Get a User’s Location with JavaScript
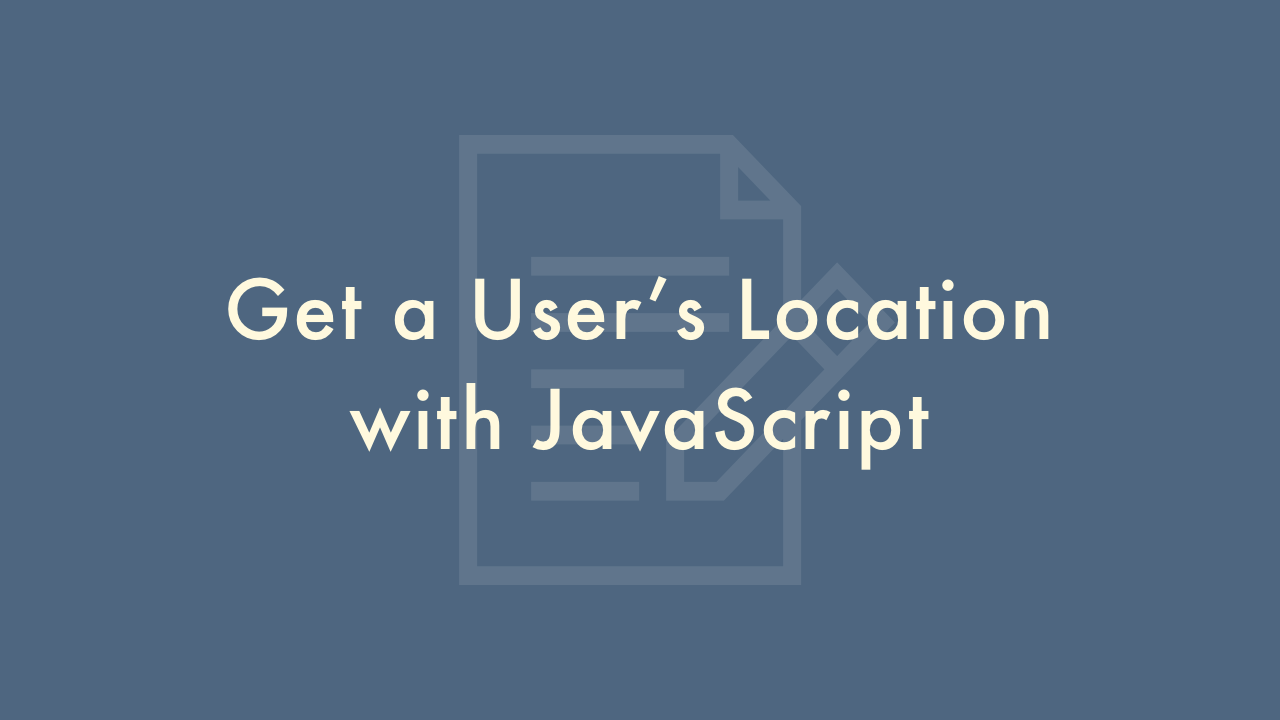
10/10/2021
Contents
In this article, you will learn how to get a user’s location with JavaScript.
Geolocation API
To get the location information of a user, you can use the Geolocation API.
For privacy reasons, user approval is required to get the location information.
Demo
You can get your location information by clicking the button.
Code
HTML
<button type="button" id="btn">Show Location</button>
<div id="result"></div>
JavaScript
function getLocation() {
if (navigator.geolocation) {
const options = {
enableHighAccuracy: true,
maximumAge: 30000,
timeout: 27000
};
navigator.geolocation.getCurrentPosition(showLocation, showError, options);
} else {
document.getElementById('result').innerHTML = 'Your browser does not support Geolocation.';
}
}
function showLocation(position) {
const data = position.coords;
const lat = data.latitude;
const lng = data.longitude;
const alt = data.altitude;
const acc = data.accuracy;
const altAcc = data.altitudeAccuracy;
const heading = data.heading;
const speed = data.speed;
document.getElementById('result').innerHTML = '<dl><dt>latitude</dt><dd>' + lat + '</dd><dt>longitude</dt><dd>' + lng + '</dd><dt>altitude</dt><dd>' + alt + '</dd><dt>accuracy</dt><dd>' + acc + '</dd><dt>altitudeAccuracy</dt><dd>' + altAcc + '</dd><dt>heading</dt><dd>' + heading + '</dd><dt>speed</dt><dd>' + speed + '</dd></dl>';
}
function showError(error) {
document.getElementById('result').innerHTML = error.message;
}
document.getElementById('btn').addEventListener('click', () => {
getLocation();
});