How to Count the Occurrence of a Substring within a String in JavaScript
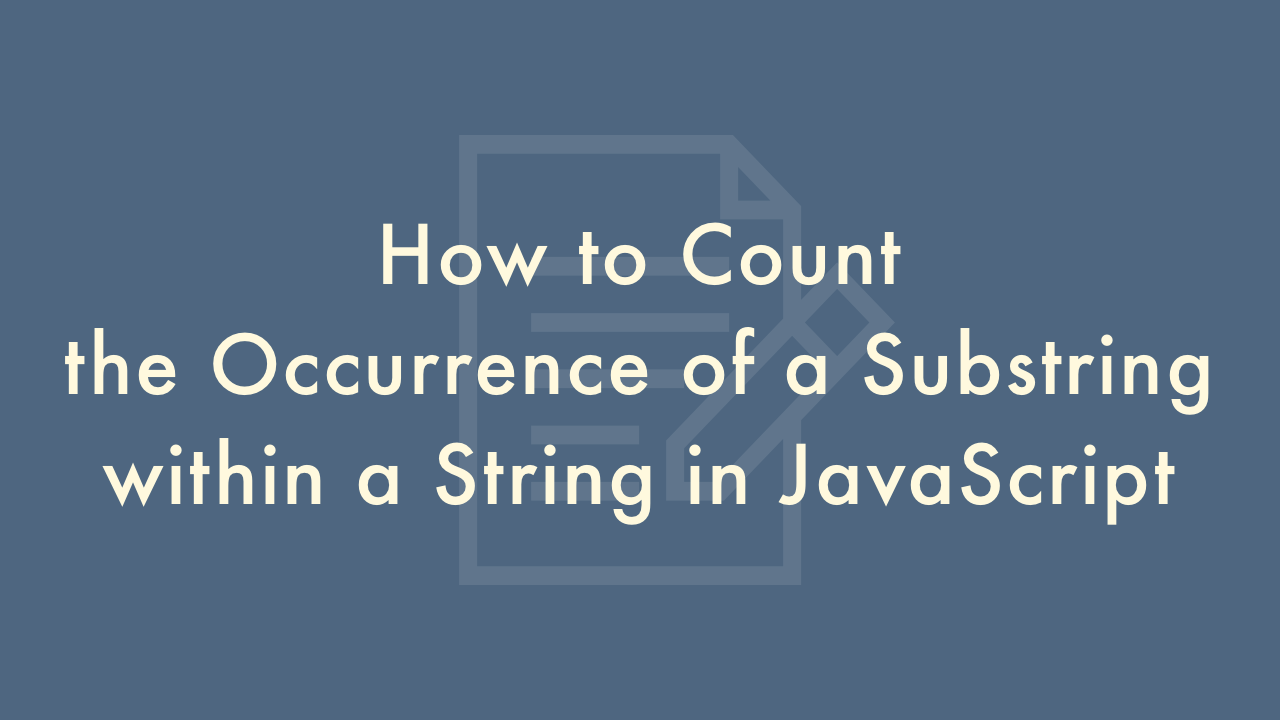
Contents
In this article, you will learn how to count the occurrence of a substring in a string in JavaScript.
Counting the occurrence of a substring within a string
Here’s a guide on how to count the occurrence of a substring in a string in JavaScript:
Using the String.prototype.match() method
The match() method returns an array of all matches found in a string based on a regular expression. We can use a regular expression to match the substring and then count the number of matches found in the array.
const string = 'I love JavaScript and JavaScript is my favorite programming language';
const substring = 'JavaScript';
const matches = string.match(new RegExp(substring, 'g'));
const count = matches ? matches.length : 0;
console.log(count); // Output: 2
Using the String.prototype.split() method
The split() method splits a string into an array of substrings based on a specified separator. We can split the string using the substring as the separator and then count the length of the resulting array minus one.
const string = 'I love JavaScript and JavaScript is my favorite programming language';
const substring = 'JavaScript';
const count = string.split(substring).length - 1;
console.log(count); // Output: 2
Using a while loop
We can use a while loop to iterate over the string and count the occurrence of the substring using the indexOf() method.
const string = 'I love JavaScript and JavaScript is my favorite programming language';
const substring = 'JavaScript';
let count = 0;
let index = string.indexOf(substring);
while (index !== -1) {
count++;
index = string.indexOf(substring, index + 1);
}
console.log(count); // Output: 2
Using the ES6 spread operator and the String.prototype.matchAll() method
The matchAll() method returns an iterator of all matches found in a string based on a regular expression. We can use the spread operator to convert the iterator to an array and then count the length of the array.
const string = 'I love JavaScript and JavaScript is my favorite programming language';
const substring = 'JavaScript';
const matches = [...string.matchAll(new RegExp(substring, 'g'))];
const count = matches.length;
console.log(count); // Output: 2