Check If Variable is Undefined in JavaScript
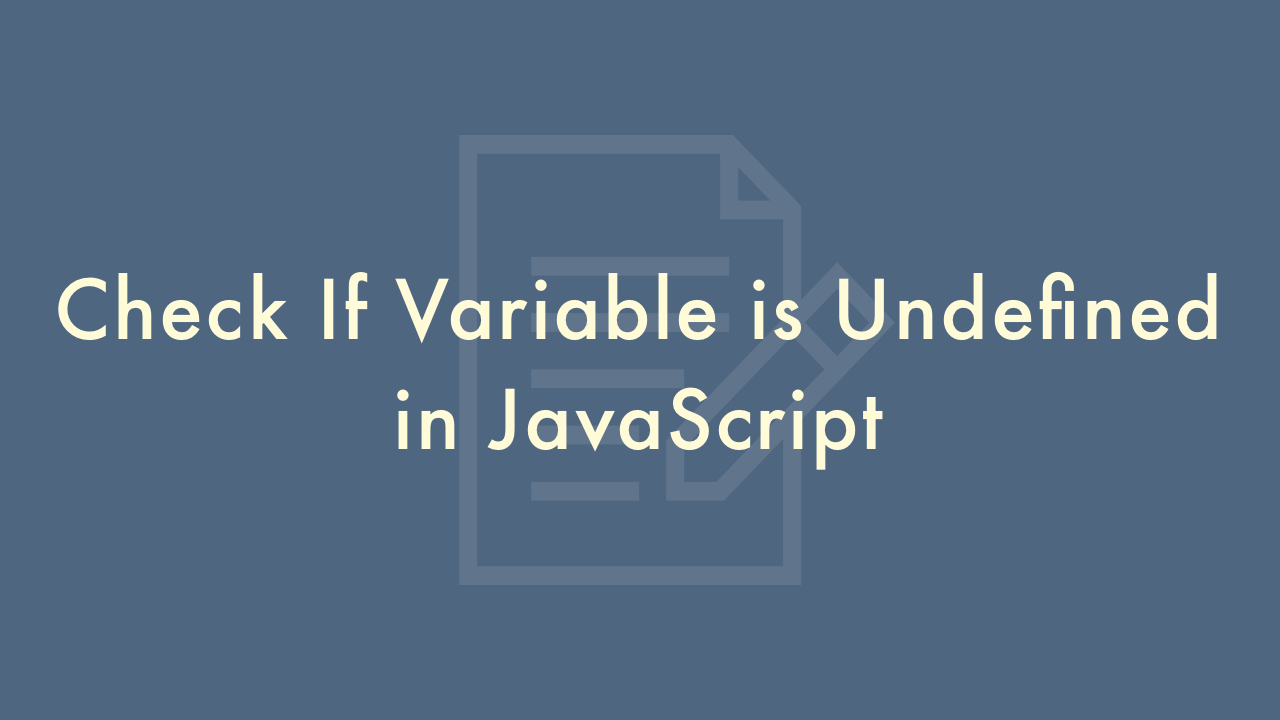
Contents
In this article, you will learn how to check if variable is undefined in JavaScript.
What is “undefined” in JavaScript?
If a variable that does not exist is referenced, as in the script below, “undefined” is returned.
const test;
console.log(test);
console.log(window.test);
Strictly speaking, “undefined” is returned as a return value when an uninitialized variable is referenced.
It is also returned as a return value if the object does not exist.
Even if the process is normal, if there is no return value, the return statement will be executed and “undefined” will be output.
Check if variable is undefined
Below is the sample code to check if variable is undefined.
The undefined judgment is made by the typeof operator using an if
statement.
let _undefined;
if (typeof _undefined == "undefined") {
console.log("Variable is Undefined")
}
This judgment may be used to detect when an abnormality occurs in the process and to control a partial outage to avoid a full outage of the system.
However, it is not the process that should be done, so if this process is executed, the program source code to realize the function should also be improved.