The Execution Order in JavaScript
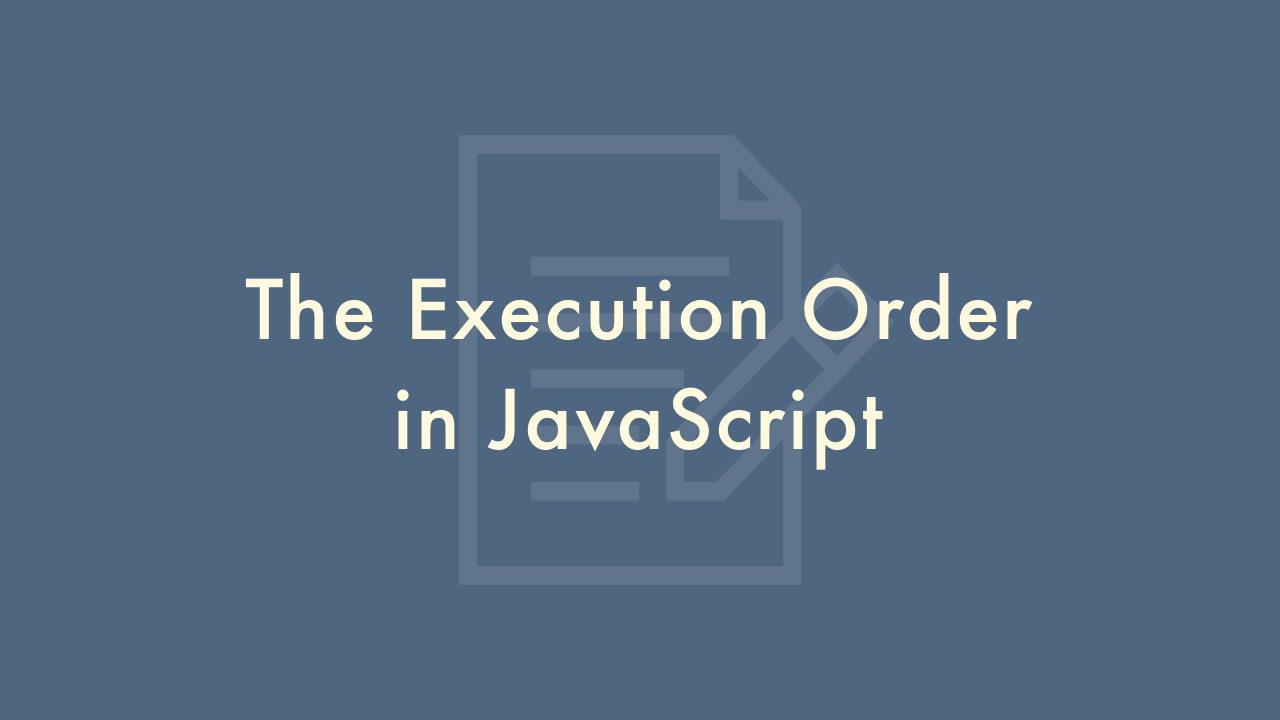
Contents
In this article, you will learn the execution order in JavaScript.
When JavaScript is executed
Web pages consist of HTML, CSS, and JavaScript.
JavaScript can be written directly in the script tag or refer to an external file.
Web pages are basically loaded with HTML in order from the top, and the CSS and JavaScript written there are interpreted.
Therefore, note that the operation may change depending on the description location.
The execution order in JavaScript
As an example, if you have the following HTML and JavaScript:
HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>The Execution Order in JavaScript</title>
<script type="text/javascript" src="main.js"></script>
</head>
<body>
<input type="text" value="name" id="name">
<input type="button" value="submit" id="button">
</body>
</html>
JavaScript – main.js
document.getElementById("button").onclick = () => {
name = document.getElementById("name").value;
console.log(`${name}`);
};
Executing the above will result in an error.
This is because the reference of the JavaScript file is written in the head tag, so the JavaScript is interpreted before the element in the body tag is loaded.
At the time of interpreting JavaScript, the HTML elements don’t exist, so an error will occur.
To avoid errors, put a JavaScript file reference at the bottom of the body tag.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>The Execution Order in JavaScript</title>
</head>
<body>
<input type="text" value="name" id="name">
<input type="button" value="submit" id="button">
<script type="text/javascript" src="main.js"></script>
</body>
</html>
Be careful when loading multiple JavaScript files.
Specifically, load the library such as jQuery first.
It’s a good idea to load the functions and libraries that are supposed to exist first.