How to Get Current Date and Time in JavaScript
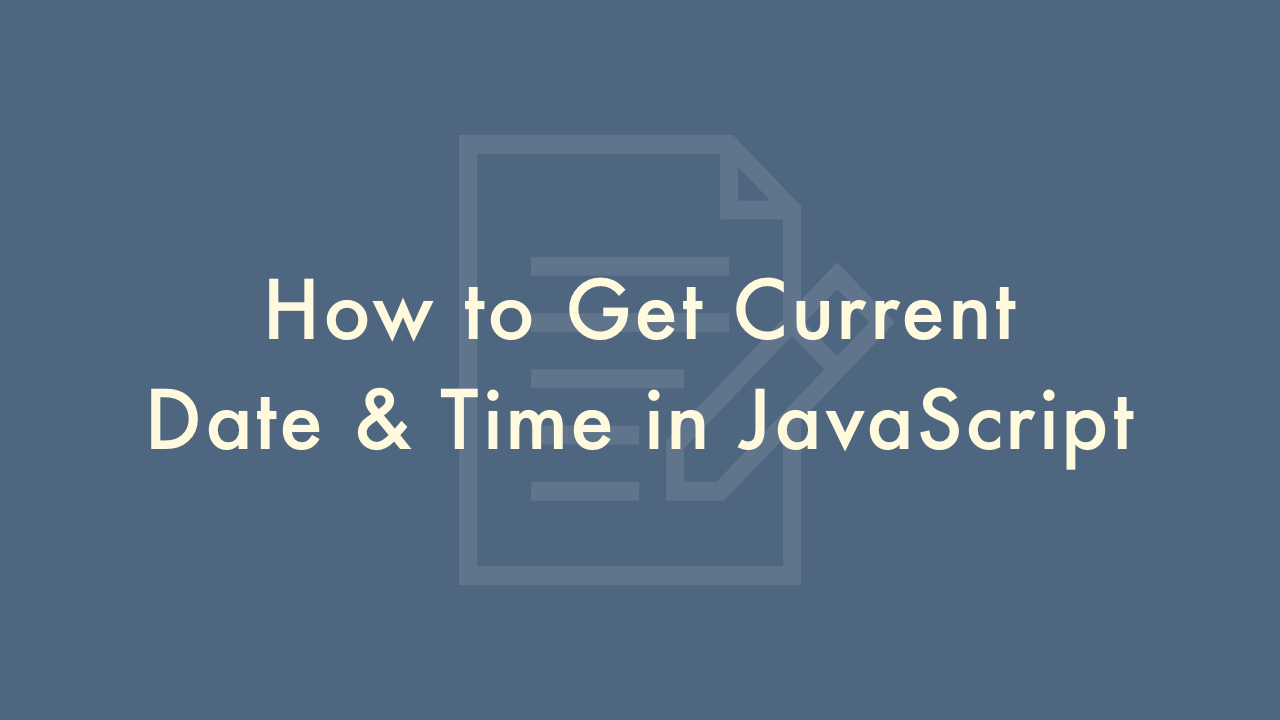
Contents
In this article, you will learn how to get current date and time in JavaScript.
JavaScript Date Objects
The Date object is one of the standard built-in objects used when dealing with dates.
If you use this object, use new
to create it like this:
const d = new Date();
After creating a new date object, you can use various methods to get the current date and time.
getFullYear()
You can get the current year with the getFullYear() method.
The getFullYear() method returns a four-digit number, for example, 1990.
Below is a sample to get the current year.
const today = new Date();
const year = today.getFullYear();
getMonth()
You can get the current month with the getMonth() method.
The getMonth() method returns an integer value between 0 and 11.
January is 0, February is 1, and so on.
Therefore, you need to add 1 as shown in the sample below.
const today = new Date();
const month = today.getMonth() + 1;
getDate()
You can get the current day of the month with the getDate() method.
The getDate() method returns an integer value between 1 and 31.
Below is a sample to get the current day of the month.
const today = new Date();
const day = today.getDate();
getDay()
You can get the current day of the week with the getDay() method.
The getDay() method returns an integer value between 0 and 6.
0 is Sunday, 1 is Monday, 2 is Tuesday, and so on.
Below is a sample to get the current day of the week.
const today = new Date();
const weekday = today.getDay();
getHours()
You can get the hour of the current time with the getHours() method.
The getHours() method returns an integer value between 0 and 23.
Below is a sample to get the current hour.
const today = new Date();
const hours = today.getHours();
getMinutes()
You can get the minutes of the current time with the getMinutes() method.
The getMinutes() method returns an integer value between 0 and 59.
Below is a sample to get the minutes of the current time.
const today = new Date();
const minutes = today.getMinutes();
getSeconds()
You can get the seconds of the current time with the getSeconds() method.
The getSeconds() method returns an integer value between 0 and 59.
Below is a sample to get the seconds of the current time.
const today = new Date();
const seconds = today.getSeconds();
getMilliseconds()
You can get the milliseconds of the current time with the getMilliseconds() method.
The getMilliseconds() method returns an integer value between 0 and 999.
Below is a sample to get the milliseconds of the current time.
const today = new Date();
const getMilliseconds = today.getMilliseconds();