.day
- Get the day of the week: date_object.strftime(“%A”)
- Get the month name: date_object.strftime(“%B”)
Here’s an example that demonstrates how to use a Date object:
date_string = "2022-01-01"
date_object = Date.parse(date_string)
puts "Year: #{date_object.year}"
puts "Month: #{date_object.month}"
puts "Day: #{date_object.day}"
puts "Day of the week: #{date_object.strftime("%A")}"
puts "Month name: #{date_object.strftime("%B")}"
This will output:
Year: 2022
Month: 1
Day: 1
Day of the week: Saturday
Month name: January