How to Use Local Storage with JavaScript
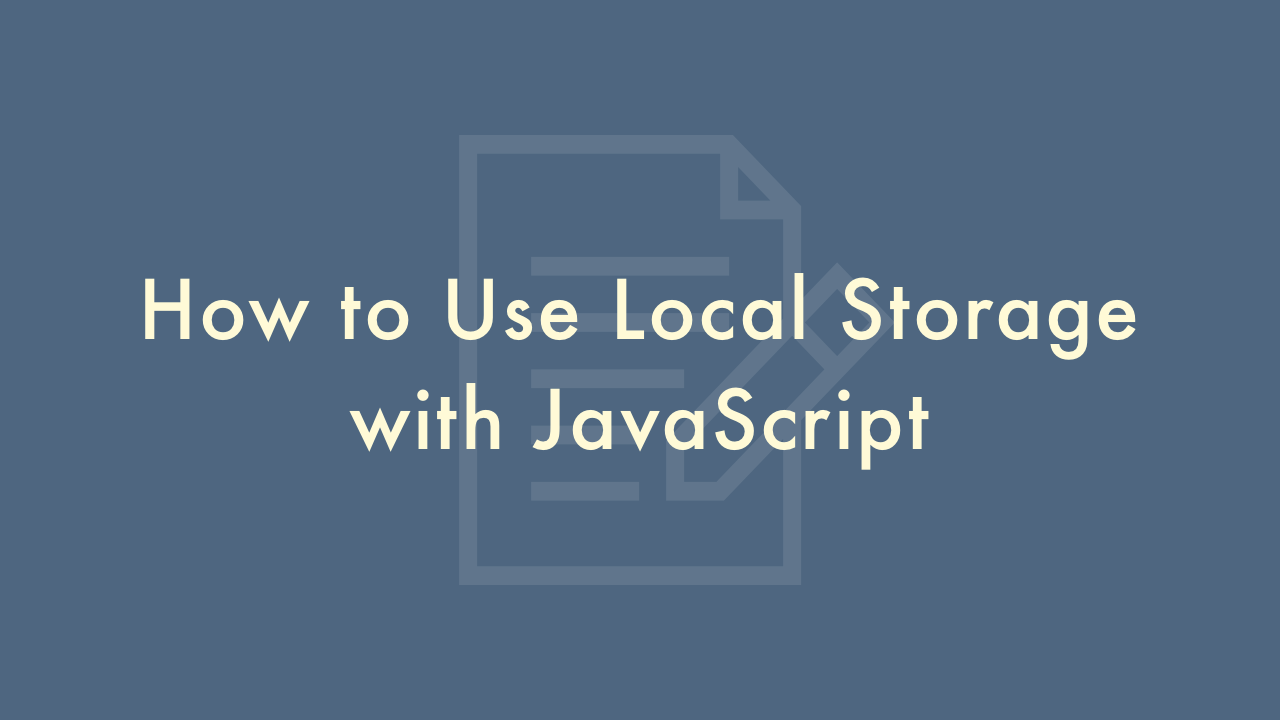
Contents
In this article, you will learn how to use local storage with JavaScript.
What is localStorage?
The localStorage is the area where data is stored in the web browser.
The feature is that it remains saved even when the browser is closed.
You can use this localStorage to store and retrieve data.
The localStorage is a Storage object and is referenced as a property of the Window object.
Let’s check by outputting the localStorage to the console.
console.log(window.localStorage)
// or
console.log(localStorage)
If you check the localStorage object in console, you can see that it has only the length property.
This represents the number of data stored in the localStorage.
If nothing is saved, the value will be 0.
Save data to localStorage
Use the setItem() method to store the data in localStorage.
Pass the name of the key in the first argument and the value in the second argument.
localStorage.setItem('key', 'value')
Let’s execute this method and output the status of localStorage to the console to check it.
Since you saved one data, the value of the length property should be incremented by one.
Get data from localStorage
To get the data from the localStorage, use the getItem() method.
By specifying the name of the key in the argument, the value corresponding to the key is returned as the return value.
const value = localStorage.getItem('key')
Remove data from localStorage
To remove data from the localStorage, use the removeItem() method.
localStorage.removeItem('key')
If you execute this method and check the status of localStorage, you’ve remove one piece of data, so the value of the length property should be decremented by one.