Split an Array with JavaScript
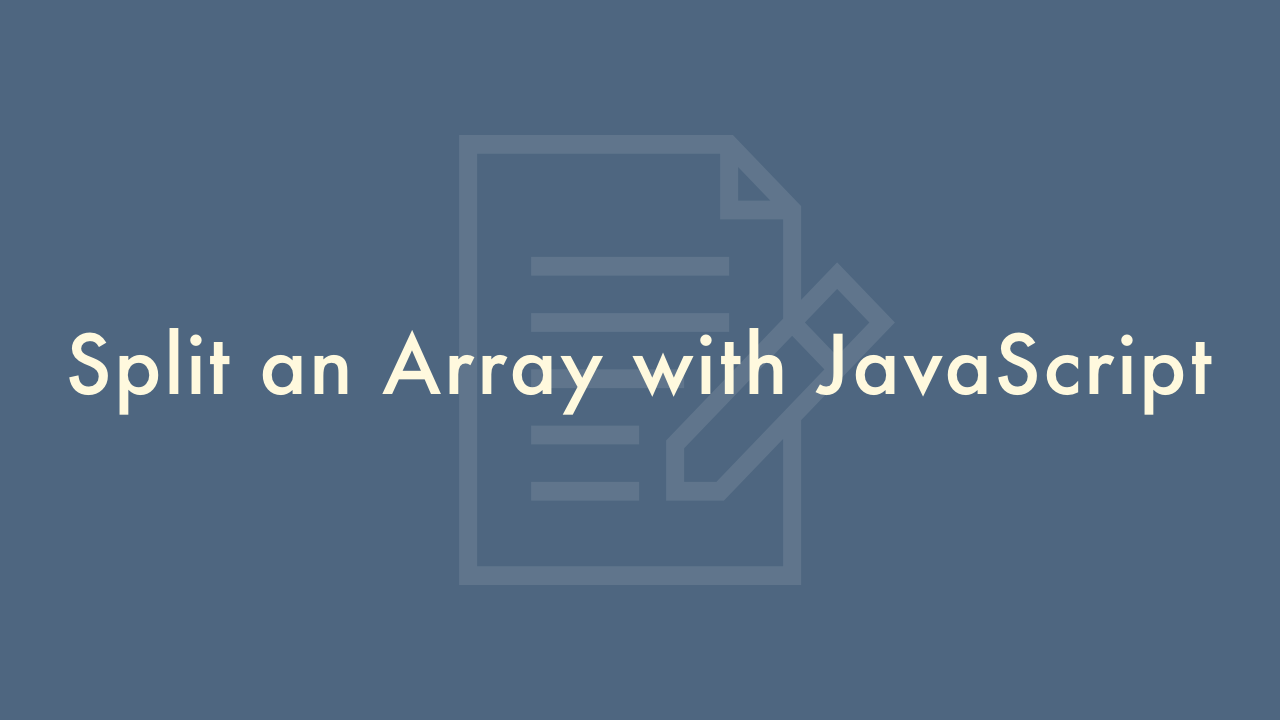
Contents
In this article, you will learn how to split an array with JavaScript.
The slice() method
The slice method is a method of a JavaScript array object that returns a new array that is a copy of the specified range of the array.
The original array remains the same because you are copying the specified range.
The syntax is below.
arr.slice([start[, end]])
There are two arguments for the slice() method.
The first argument is the index at the beginning of the specified range.
For example, if you want to get from the second of the array, the first argument is 1 (because it starts at 0, the second index is 1).
The second argument is the index at the end of the specified range, copying up to just before the index at the end.
For example, if you want to get up to the 4th of the array, the 2nd argument is 4.
Both arguments are optional, and if both are omitted, the entire array is copied from start to finish.
If only the second argument is omitted, it can be obtained from the index specified by the first argument to the end.
How to split an Array
Array splitting can be achieved by using the slice method to specify the range you want to split.
Below is sample code to split an array.
let arr1 = ['1', '2', '3', '4', '5'];
let arr2 = array.slice(1);
let arr3 = array.slice(1,4);
let arr4 = array.slice();
//result
console.log(arr2); // ['2', '3', '4', '5']
console.log(arr3); // ['2', '3', '4']
console.log(arr4); // ['1', '2', '3', '4', '5']