How to Use the Ruby dup Method
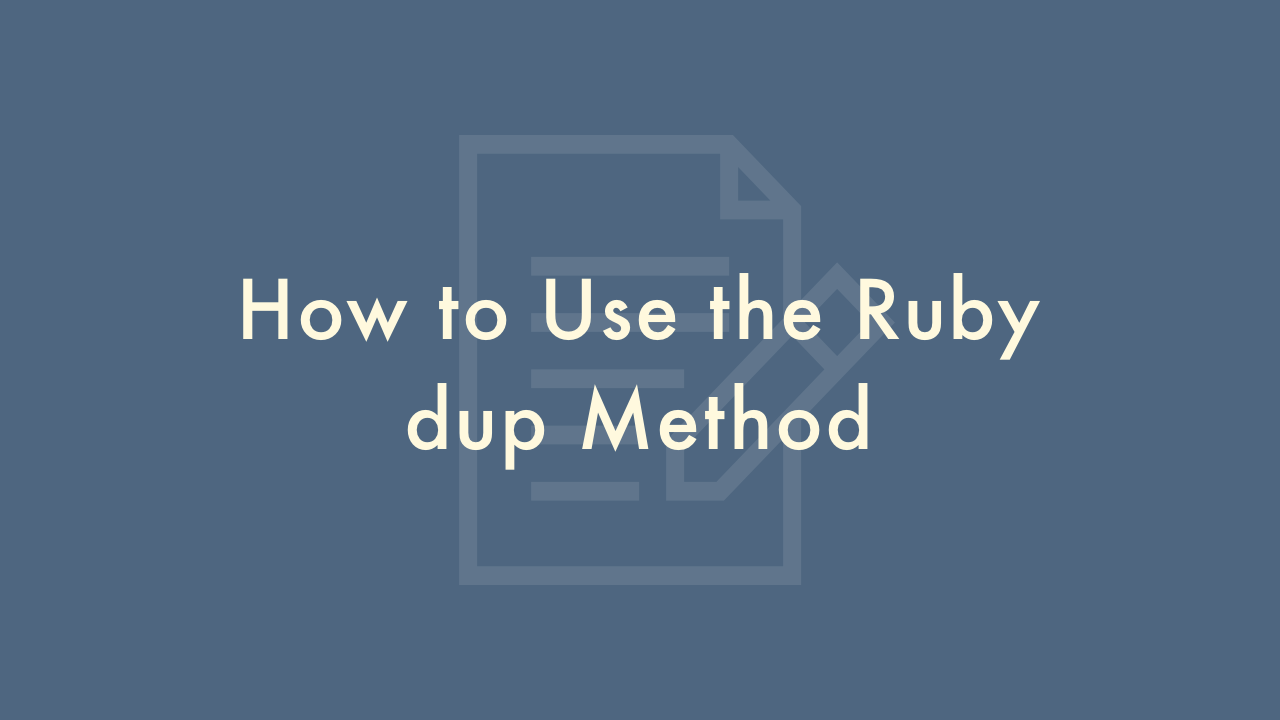
Contents
In this article, you will learn how to use the Ruby dup method.
Using the dup method
The dup method is a Ruby built-in method that creates a shallow copy of an object. It is a method that can be used on any Ruby object to create a duplicate of that object. The dup method can be used with a variety of data types, including arrays, hashes, strings, and custom objects.
Here are some common use cases for the dup method:
Copying an array or hash
The dup method can be used to create a shallow copy of an array or hash. This is useful if you want to make changes to a copy of an array or hash without modifying the original.
original_array = [1, 2, 3, 4]
new_array = original_array.dup
new_array << 5
puts original_array.inspect # prints [1, 2, 3, 4]
puts new_array.inspect # prints [1, 2, 3, 4, 5]
In this example, new_array is a duplicate of original_array, and the << operator is used to append the value 5 to new_array. The inspect method is used to print the contents of the arrays before and after the modification.
Copying a string
The dup method can also be used to create a copy of a string. This is useful if you want to modify a string without changing the original.
original_string = "hello"
new_string = original_string.dup
new_string.upcase!
puts original_string # prints "hello"
puts new_string # prints "HELLO"
In this example, new_string is a duplicate of original_string, and the upcase! method is used to convert the string to uppercase. Because upcase! modifies the string in place, original_string remains unchanged.
Creating a copy of a custom object
The dup method can also be used to create a copy of a custom object. This is useful if you have an object that you want to modify without changing the original.
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
end
person1 = Person.new("Alice", 30)
person2 = person1.dup
person2.name = "Bob"
puts person1.name # prints "Alice"
puts person2.name # prints "Bob"
In this example, person2 is a duplicate of person1, and the name attribute is changed to "Bob". Because person2 is a separate object from person1, changing the name attribute of person2 does not affect person1.