Get the Width and Height of an Element with JavaScript
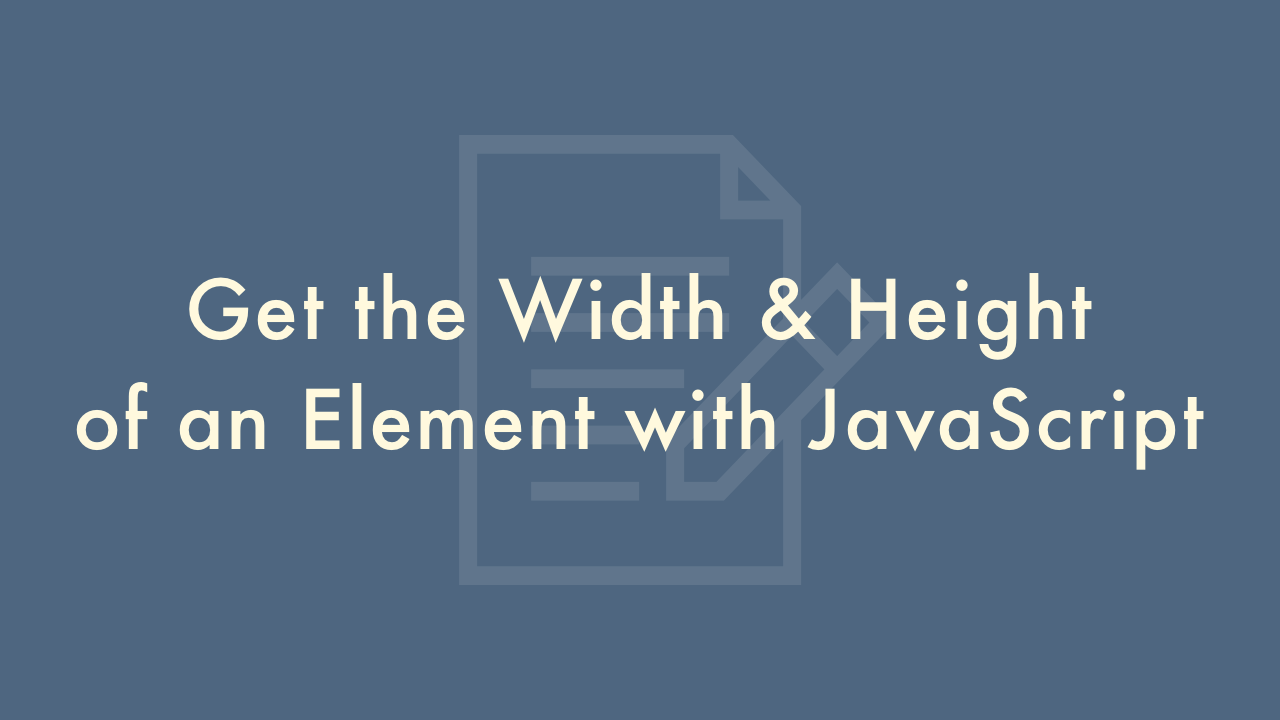
Contents
In this article, you will learn how to get the width and height of an element with JavaScript.
offsetWidth and offsetHeight
The offsetWidth and offsetHeight properties return the visible width and height of an element in pixels, including padding, border and scrollbar (if rendered), but not margin.
