How to Calculate the Arc Length of a Sector in JavaScript
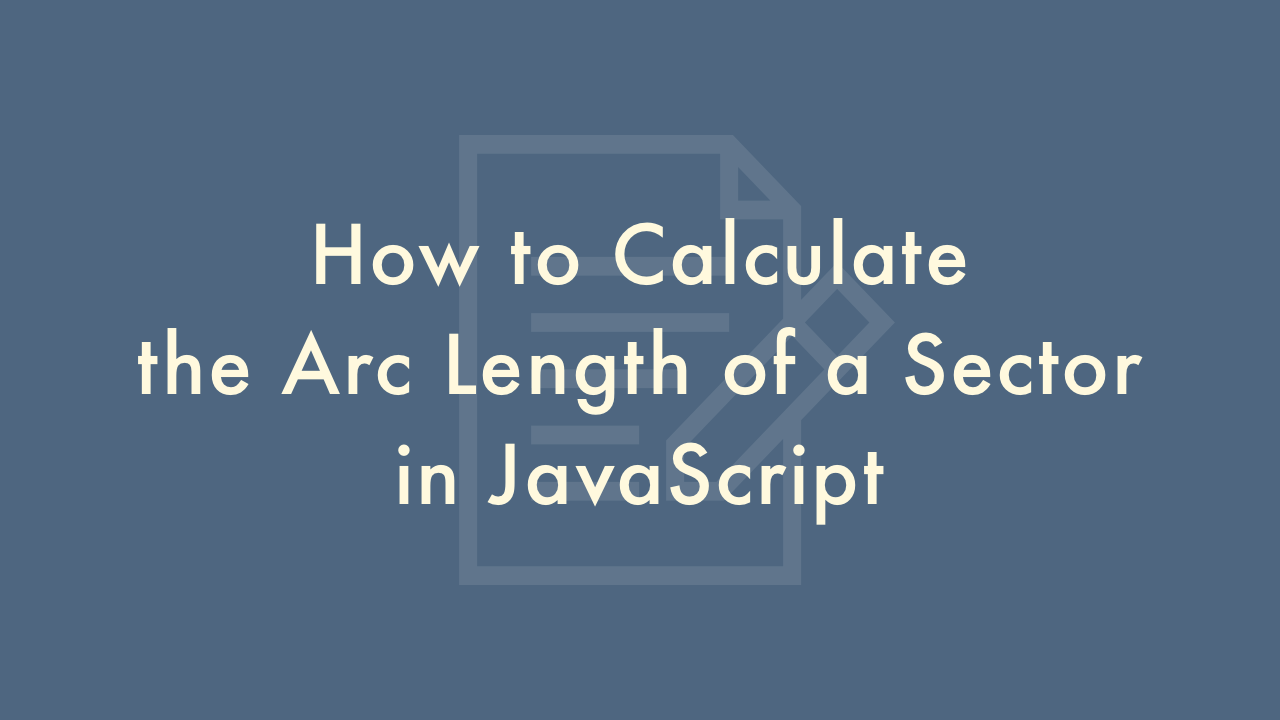
Contents
In this article, you will learn how to calculate the arc length of a sector in JavaScript.
Calculating the arc length of a sector in JavaScript
The arc length of a sector is the length of the portion of the circumference of a circle that corresponds to the central angle of the sector. In JavaScript, we can use the following formula to calculate the arc length of a sector:
Arc Length = (central angle / 360) * 2 * π * r
where central angle is the angle subtended by the sector at the center of the circle (in degrees), r is the radius of the circle, and π is the mathematical constant pi, which is approximately equal to 3.14159.
Examples
Calculating the arc length of a sector with a central angle of 60 degrees and a radius of 5 units
const centralAngle = 60; // in degrees
const radius = 5; // in units
const arcLength = (centralAngle / 360) * 2 * Math.PI * radius;
console.log(arcLength); // 5.235987755982989
In this example, the central angle is 60 degrees, the radius is 5 units, and the output is the arc length of the sector, which is approximately 5.236 units.
Calculating the arc length of a sector with a central angle of 120 degrees and a radius of 3.5 units
const centralAngle = 120; // in degrees
const radius = 3.5; // in units
const arcLength = (centralAngle / 360) * 2 * Math.PI * radius;
console.log(arcLength); // 7.266478599682293
In this example, the central angle is 120 degrees, the radius is 3.5 units, and the output is the arc length of the sector, which is approximately 7.266 units.
Calculating the arc length of a semicircle with a radius of 10 units
const centralAngle = 180; // in degrees (since it's a semicircle)
const radius = 10; // in units
const arcLength = (centralAngle / 360) * 2 * Math.PI * radius;
console.log(arcLength); // 31.41592653589793
In this example, the central angle is 180 degrees (since it’s a semicircle), the radius is 10 units, and the output is the arc length of the semicircle, which is approximately 31.416 units.
Note: If you have the length of the arc and you want to calculate the central angle, you can rearrange the formula as follows:
Central Angle (in degrees) = (Arc Length / (2 * π * r)) * 360