How to Use the Math.log() Method in JavaScript
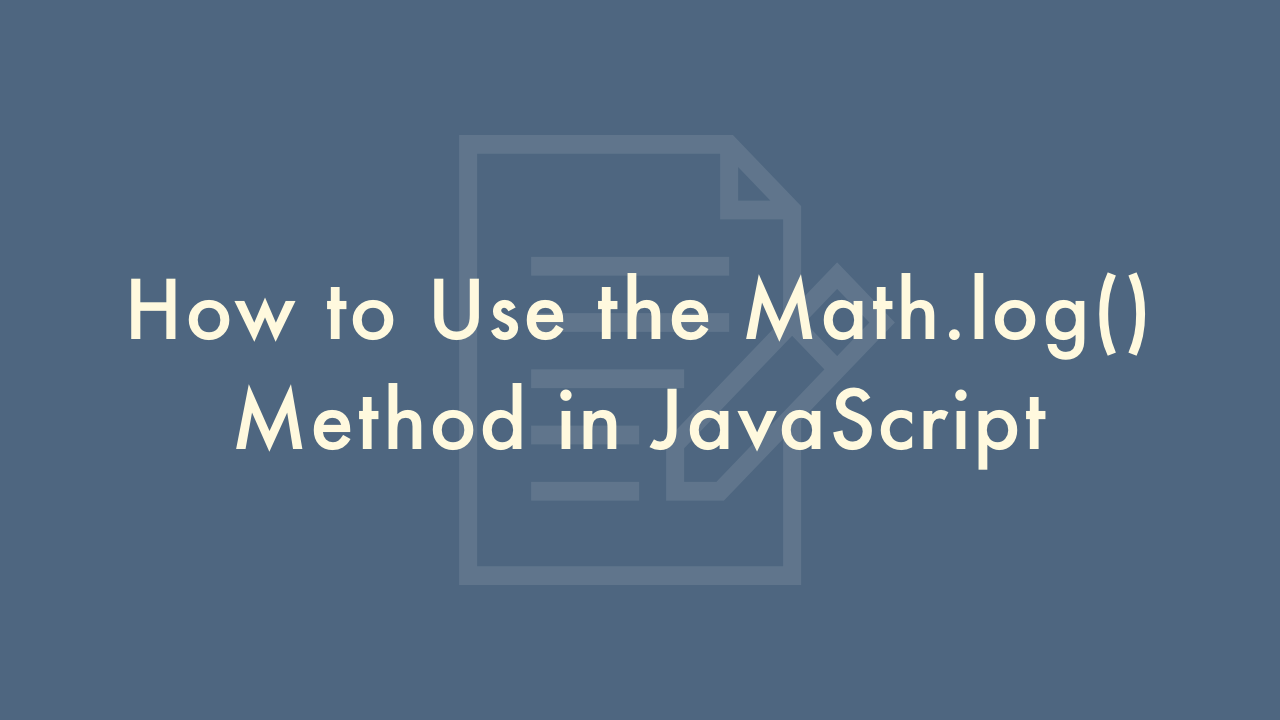
Contents
In this article, you will learn how to use the Math.log() method in JavaScript.
Using the Math.log() method in JavaScript
The Math.log() method is a built-in function in JavaScript that returns the natural logarithm (base e) of a given number. The input parameter must be a positive number.
Syntax
The syntax for using the Math.log() method is:
Math.log(x)
where x is the input parameter that represents the number to calculate the natural logarithm of.
Examples
Using Math.log() to calculate the natural logarithm of a number
const x = 5;
const naturalLogarithm = Math.log(x);
console.log(naturalLogarithm); // 1.6094379124341003
In this example, the input parameter is 5. The output is the natural logarithm (base e) of 5, which is approximately 1.6094.
Using Math.log() with a value less than or equal to 0
const x = -2;
const naturalLogarithm = Math.log(x);
console.log(naturalLogarithm); // NaN
In this example, the input parameter is -2, which is less than or equal to 0. Therefore, the output is NaN.
Using Math.log() with a value greater than 0
const x = 0.2;
const naturalLogarithm = Math.log(x);
console.log(naturalLogarithm); // -1.6094379124341003
In this example, the input parameter is 0.2. The output is the natural logarithm (base e) of 0.2, which is approximately -1.6094.
Note: If you want to calculate the logarithm of a number using a different base, you can use the following formula:
logb(x) = Math.log(x) / Math.log(b)
where x is the number to calculate the logarithm of, and b is the desired base.