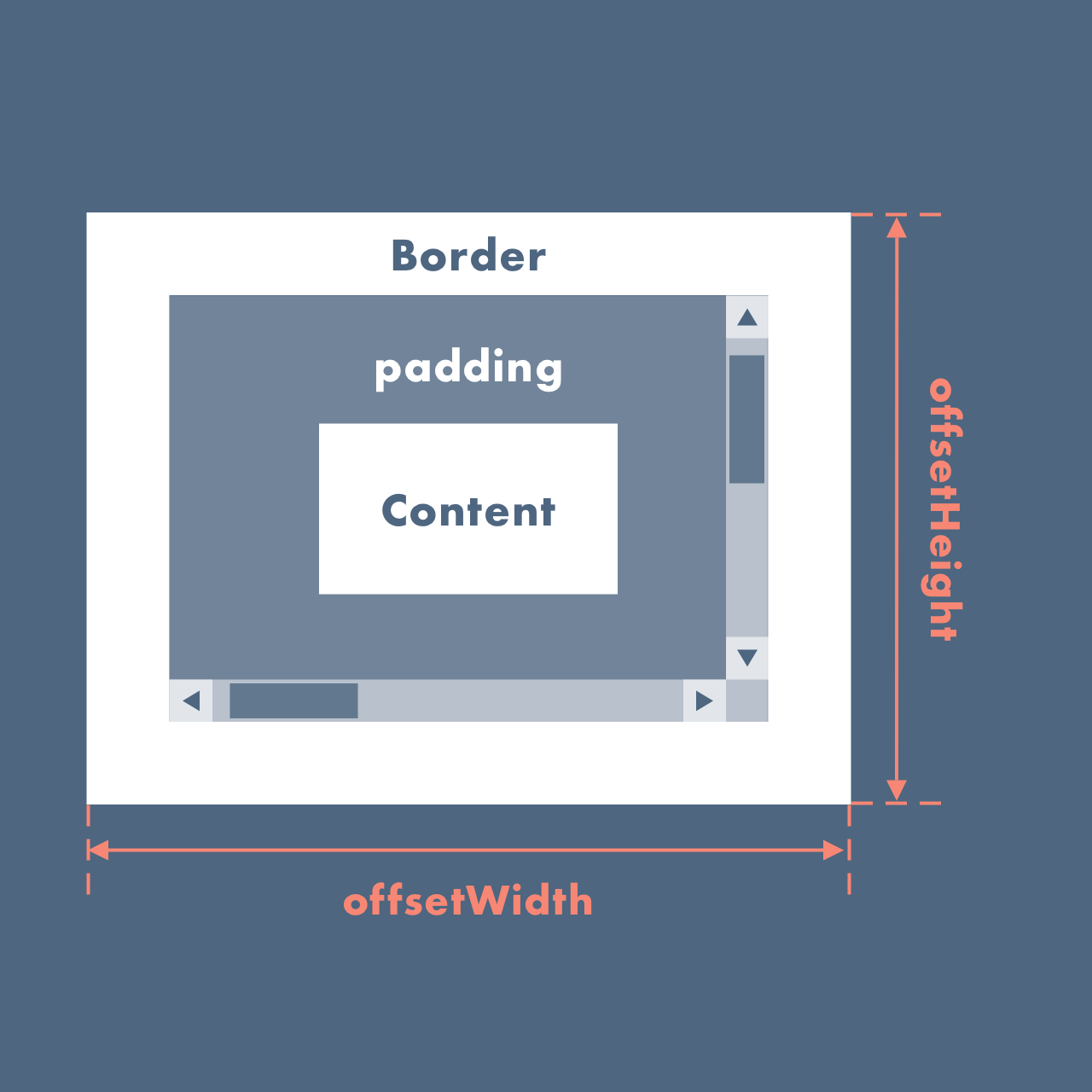
Demo
Code
HTML
<div id="el">Content</div>
<p class="size">
offsetWidth = <span id="offsetWidth"></span><br>
offsetHeight = <span id="offsetHeight"></span><br>
</p>
CSS
#el {
width: 100px;
height: 50px;
margin: 10px;
padding: 10px;
border: 10px solid #333;
}
JavaScript
const element = document.getElementById('el');
const oWidth = document.getElementById('offsetWidth');
const oHeight = document.getElementById('offsetHeight');
// width + padding-left + padding-right + border-left + border-right
oWidth.innerHTML = element.offsetWidth; // 100 + 10 + 10 + 10 + 10 = 140
// height + padding-top + padding-bottom + border-top + border-bottom
oHeight.innerHTML = element.offsetHeight; // 50 + 10 + 10 + 10 + 10 = 90
clientWidth and clientHeight
The clientWidth and clientHeight properties return the visible width and height of an element in pixels, including padding, but not border, scrollbar (if rendered) and margin.
