Get Child Elements with JavaScript
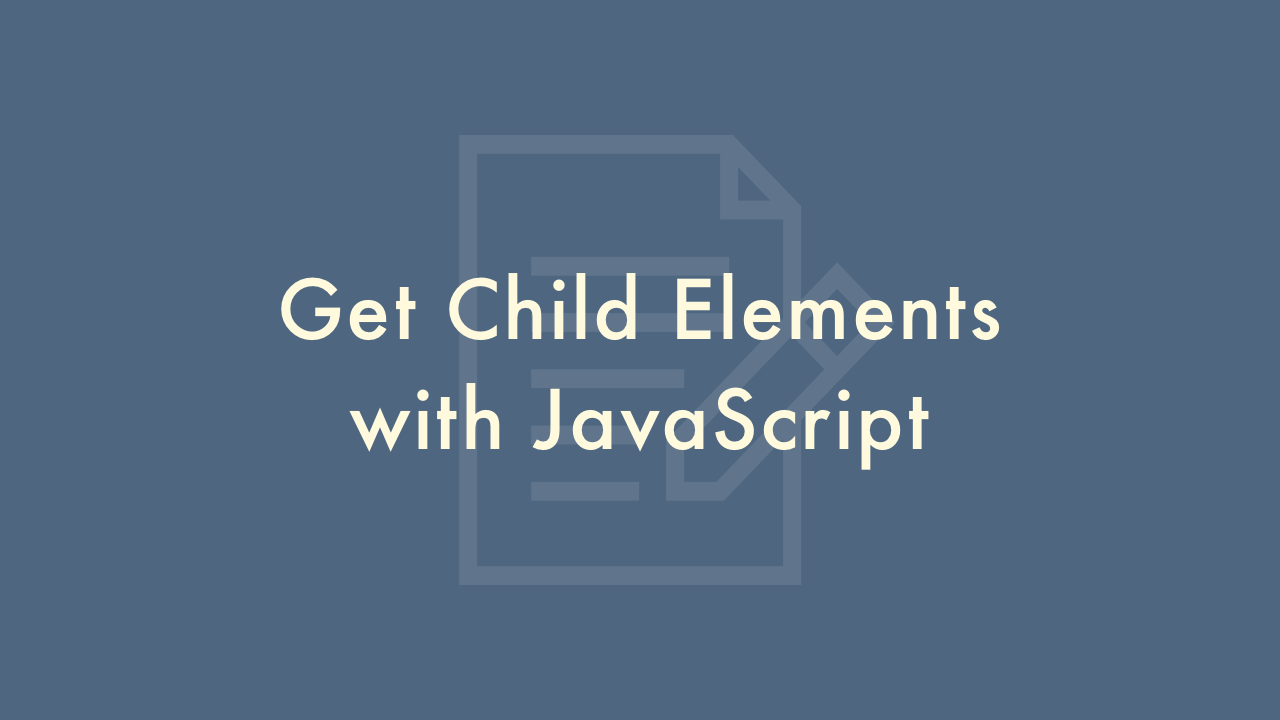
01/06/2022
Contents
In this article, you will learn how to get child elements with JavaScript.
Introduction
This article uses the list below for validation.
<ul id="parent">
<li>Item1</li>
<li>Item2</li>
<li>Item3</li>
<li>Item4</li>
<li>Item5</li>
</ul>
Get the first child element
To get the first child element, use the firstElementChild property.
JavaScript
let parent = document.getElementById("parent");
console.log(parent.firstElementChild);
//result
//<li>Item1</li>
Get the last child element
To get the last child element, use the lastElementChild property.
JavaScript
let parent = document.getElementById("parent");
console.log(parent.lastElementChild);
//result
//<li>Item5</li>
Get the nth child element
To get the nth child element, use the children of the element property.
JavaScript
let parent = document.getElementById("parent");
console.log(parent.children[1]);
//result
//<li>Item2</li>
Get odd-numbered child elements
To get the odd-numbered child elements, use the children of the element property.
JavaScript
let parent = document.getElementById("parent");
for (let i = 0; i < list.children.length; i++){
if(0 == i % 2){
console.log(list.children[i]);
}
}
//result
//<li>Item1</li>
//<li>Item3</li>
//<li>Item5</li>
Get even-numbered child elements
To get the even-numbered child elements, use the children of the element property.
JavaScript
let parent = document.getElementById("parent");
for (let i = 0; i < list.children.length; i++){
if(0 != i % 2){
console.log(list.children[i]);
}
}
//result
//<li>Item2</li>
//<li>Item4</li>
Get the child element without getting the parent element
If you don't need to get the parent element, you can get this element more easily by using querySelector or querySelectorAll.
JavaScript
//Get the first child element
var listItem = document.querySelector("#parent li:first-child");
//Get the last child element
var listItem = document.querySelector("#parent li:last-child");
//Get the nth child element
var listItem = document.querySelector("#parent li:nth-child(2)");
//Get odd-numbered child elements
var listItem = document.querySelectorAll("#parent li:nth-child(odd)");
//Get even-numbered child elements
var listItem = document.querySelectorAll("#parent li:nth-child(even)");