Get the Touch Position with JavaScript
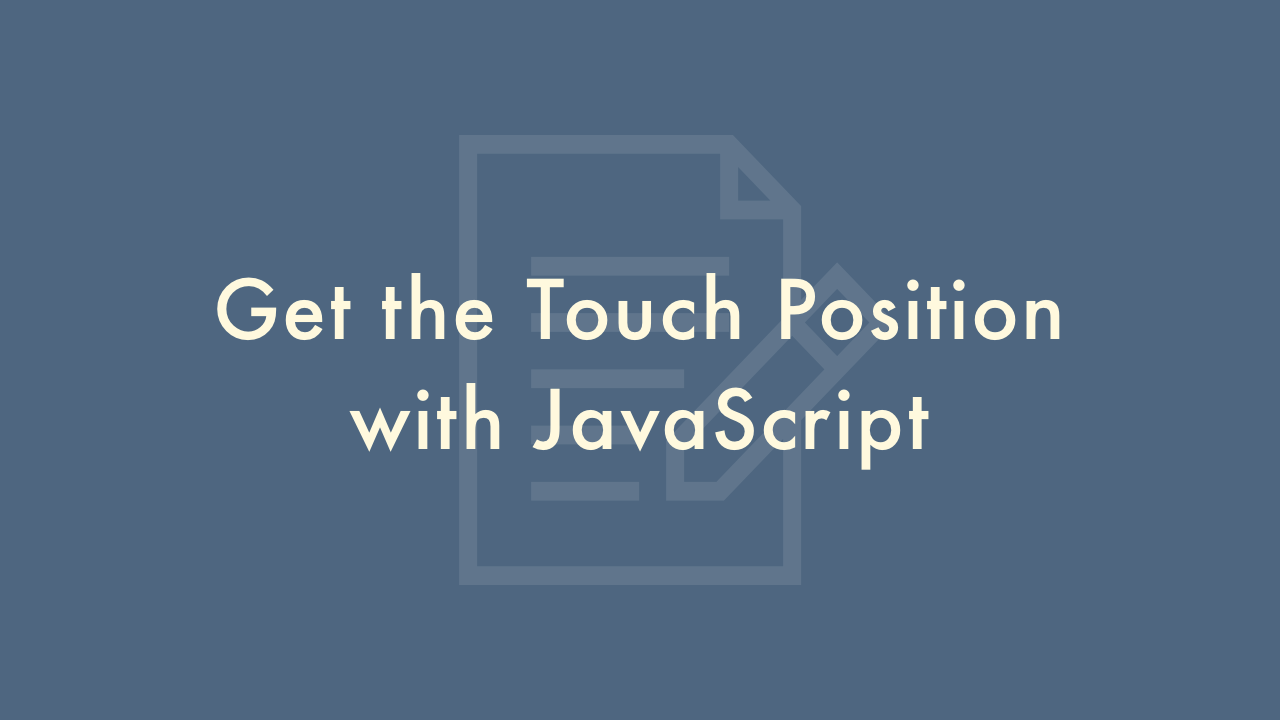
10/17/2021
Contents
In this article, you will learn how to get the touch position with JavaScript.
Touch.clientX/clientY
To get the touch position, you can use the JavaScript Touch.clientX and Touch.clientY property.
Demo
You can get the touch position by touching the screen within the demo range (the origin is at the upper left of the demo range).
Code
HTML
<p class="position">
X: <span id="x"></span><br>
Y: <span id="y"></span>
</p>
JavaScript
let touchHandler = function(event) {
let x = 0, y = 0;
if (event.touches && event.touches[0]) {
x = event.touches[0].clientX;
y = event.touches[0].clientY;
} else if (event.originalEvent && event.originalEvent.changedTouches[0]) {
x = event.originalEvent.changedTouches[0].clientX;
y = event.originalEvent.changedTouches[0].clientY;
} else if (event.clientX && event.clientY) {
x = event.clientX;
y = event.clientY;
}
document.getElementById('x').innerHTML = x;
document.getElementById('y').innerHTML = y;
}
window.addEventListener('touchstart', touchHandler, false);
window.addEventListener('touchmove', touchHandler, false);
window.addEventListener('touchend', touchHandler, false);