How to Use JavaScript Promise
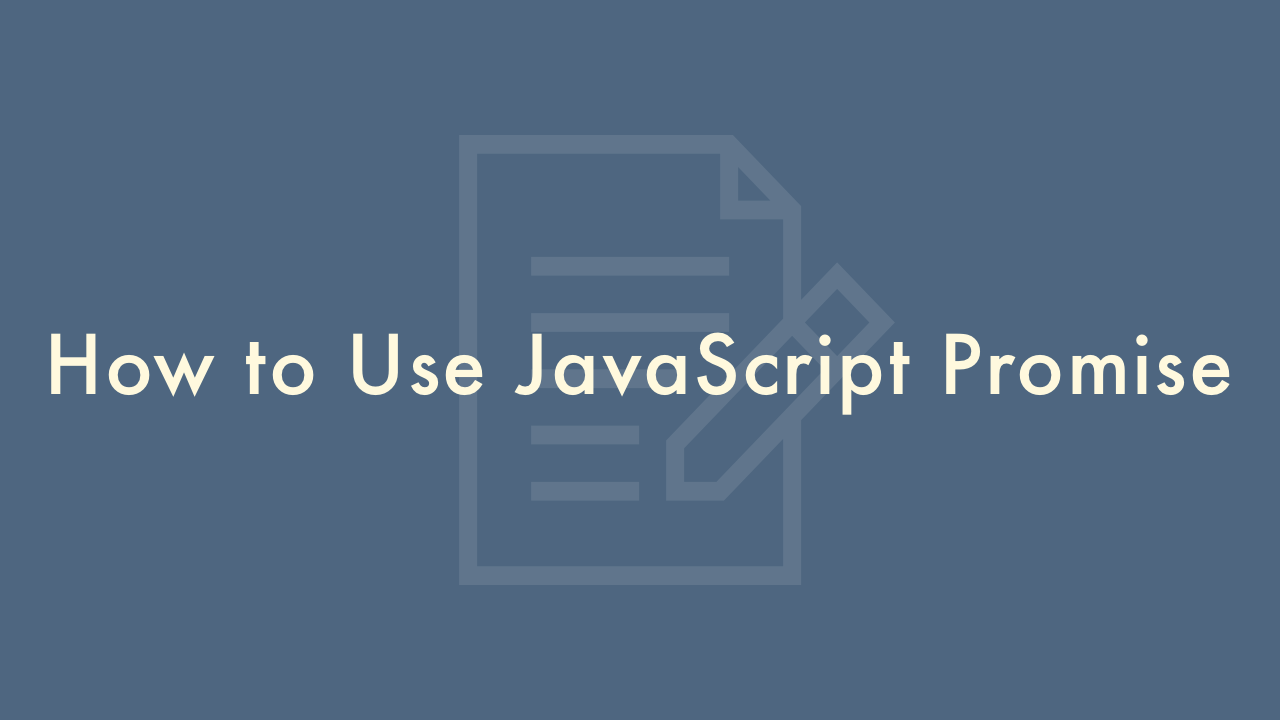
Contents
In this article, you will learn how to use JavaScript promise.
What is JavaScript Promise?
The Promise object is used to realize asynchronous processing.
This object is a value that represents the result of asynchronous processing.
Results can be divided into success (both completed and resolved) and failure (both error and rejection).
If it succeeds, it calls the function resolve that is passed as an argument, and if it fails, it calls reject that is passed as an argument.
Below is a simple sample code that implements asynchronous processing using a Promise object.
Asynchronously executes the process of starting the stopwatch timer and stopping it after n seconds.
const func = n => new Promise((resolve, reject) => {
setTimeout(() => {
resolve('SUCCESS');
}, n * 1000);
});
func(2).then(s => console.log(s)) // SUCCESS
func(3).then(s => console.log(s)) // SUCCESS
The characters SUCCESS are output 2 seconds later, and the characters SUCCESS are output again 1 second later.