The Difference Between toFixed() and toPrecision() in JavaScript
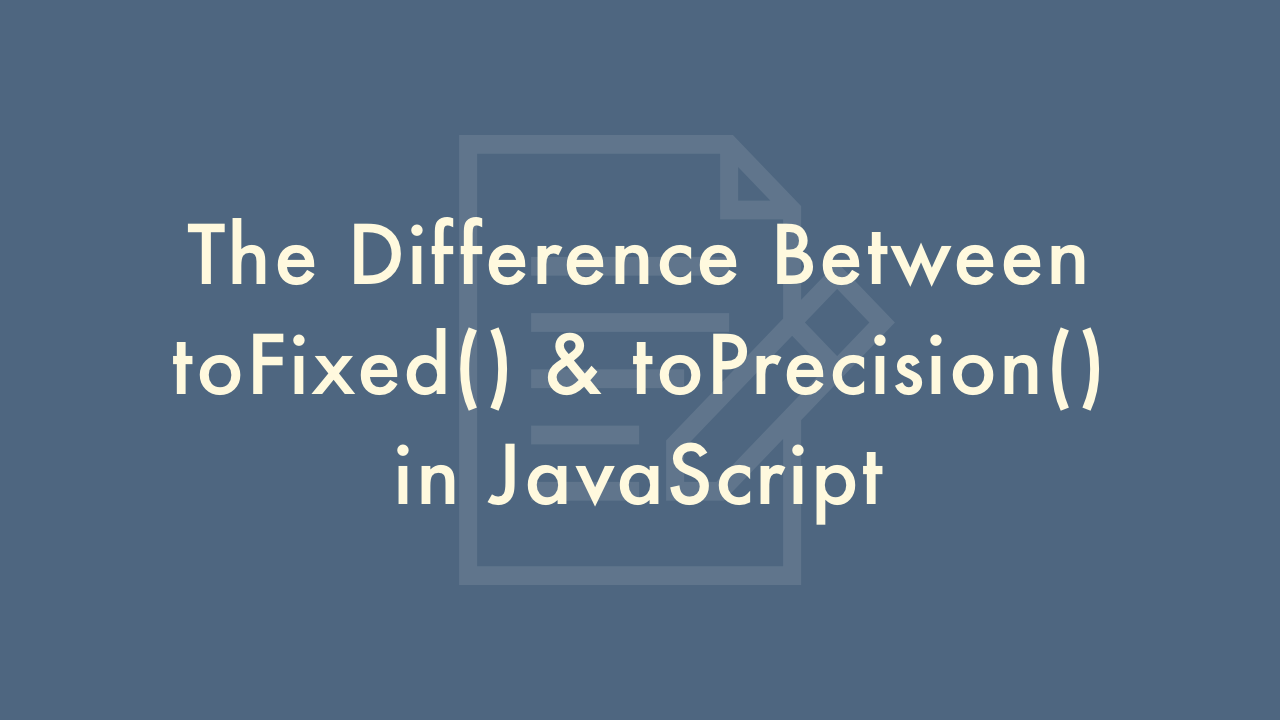
Contents
In this article, you will learn the difference between toFixed() and toPrecision() in JavaScript.
toFixed()
The toFixed () method is a method that executes the process of rounding to the number of digits specified in the argument of the method after the decimal point.
Therefore, specify the number of digits you want to keep in the argument.
Below is a sample.
let value = 123.456
value.toFixed(1);
//result:123.5
value.toFixed(2);
//result:123.46
If the number of digits you want to keep is fixed, use the toFixed () method.
toPrecision()
The toPrecision () method is a method that rounds the entire number including integers and decimals to the number of digits specified in the argument.
Therefore, specify the number of digits of the entire number in the argument.
Below is a sample.
let value = 123.456
value.toPrecision(4);
//result:123.5
value.toPrecision(5);
//result:123.46
If the total number of digits including integers and decimals is fixed, use the toPrecision() method.
What toFixed() and toPrecision() have in common
Basically, the data returned as a result of program execution is often not constant.
As shown in the sample below, if the specified number of digits is not reached, the result is that the shortage is filled with zeros.
let value = 123.456
console.log(value.toFixed(7));
//result:123.4560000
console.log(value.toPrecision(10));
//result:123.4560000