How to Use the Array forEach() Method in JavaScript
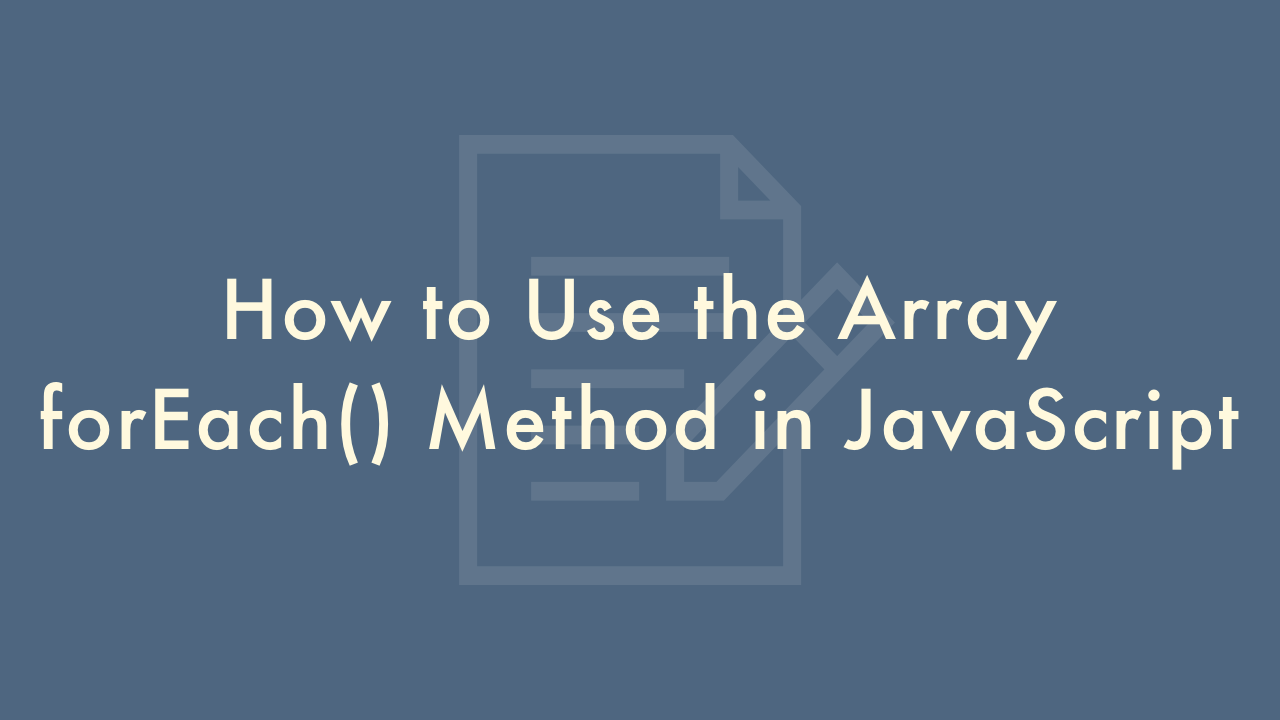
Contents
In this article, you will learn how to the array forEach() method in JavaScript.
The forEach() method
In JavaScript, there is a type called an array that can handle multiple data at once.
The forEach method is used when you want to process the data stored in this array at once by loop processing.
Normally, the for statement is used to execute a loop process in a program, but when operating the for statement on an array, the process can be implemented more easily by using the forEach method.
forEach() usage
The forEach method is a method implemented in an Array object and can be used with the following syntax.
array.forEach(callback(currentValue[, index[, array]]) {
// execute something
}[, thisArg]);
The forEach method can describe the process more easily than the for statement, but it is necessary to specify functon called a callback function in the argument part.
Below is a sample that executes loop processing using the forEach method.
var fruits = [ "apple", "banana", "lemon" ];
fruits.forEach( function( item ) {
console.log( item );
});
//result
//apple
//banana
//lemon
The forEach method executes the specified callback function individually for all the elements (items) stored in the array.
Let’s take a look at a sample when the same process as above is executed with the for statement.
var fruits = [ "apple", "banana", "lemon" ];
for (var i = 0, len = fruits.length; i < len; ++i) {
console.log(fruits[i]);
}
If you try to manipulate all the elements of an array using a for statement, you will find that it requires very esoteric programming. Since the for statement can repeatedly execute various processes other than arrays, the method of specifying variables and processes becomes complicated.
Write the forEach method in a lambda expression
By using the JavaScript lambda expression "arrow function", the forEach method can be written more intuitively and easily.
Below is a sample.
var fruits = [ "apple", "banana", "lemon" ];
fruits.forEach( item => console.log( item ) );
//When writing processing of two or more lines in the callback function
fruits.forEach( item => {
console.log( "start" );
console.log( item );
console.log( "end" );
});