JavaScript AddEventListener Fires Only Once
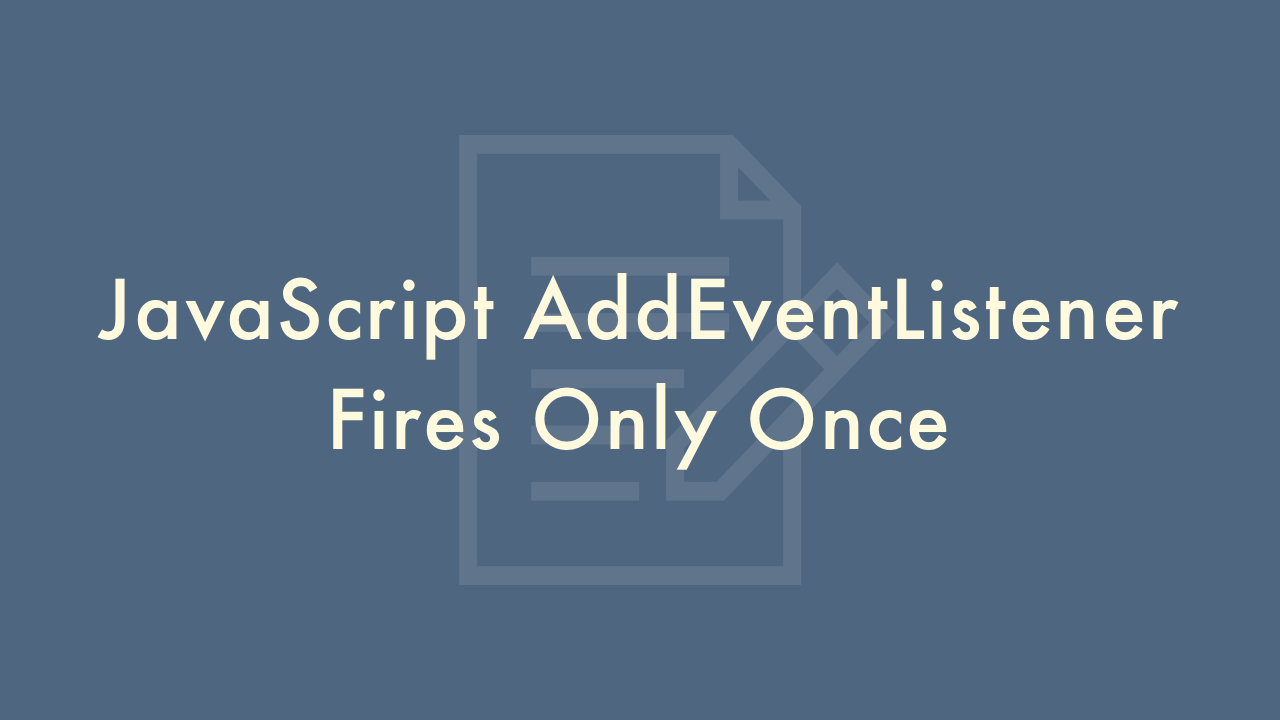
01/20/2022
Contents
In this article, you will learn how to fire JavaScript AddEventListener only once.
Using the once option
The easiest way to execute the process only once is to set once: true to the option of addEventListener.
The following is a sample that executes the process only for the first click.
let btn = document.getElementById('btn');
btn.addEventListener(
'click',
function () {
alert('Clicked!!');
},
{ once: true }
);
However, the once option cannot be used in IE11.
Execute the process only once in IE11
There are two ways to execute the process only once in IE11.
Using the flag
The first is to use a flag.
Below is a sample that sets a flag and branches conditional when the button is clicked.
let clicked;
let btn = document.getElementById('btn');
btn.addEventListener('click', function () {
if (clicked !== true) {
alert('Clicked!');
}
clicked = true;
});
Remove the process after the first execution
The second method is to remove the process after the first execution.
Below is a sample.
let btn = document.getElementById('btn');
function myFunc(){
alert('Clicked!');
btn.removeEventListener('click', myFunc, false);
}
btn.addEventListener('click', myFunc, false);