How to Use the Array push() Method in JavaScript
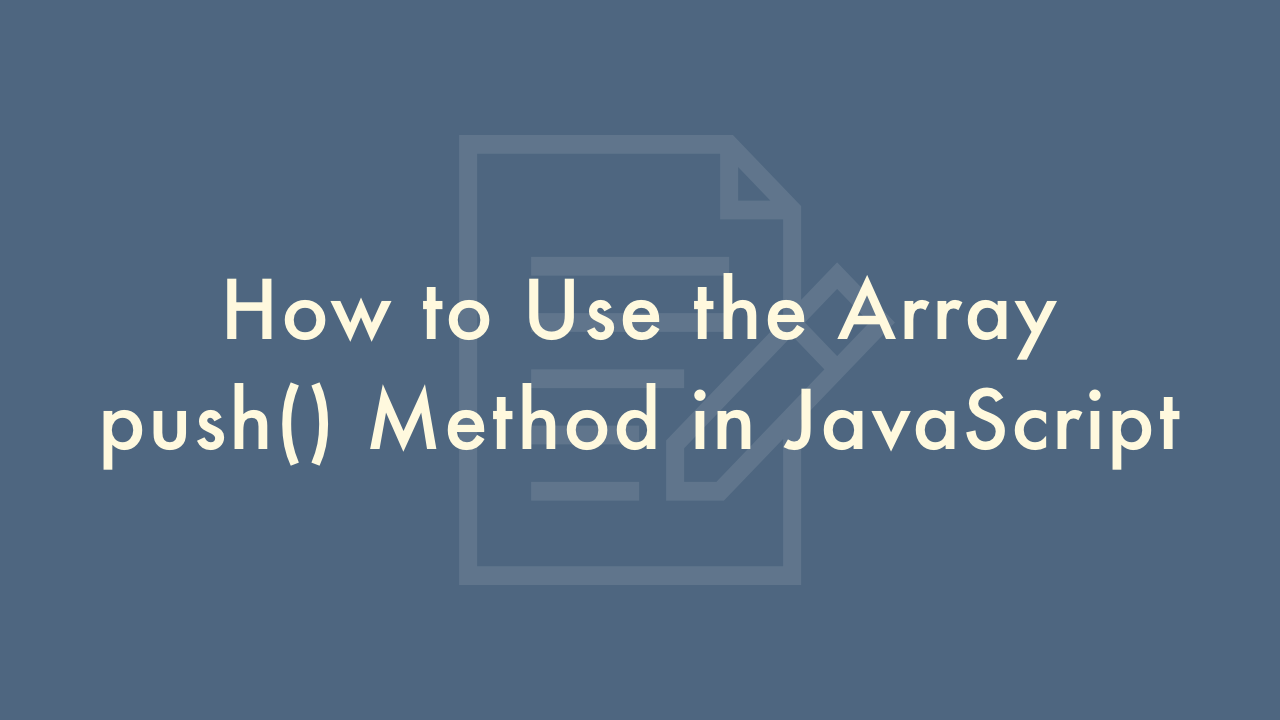
01/29/2022
Contents
In this article, you will learn how to use the array push() method in JavaScript.
The push() method
The push() method is a method for adding one or more elements to the end of an array.
For example, if you add ‘strawberry’ to the array [‘apple’,’orange’,’banana’], it will look like [‘apple’,’orange’,’banana’,’strawberry’].
The syntax is below.
array.push(element0)
array.push(element0,element1)
array.push(element0,element1,...,elementN)
Add elements to the array with push()
Below is a sample to add elements to the array.
const score_a= 80;
const score_b = 90;
const score_c = 70;
// Define an array without data
let scores = [];
// Add elements to the array
scores.push(score_a);
scores.push(score_b);
scores.push(score_c);
console.log(scores);
//[80, 90, 70]
Next, let’s see if we can add the object type to the array.
const score_a = { subject: 'a', score: 80 };
const score_b = { subject: 'b', score: 90 };
const score_c = { subject: 'c', score: 70 };
// Define an array without data
let scores = [];
// Add elements to the array
scores.push(score_a);
scores.push(score_b);
scores.push(score_c);
console.log(scores);
// [
// { subject: 'a', score: 80 },
// { subject: 'b', score: 90 },
// { subject: 'c', score: 70 }
// ]