How to Use the Ruby between? Method
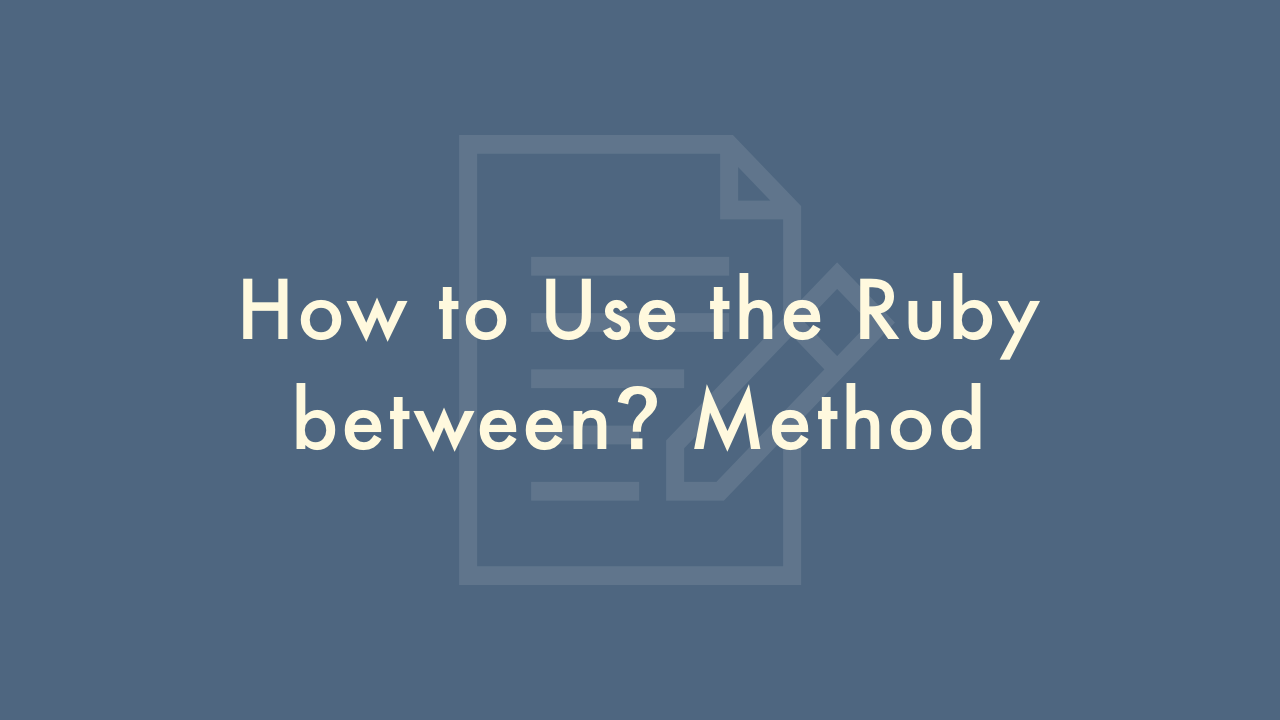
Contents
In this article, you will learn how to use the Ruby between? method.
Using the between? method
The between? method in Ruby is used to determine if a given object falls between two other objects in a range. It returns true if the object is within the range, and false otherwise. The between? method can be called on any object that can be compared using the <=> operator, such as numbers, strings, and dates.
Syntax
The basic syntax for the between? method is as follows:
object.between?(min, max)
Here, object is the object being tested, min is the lower limit of the range, and max is the upper limit of the range. The method returns true if object is greater than or equal to min and less than or equal to max, and false otherwise.
Examples
For example, let’s say we want to check if the number 5 is between 1 and 10. We can use the between? method as follows:
5.between?(1, 10) #=> true
This returns true since 5 is between 1 and 10. Similarly, we can use the between? method to check if a string falls within a certain alphabetical range. For instance, we can check if the string “ruby” falls between “java” and “python” as follows:
"ruby".between?("java", "python") #=> true
This also returns true since “ruby” falls between “java” and “python” alphabetically.
We can also use the between? method with dates. For instance, we can check if a given date falls between two other dates as follows:
require 'date'
date = Date.parse('2020-03-16')
start_date = Date.parse('2020-01-01')
end_date = Date.parse('2020-12-31')
date.between?(start_date, end_date) #=> true
Here, we are using the Date.parse method to convert the date strings into Date objects, and then checking if the date falls between start_date and end_date. This also returns true since date falls between start_date and end_date.