Get Parent Element with JavaScript
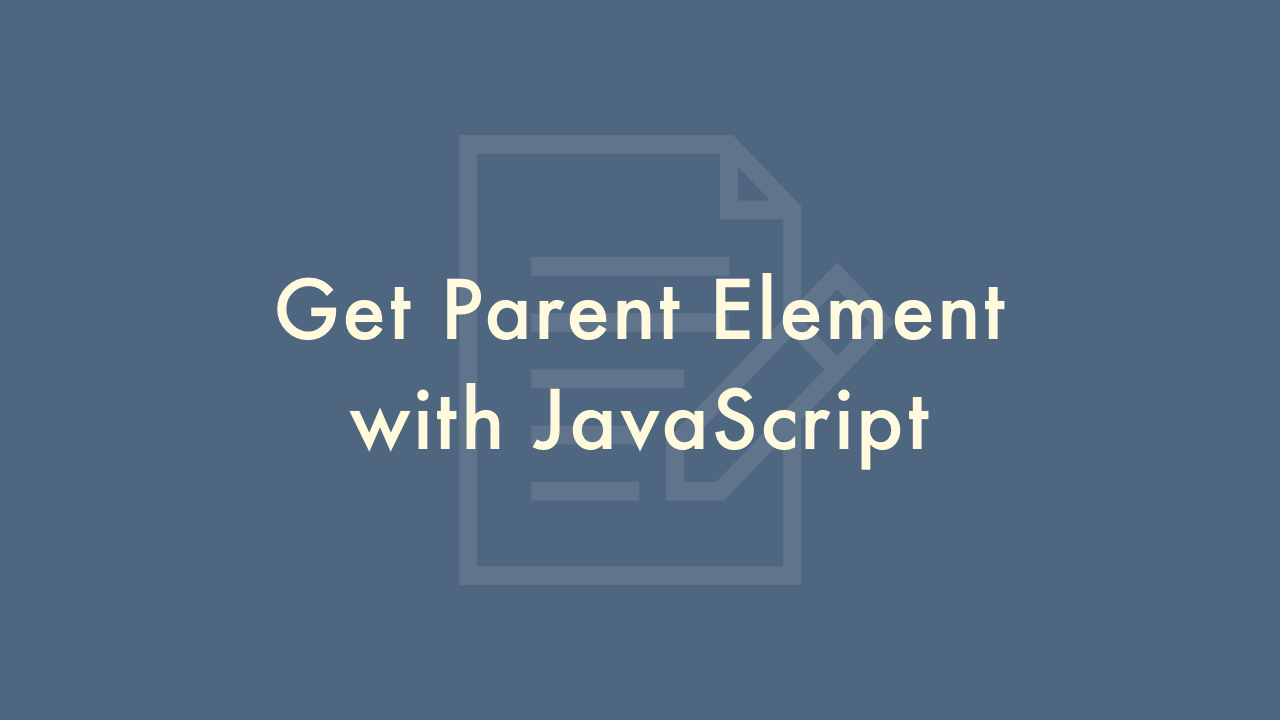
01/07/2022
Contents
In this article, you will learn how to get parent element with JavaScript.
parentElement
You can get the element that is the parent of the specified DOM node with the parentElement.
It returns null if the parent node does not exist or is not an element.
Below is a sample to get the parent element of child with parentElement.
HTML
<div id="parent">
<div id="child"></div>
</div>
JavaScript
const child = document.getElementById("child");
console.log(child.parentElement);
//result
//<div id="parent">
// <div id="child"></div>
//</div>
parentNode
You can get the parent node in the DOM tree of the node specified with parentNode.
Below is a sample to get the parent element of child with parentNode.
HTML
<div id="parent">
<div id="child"></div>
</div>
JavaScript
const child = document.getElementById("child");
console.log(child.parentNode);
//result
//<div id="parent">
// <div id="child"></div>
//</div>
The difference between parentElement and parentNode
The biggest difference between parentElement and parentNode is the result when getting the document node.
//parentElement
console.log(document.documentElement.parentElement);
//result
//null
//parentNode
console.log(document.documentElement.parentNode);
//result
//<!DOCTYPE html>
//<html>
//<head>
//</head>
//<body>
//</body>
//</html>