How to Submit a Form in JavaScript
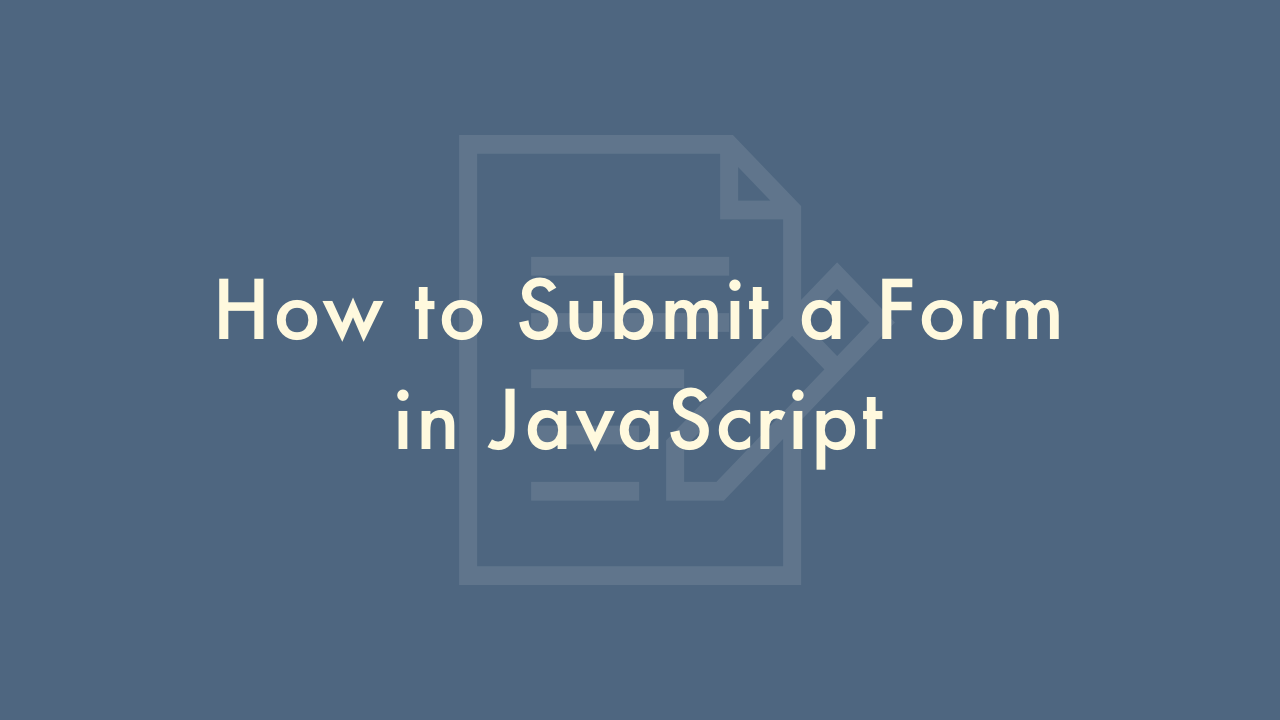
Contents
In this article, you will learn how to submit a form in JavaScript.
Submitting a form
In JavaScript, you can submit a form by accessing the form element and calling the submit() method. This method sends the form data to the server for processing.
const myForm = document.getElementById('my-form');
myForm.submit();
In this example, the getElementById() method is used to get the form element with the ID of my-form. The submit() method is then called on the form element to submit its data to the server.
Submitting a form with a button
You can also submit a form using a button element with the type attribute set to submit. Clicking this button will submit the form.
<form id="my-form">
<input type="text" name="input-1">
<input type="text" name="input-2">
<button type="submit">Submit</button>
</form>
In this example, a form element is created with two input fields and a button element with the type attribute set to submit. Clicking the Submit button will submit the form.
Submitting a form using XMLHttpRequest
You can also submit a form programmatically using the XMLHttpRequest object or the fetch() method. This allows you to submit the form data without reloading the page.
const myForm = document.getElementById('my-form');
const xhr = new XMLHttpRequest();
xhr.open('POST', myForm.action);
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send(new FormData(myForm));
In this example, the getElementById() method is used to get the form element with the ID of my-form. An XMLHttpRequest object is then created and the open() method is called to specify the HTTP method and URL. The setRequestHeader() method is used to set the Content-Type header to application/x-www-form-urlencoded. The onreadystatechange event is then set to a function that logs the response text if the request is successful. Finally, the send() method is called with a FormData object created from the form element to send the form data to the server.
Submitting a form using fetch
const myForm = document.getElementById('my-form');
fetch(myForm.action, {
method: 'POST',
body: new FormData(myForm)
})
.then(response => response.text())
.then(data => console.log(data));
In this example, the getElementById() method is used to get the form element with the ID of my-form. The fetch() method is then called with the form action URL and an options object that specifies the HTTP method and body. The then() method is used to log the response text if the request is successful.