How to Use the String length Property in JavaScript
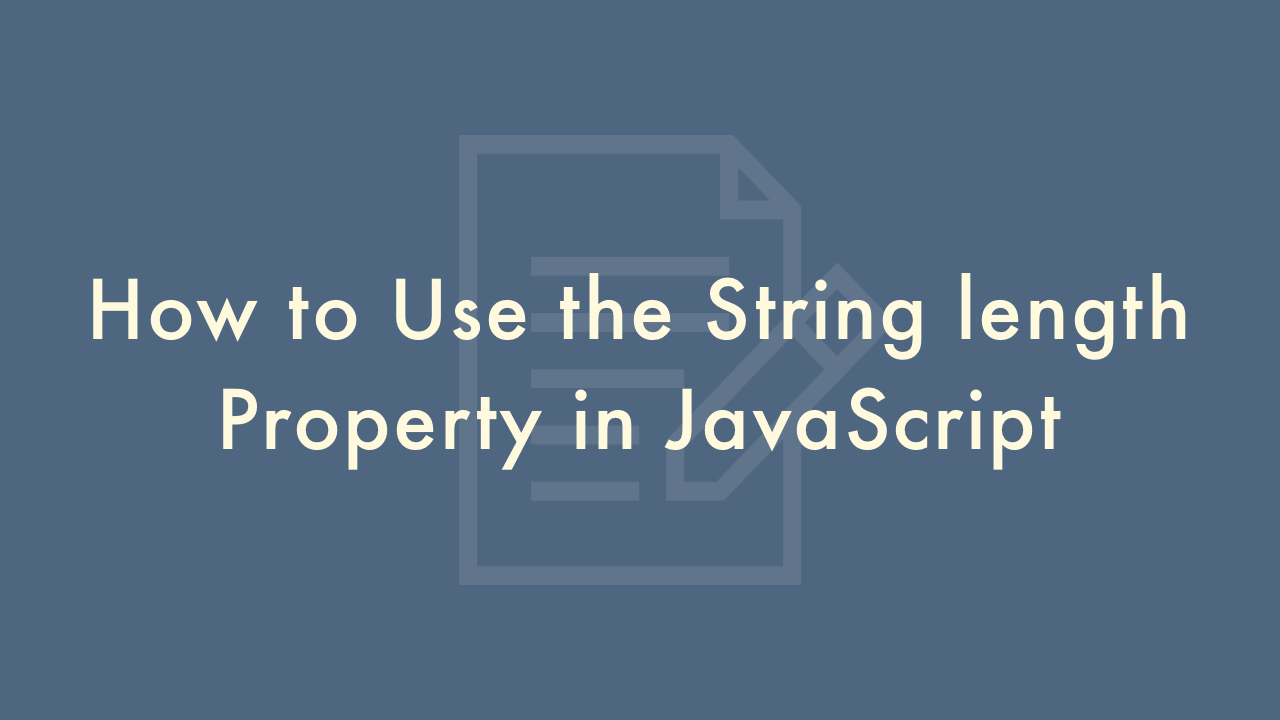
Contents
In this article, you will learn how to use the string length property in JavaScript.
Using the string length property in JavaScript
The length property is a built-in property of JavaScript strings. It returns the number of characters in a string. Here’s how to use the length property in JavaScript:
Accessing the length property
To access the length property of a string, simply append .length to the end of the string variable. For example:
let myString = "Hello, world!";
console.log(myString.length); // Output: 13
Using the length property in a conditional statement
The length property can be used in conditional statements to check if a string meets a certain length requirement. For example:
let myString = "Hello, world!";
if (myString.length > 10) {
console.log("String is long enough");
} else {
console.log("String is too short");
}
// Output: String is long enough
Concatenating strings using the length property
The length property can also be used to concatenate strings. For example:
let str1 = "Hello";
let str2 = "World";
let str3 = "!";
let combinedString = str1 + str2 + str3;
let lengthOfCombinedString = combinedString.length;
console.log(combinedString); // Output: "HelloWorld!"
console.log(lengthOfCombinedString); // Output: 11
Using the length property with the slice() method
The slice() method is used to extract a portion of a string. The length property can be used to determine the end point of the slice. For example:
let myString = "Hello, world!";
let slicedString = myString.slice(0, 5);
console.log(slicedString); // Output: "Hello"
Here, 0 is the starting index, and 5 is the end index (exclusive).