How to Use Strict Mode in JavaScript
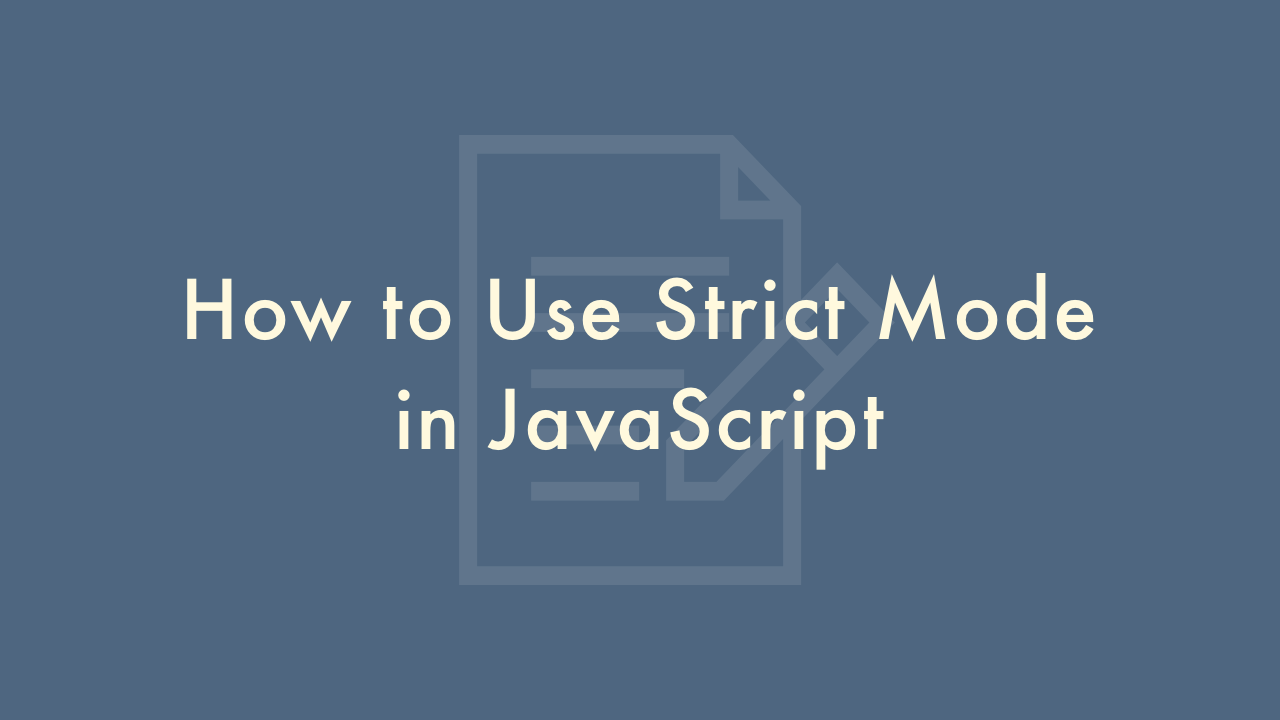
09/30/2021
Contents
In this article, you will learn how to use strict mode in JavaScript.
Using strict mode in JavaScript
Strict mode is a feature in JavaScript that was introduced in ECMAScript 5 (ES5). It is a way to opt into a more restricted mode of JavaScript, where certain types of errors that would have gone unnoticed in the past are caught and thrown as errors. This helps developers write more reliable and predictable code.
To use strict mode in JavaScript, you need to add the following line of code at the beginning of your script or function:
"use strict";
Here are some ways in which strict mode can help you write better JavaScript code:
- Prevents the use of undeclared variables: In non-strict mode, if you use a variable without declaring it first, JavaScript will create a global variable with that name. This can lead to unexpected behavior and bugs. In strict mode, using an undeclared variable will result in a ReferenceError.
- Makes eval() safer: In non-strict mode, using the eval() function to execute code can be dangerous, as it can execute any code passed to it, including potentially malicious code. In strict mode, using eval() creates a new variable scope, preventing it from accessing variables from the calling scope.
- Disallows duplicate parameter names: In non-strict mode, if you define a function with duplicate parameter names, the last one will override the previous ones. In strict mode, defining a function with duplicate parameter names will result in a SyntaxError.
- Prevents the use of reserved keywords as variable names: In non-strict mode, you can use reserved keywords as variable names without any issues. In strict mode, using a reserved keyword as a variable name will result in a SyntaxError.
- Makes debugging easier: In non-strict mode, certain types of errors can go unnoticed, leading to bugs that are difficult to debug. In strict mode, these errors are caught and thrown as errors, making it easier to find and fix them.