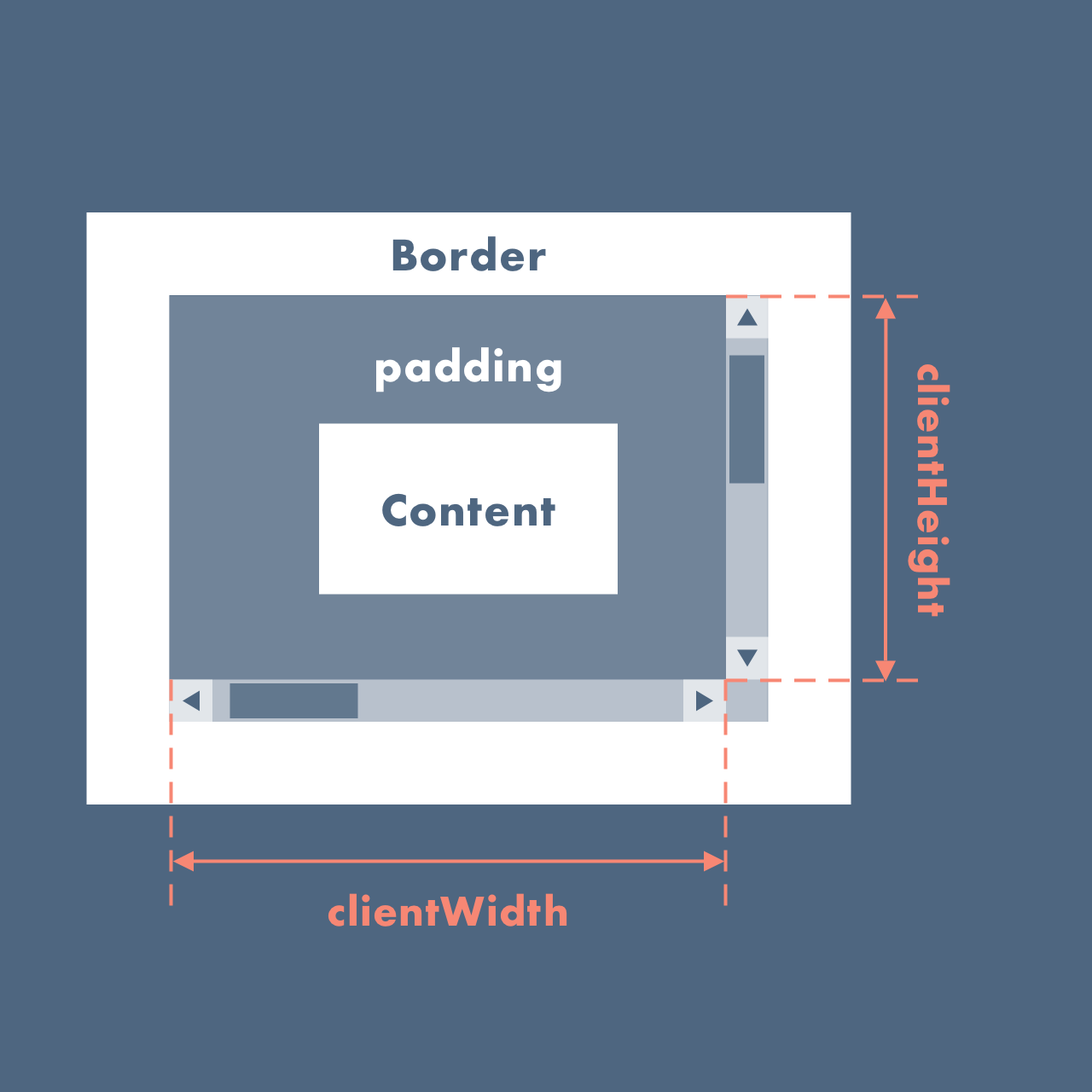
Demo
Code
HTML
<div id="el">Content</div>
<p class="size">
clientWidth = <span id="clientWidth"></span><br>
clientHeight = <span id="clientHeight"></span><br>
</p>
CSS
#el {
width: 100px;
height: 50px;
margin: 10px;
padding: 10px;
border: 10px solid #333;
}
JavaScript
const element = document.getElementById('el');
const cWidth = document.getElementById('clientWidth');
const cHeight = document.getElementById('clientHeight');
// width + padding-left + padding-right
cWidth.innerHTML = element.clientWidth; // 100 + 10 + 10 = 120
// height + padding-top + padding-bottom
cHeight.innerHTML = element.clientHeight; // 50 + 10 + 10 = 70
getBoundingClientRect()
The width and height properties of the getBoundingClientRect() method return the visible width and height of an element in pixels, including padding, border and scrollbar (if rendered), but not margin.
In case of transforms, if width: 100px; and transform: scale(0.5); are set for the element, the getBoundingClientRect() will return 50 as the width, while the offsetWidth will return 100.
