How to Use the Ruby kind_of? Method
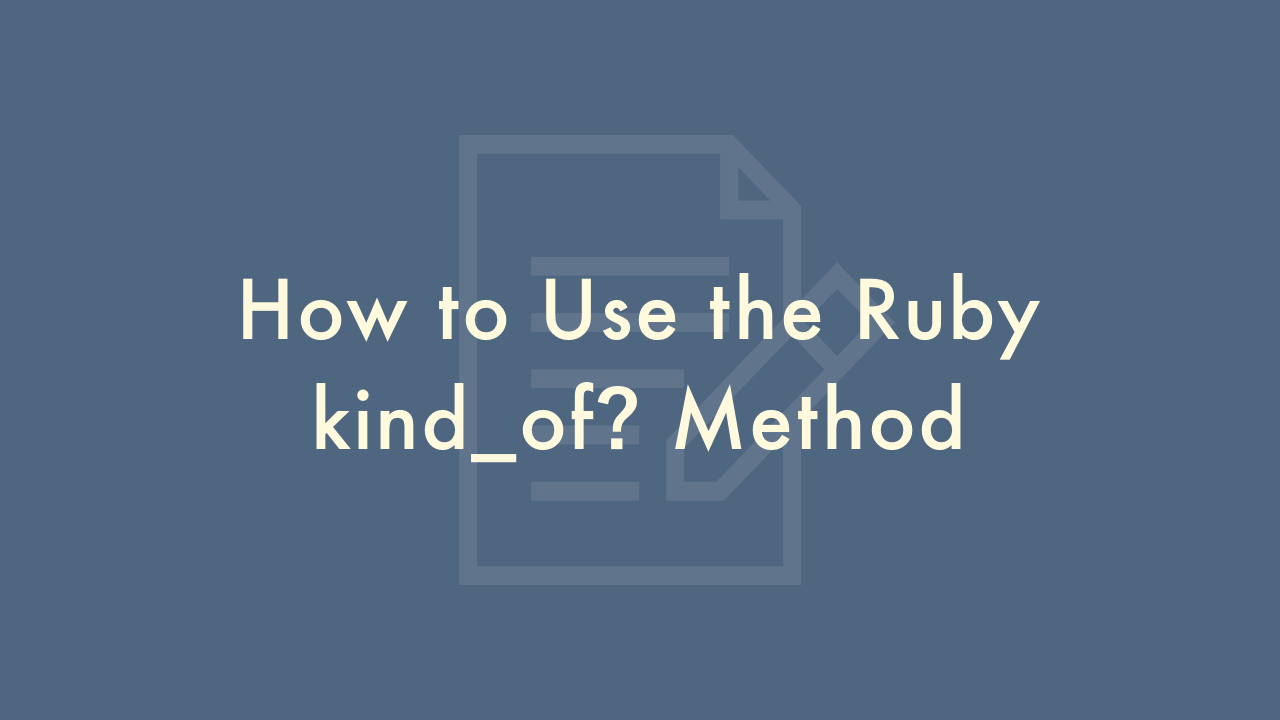
Contents
In this article, you will learn how to use the Ruby kind_of? method.
Using the kind_of? Method
The kind_of? method in Ruby is used to determine whether an object belongs to a particular class or its subclass. It is an instance method of the Object class, which means it can be called on any object in Ruby.
Syntax
The syntax for using the kind_of? method is as follows:
object.kind_of?(class)
where object is the object you want to check and class is the class you want to check against.
The kind_of? method returns true if object belongs to the specified class or any of its subclasses, and false otherwise.
Example
Here is an example of how to use the kind_of? method:
class Animal
end
class Dog < Animal
end
class Cat < Animal
end
my_dog = Dog.new
my_cat = Cat.new
puts my_dog.kind_of?(Dog) # true
puts my_dog.kind_of?(Animal) # true
puts my_dog.kind_of?(Cat) # false
puts my_cat.kind_of?(Dog) # false
puts my_cat.kind_of?(Animal) # true
puts my_cat.kind_of?(Cat) # true
In the example above, we define three classes: Animal, Dog, and Cat. We then create an instance of Dog and Cat and store them in my_dog and my_cat, respectively.
We then use the kind_of? method to check whether my_dog and my_cat belong to certain classes. We can see that my_dog belongs to the Dog and Animal classes, but not the Cat class, while my_cat belongs to the Cat and Animal classes, but not the Dog class.
The kind_of? method is useful when you want to check whether an object belongs to a certain class or not. It is commonly used in conditionals and loops, where you may want to perform different actions depending on the class of an object.