How to Use Ruby prepend
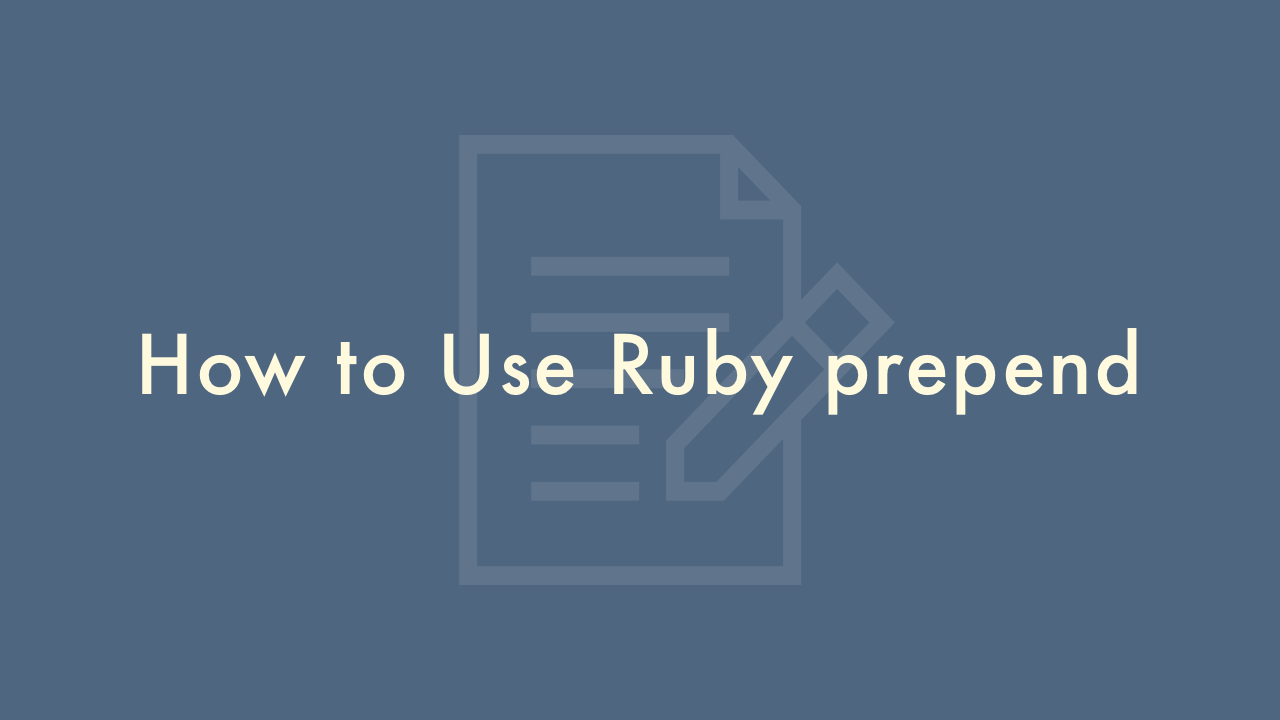
Contents
In this article, you will learn how to use Ruby prepend.
Using Ruby prepend
prepend
is a method in Ruby that allows you to add a module to the inheritance chain of a class or module. The methods defined in the module will become available to the class or module as if they were defined in the class or module itself, but they will take precedence over any existing methods with the same name.
Here is an example of how to use prepend in Ruby:
module MyModule
def greet
puts "Hello from MyModule!"
end
end
class MyClass
prepend MyModule
def greet
puts "Hello from MyClass!"
end
end
my_obj = MyClass.new
my_obj.greet
In this example, we define a module MyModule that defines a greet method. We then define a class MyClass that also defines a greet method. We use the prepend method to add MyModule to the inheritance chain of MyClass, which means that the greet method defined in MyModule takes precedence over the one defined in MyClass.
When we create an instance of MyClass and call the greet method on it, the output will be “Hello from MyModule!” instead of “Hello from MyClass!”. This is because MyModule#greet takes precedence over MyClass#greet due to the use of prepend.
Keep in mind that prepend is only available for modules and classes, and cannot be used with instances of objects. Also, prepend only affects the current class or module and any subclasses or submodules that are defined after the prepend statement. Any existing subclasses or submodules will not be affected by the prepend statement.