How to Use the Ruby unshift Method
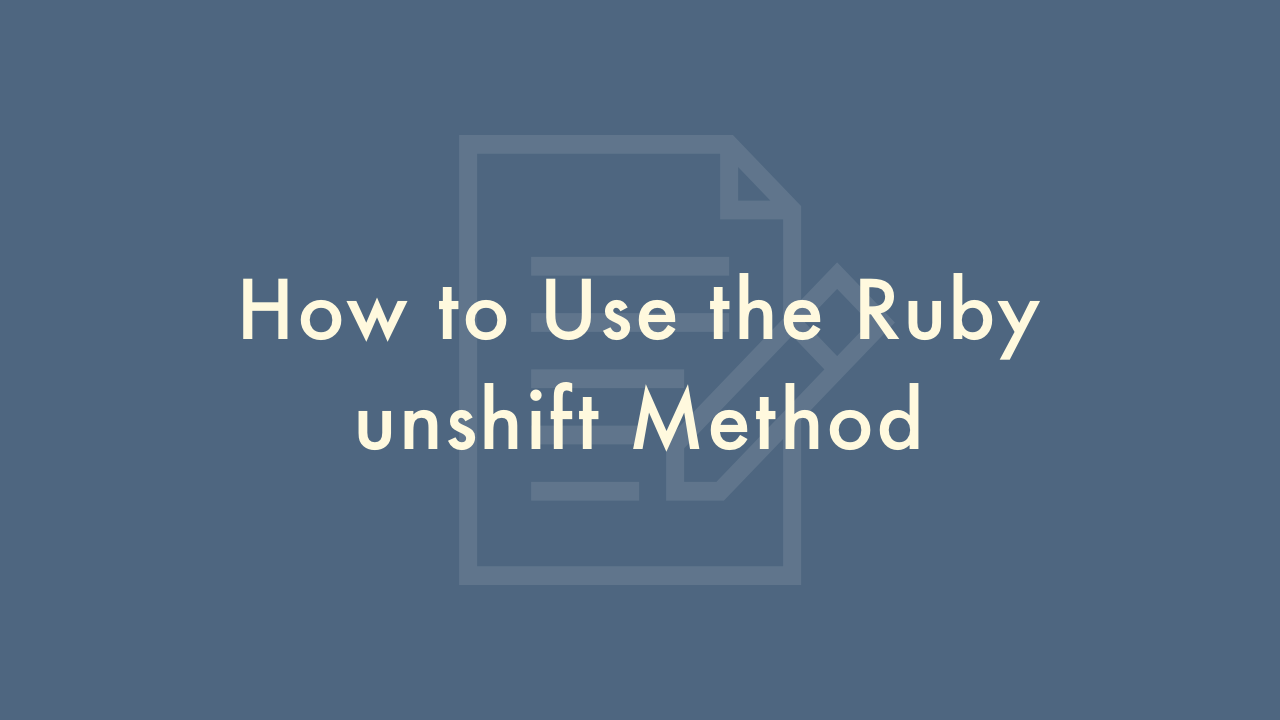
Contents
In this article, you will learn how to use the Ruby unshift method.
Using the unshift method
The unshift method in Ruby is a built-in method that adds one or more elements to the beginning of an array and returns the updated array. This method can be useful in situations where you need to add items to the front of an array without changing the order of existing elements.
Here’s how to use the unshift method in Ruby:
First, create an array that you want to modify using unshift. For example:
fruits = ["apple", "banana", "orange"]
Call the unshift method on the array and pass in one or more arguments to add to the beginning of the array. For example:
fruits.unshift("pear")
This will add the string “pear” to the beginning of the fruits array, and the new array will look like this:
["pear", "apple", "banana", "orange"]
You can also pass in multiple arguments to the unshift method to add multiple elements at once. For example:
fruits.unshift("grape", "pineapple")
This will add the strings “grape” and “pineapple” to the beginning of the fruits array, and the new array will look like this:
["grape", "pineapple", "pear", "apple", "banana", "orange"]
The unshift method returns the updated array, so you can assign it to a new variable or use it in other ways. For example:
new_fruits = fruits.unshift("kiwi")
This will add the string “kiwi” to the beginning of the fruits array and return the updated array, which you can assign to the new variable new_fruits.
You can also use the unshift method with an empty array to create a new array with one or more elements. For example:
new_array = [].unshift("foo", "bar")
This will create a new array with the strings “foo” and “bar” added to the beginning, and the new array will look like this:
["foo", "bar"]