Parse XML and JSON with JavaScript
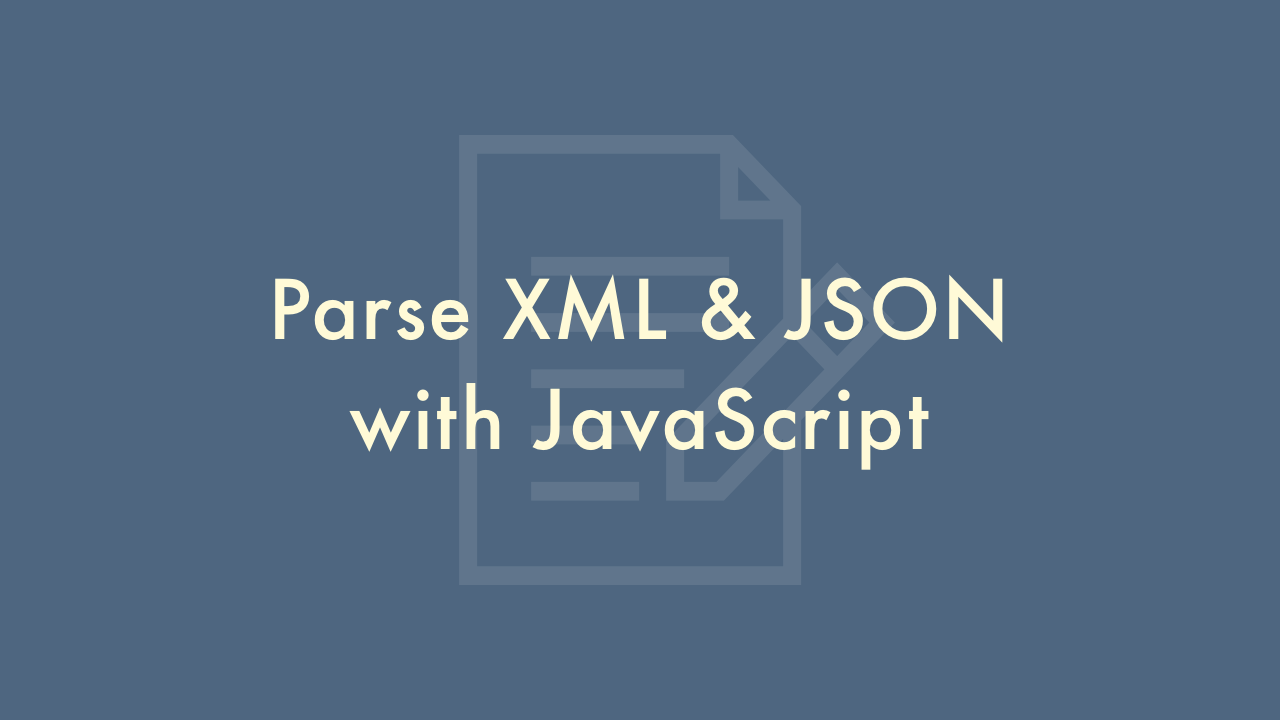
02/02/2022
Contents
In this article, you will learn how to parse XML with JavaScript.
Features of XML format and JSON format
The features of each are as follows.
Common features include nesting, arrays, and other iterations.
XML format
Features
- Define data using start and end tags
- Larger number of characters (data volume) than JSON format
Sample
XML defines data items by tag name as shown below, and the value enclosed by the start tag and end tag represents the data.
<?xml version="1.0"?>
<school>
<schoolName>Plantpot</schoolName>
<student>
<studentId>1</studentId>
<name>foo</name>
<birthday>20100101</birthday>
</student>
<student>
<studentId>2</studentId>
<name>bar</name>
<birthday>20100102</birthday>
</student>
</school>
JSON format
Features
- Define data by “definition name: value”
- The number of characters (data volume) is smaller than the XML format
Sample
JSON defines data with a pair of definition name and value as follows
{
"school":
{
"schoolName":"Plantpot",
"student":
[
{
"studentId":"1",
"name":"foo",
"birthday":"20100101"
},
{
"studentId":"2",
"name":"bar",
"birthday":"20100102"
}
]
}
}
Parse XML and JSON
Converting the object format is called parsing.
Character strings representing XML / JSON can be converted to each format.
The conversion processes are explained below.
Converting an XML string to an XML document
const strXml = '<school>
<schoolName>Plantpot</schoolName>
</school >';
const parser = new DOMParser();
let xmlData = parser.parseFromString(strXml,"text/xml");
console.log(xmlData);
Conversion from JSON string to JSON object
const strJson = '{
"school":{
"schoolName":"Plantpot"
}
}';
let jsonData = JSON.parse(strJson);
console.log(jsonData);