Separate Numbers with Commas in JavaScript
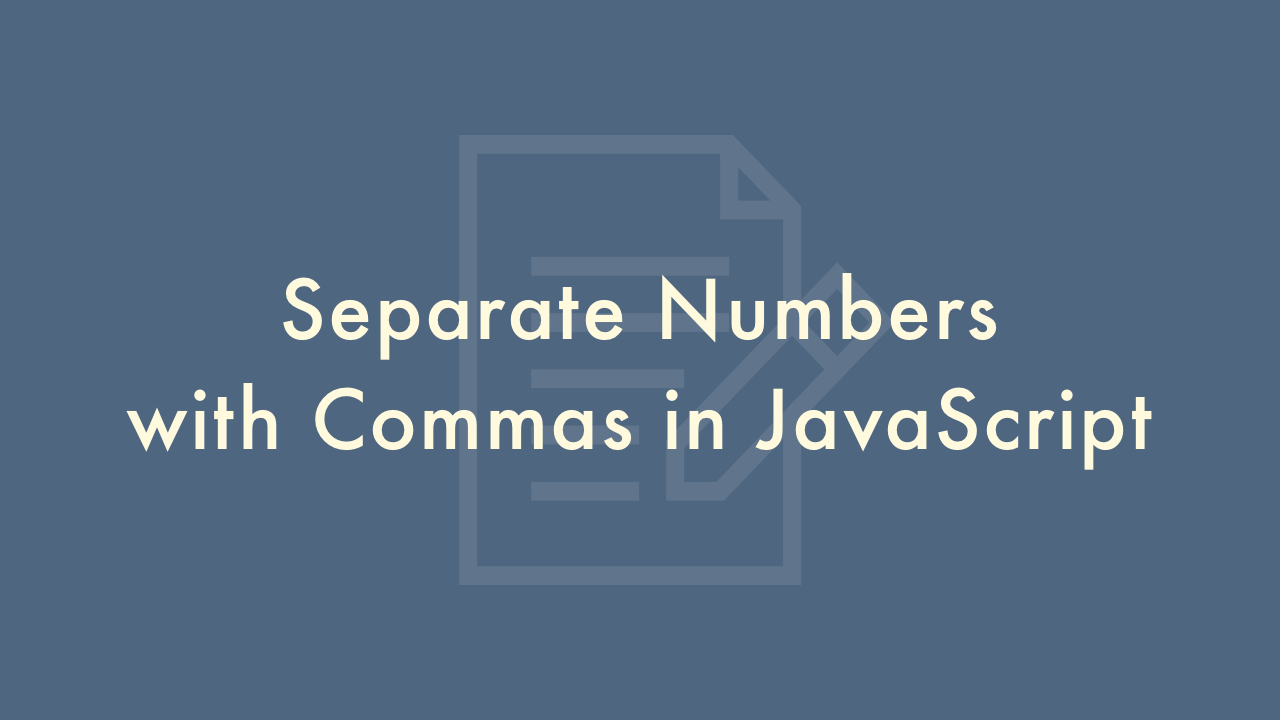
02/09/2022
Contents
In this article, you will learn how to separate numbers with commas in JavaScript.
The toLocaleString() method
The easiest way to get a comma separated string is to use the toLocaleString method.
The syntax is below.
toLocaleString()
toLocaleString(locales)
toLocaleString(locales, options)
The following is the basic usage without specifying the locale.
const num = 123456789;
console.log(num.toLocaleString()); // 123,456,789
If you specify locales, you can get the string corresponding to the output format of each country.
const number = 123456.789;
// German
console.log(number.toLocaleString('de-DE')); // 123.456,789
// Arabic
console.log(number.toLocaleString('ar-EG')); // ١٢٣٤٥٦٫٧٨٩
// India
console.log(number.toLocaleString('en-IN')); // 1,23,456.789
By specifying the argument option, you can add a unit of money or specify the number of decimal places according to the output format of each country.
const num = 12345.6789
console.log(num.toLocaleString('ja-JP', { style: 'currency', currency: 'JPY',minimumFractionDigits: 2, maximumFractionDigits: 2 }))
// ¥12,345.68
The replace() method
You can get a comma-separated string by using the replace method and a regular expression as shown below.
"12345".replace(/(d)(?=(ddd)+(?!d))/g, '$1,');
// "12,345"