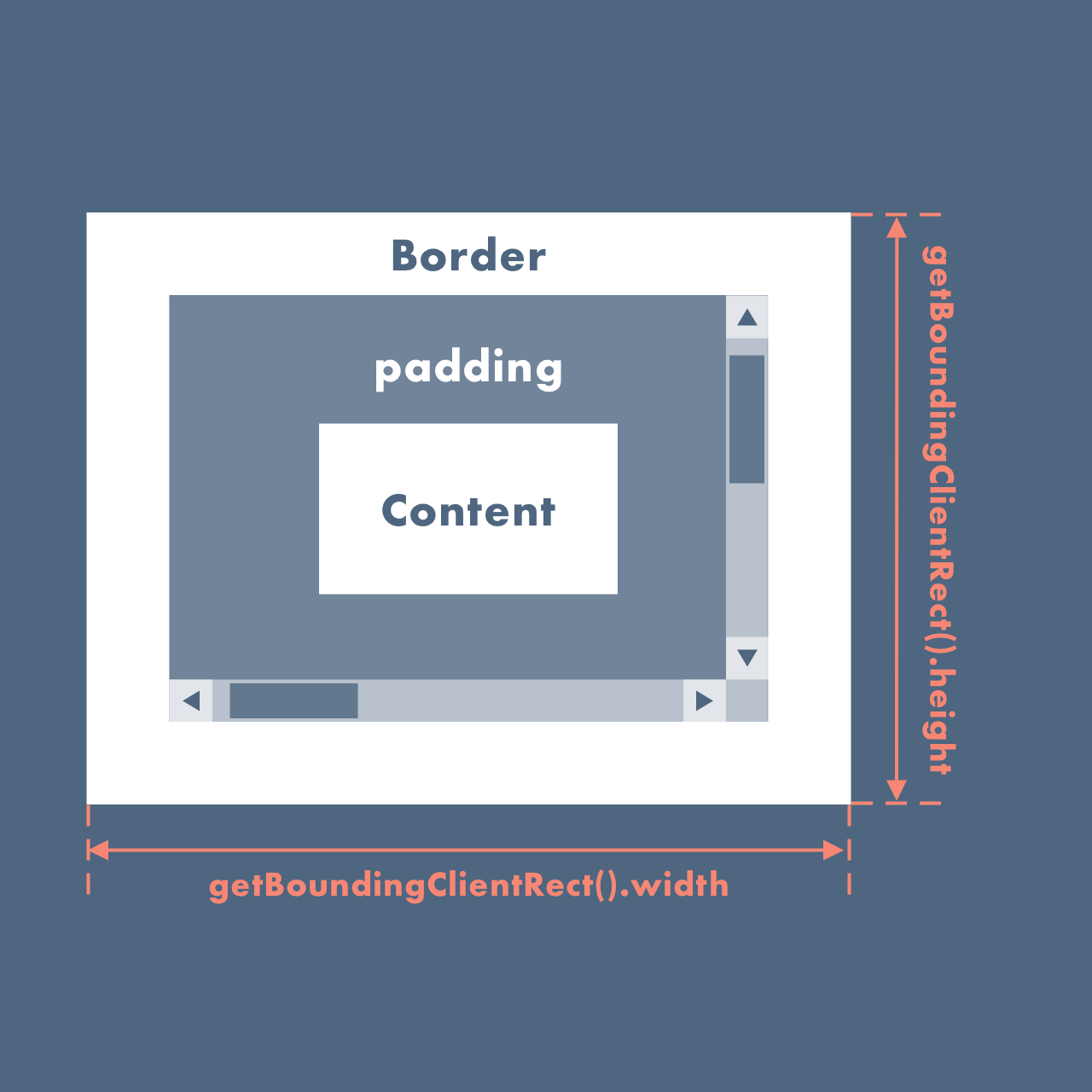
Demo
Code
HTML
<div id="el">Content</div>
<p class="size">
rectWidth = <span id="rectWidth"></span><br>
rectHeight = <span id="rectHeight"></span><br>
</p>
CSS
#el {
width: 100px;
height: 50px;
margin: 10px;
padding: 10px;
border: 10px solid #333;
}
JavaScript
const element = document.getElementById('el');
const rWidth = document.getElementById('rectWidth');
const rHeight = document.getElementById('rectHeight');
// width + padding-left + padding-right + border-left + border-right
rWidth.innerHTML = element.getBoundingClientRect().width; // 100 + 10 + 10 + 10 + 10 = 140
// height + padding-top + padding-bottom + border-top + border-bottom
rHeight.innerHTML = element.getBoundingClientRect().height; // 50 + 10 + 10 + 10 + 10 = 90
getComputedStyle()
The width and height properties of the getComputedStyle() method return the CSS width and height values of an element in pixels, not including padding, border, scrollbar (if rendered) and margin.
