Calculate the Number of Days Between Two Dates with JavaScript
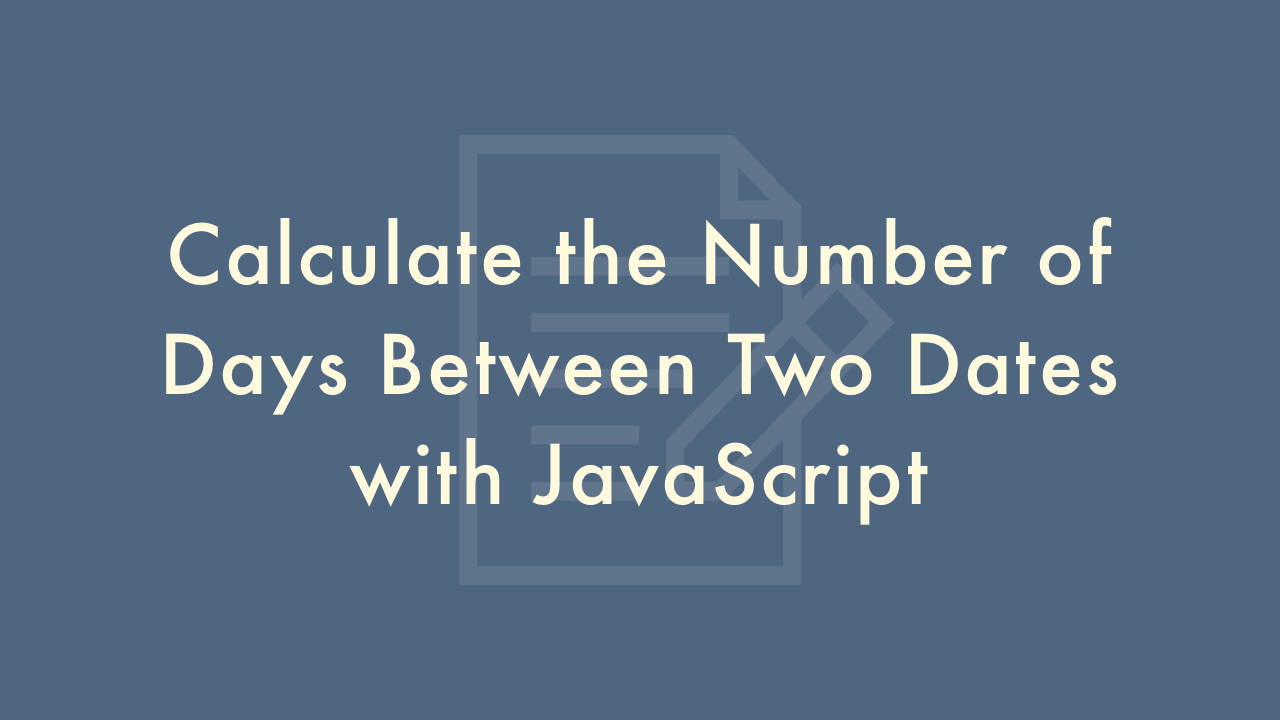
Contents
In this article, you will learn how to calculate the number of days between two dates with JavaScript.
The getTime() method
The getTime method is a method to get how many milliseconds have passed from 0:00:00 on January 1, 1970 to the present.
The value of how many seconds have passed since 0:00:00 on January 1, 1970 is called UNIX time, and it is used in various situations on computers.
For example, you can get how many milliseconds have passed from January 1, 1970 to January 1, 2022 by doing the following.
let now = new Date(2022, 1, 1);
let time = now.getTime();
console.log(time);
Calculate the number of days between two dates
Get the number of milliseconds that the two dates have passed since January 1, 1970, and calculate the difference.
Since you can get the difference in milliseconds for each date, you can divide this value by the value of one day converted to milliseconds to get the difference between the two dates.
let date1 = new Date(2022, 1, 1);
let date2 = new Date(2022, 12, 31);
let termDay = (date2 - date1) / 86400000;
console.log(termDay);
It is possible to know how many days have passed by getting each date as Date type and dividing the difference by 86400000 (1 day converted to milliseconds).