How to Parse CSV Files in Ruby
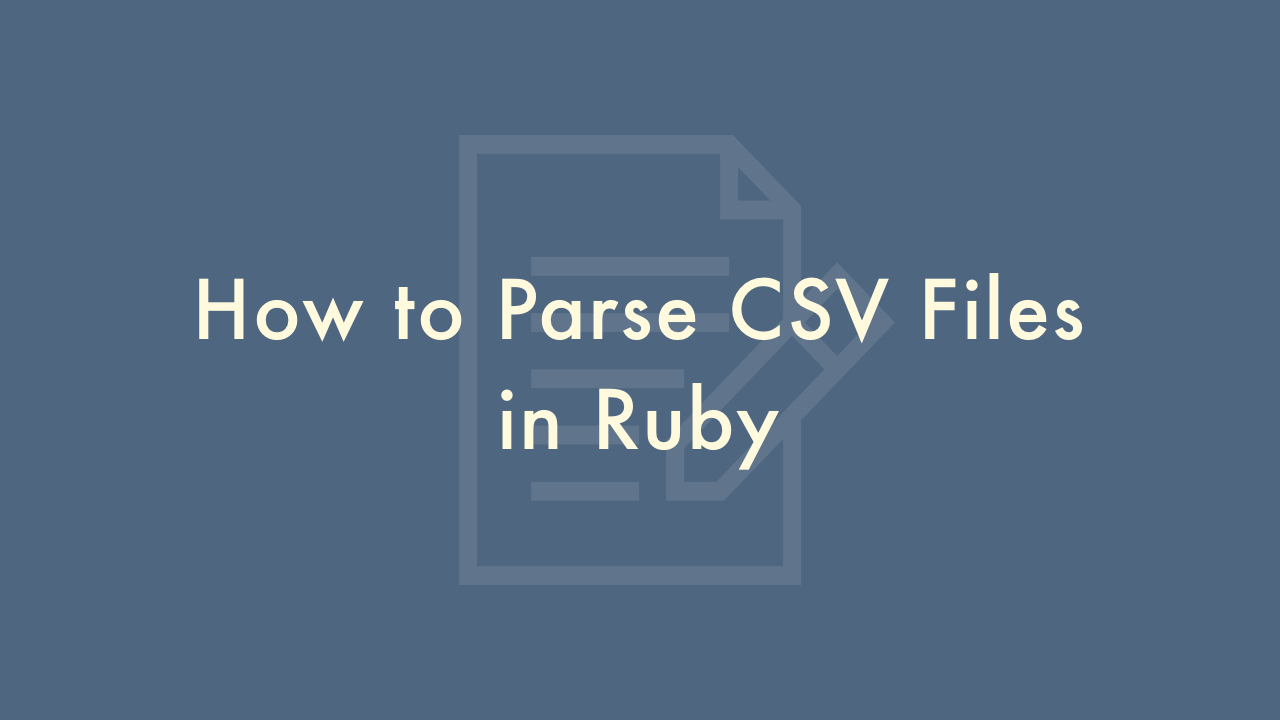
Contents
In this article, you will learn how to parse CSV files in Ruby.
Parsing CSV files
In Ruby, parsing a CSV file can be done using the built-in CSV library. Here is an example of how to parse a CSV file in Ruby:
Require the CSV library:
require 'csv'
Open the CSV file using the CSV.foreach method:
CSV.foreach("filename.csv") do |row|
# Do something with the row
end
The foreach method reads the CSV file line by line and yields each row to the block.
Within the block, you can access each value in the row using array indexing:
CSV.foreach("filename.csv") do |row|
puts row[0] # prints the first value in each row
end
Alternatively, you can use the headers option to treat the first row of the CSV file as a header row:
CSV.foreach("filename.csv", headers: true) do |row|
puts row["column_name"] # prints the value in the "column_name" column of each row
end
Here, row is a CSV::Row object, which allows you to access values by their header names.
If you want to read the entire CSV file into an array, you can use the CSV.read method:
data = CSV.read("filename.csv")
This will return an array of arrays, where each inner array represents a row of the CSV file.
You can also use the CSV.parse method to parse a CSV string:
csv_string = "1,2,3\n4,5,6\n"
data = CSV.parse(csv_string)
This will return the same array of arrays as the CSV.read method.