How to Raise Exceptions in Ruby
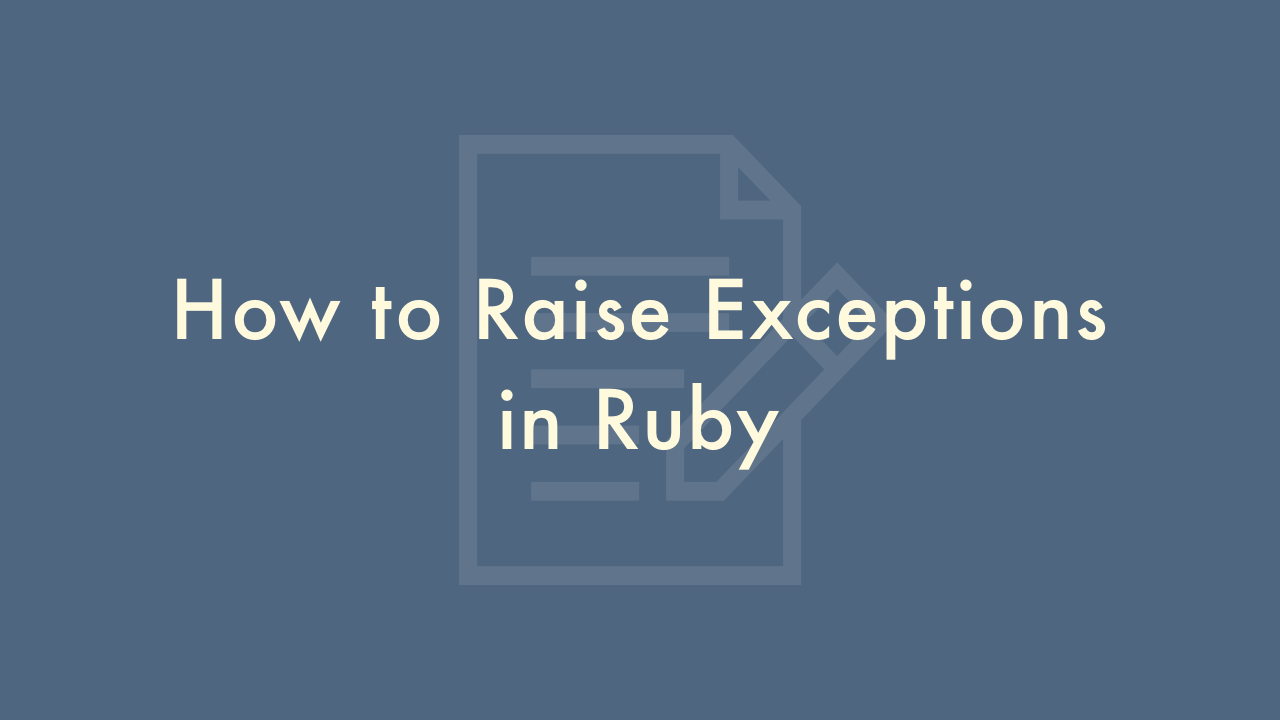
09/21/2021
Contents
In this article, you will learn how to raise exceptions in Ruby.
Raising Exceptions in Ruby
In Ruby, you can raise exceptions using the raise keyword followed by an instance of the Exception class or one of its subclasses. Here are some examples:
Raise a generic Exception
raise Exception.new("Something went wrong.")
Raise a specific exception such as ArgumentError
raise ArgumentError.new("Invalid argument.")
Raise an exception with a custom message
raise "Custom error message."
Raise an exception with a custom message and a specific type
raise TypeError.new("Expected an integer.") if !value.is_a?(Integer)
You can also pass additional arguments to the Exception or its subclass constructor to provide more context about the error. Additionally, you can rescue exceptions using the rescue keyword to handle errors gracefully. Here’s an example:
begin
# some code that might raise an exception
rescue Exception => e
# handle the exception here
puts "An error occurred: #{e.message}"
end
In this example, the rescue block catches any exception that is raised in the begin block and stores it in the variable e. The code inside the rescue block can then handle the exception in some way, such as printing an error message.