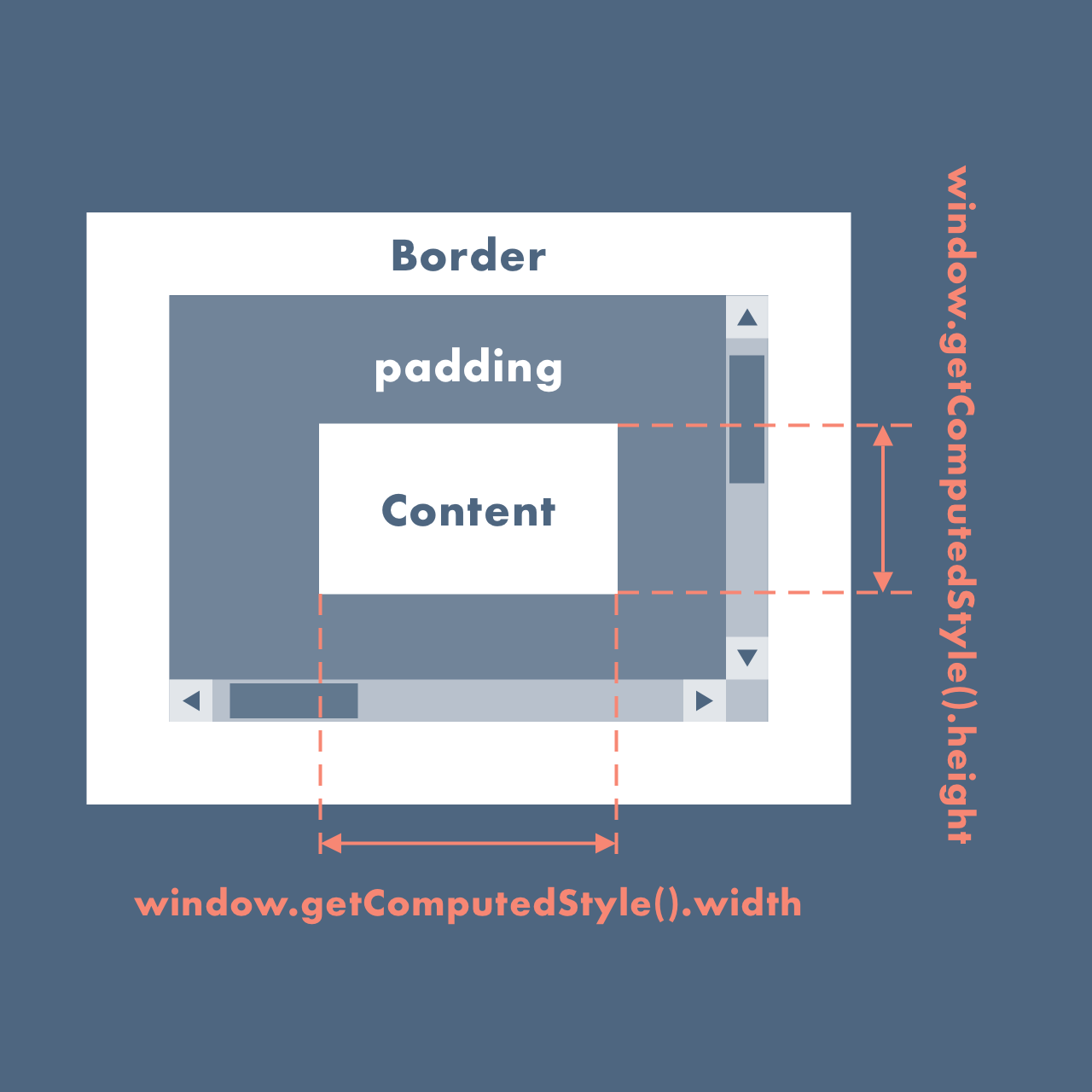
Demo
Code
HTML
<div id="el">Content</div>
<p class="size">
Width = <span id="width"></span><br>
Height = <span id="height"></span><br>
</p>
CSS
#el {
width: 100px;
height: 50px;
margin: 10px;
padding: 10px;
border: 10px solid #333;
}
JavaScript
const element = document.getElementById('el');
const Width = document.getElementById('width');
const Height = document.getElementById('height');
Width.innerHTML = parseInt(window.getComputedStyle(element).width); // 100
Height.innerHTML = parseInt(window.getComputedStyle(element).height); // 50
scrollWidth and scrollHeight
The scrollWidth and scrollHeight properties return the width and height of an element’s content in pixels, including padding, but not border, scrollbar (if rendered) and margin.
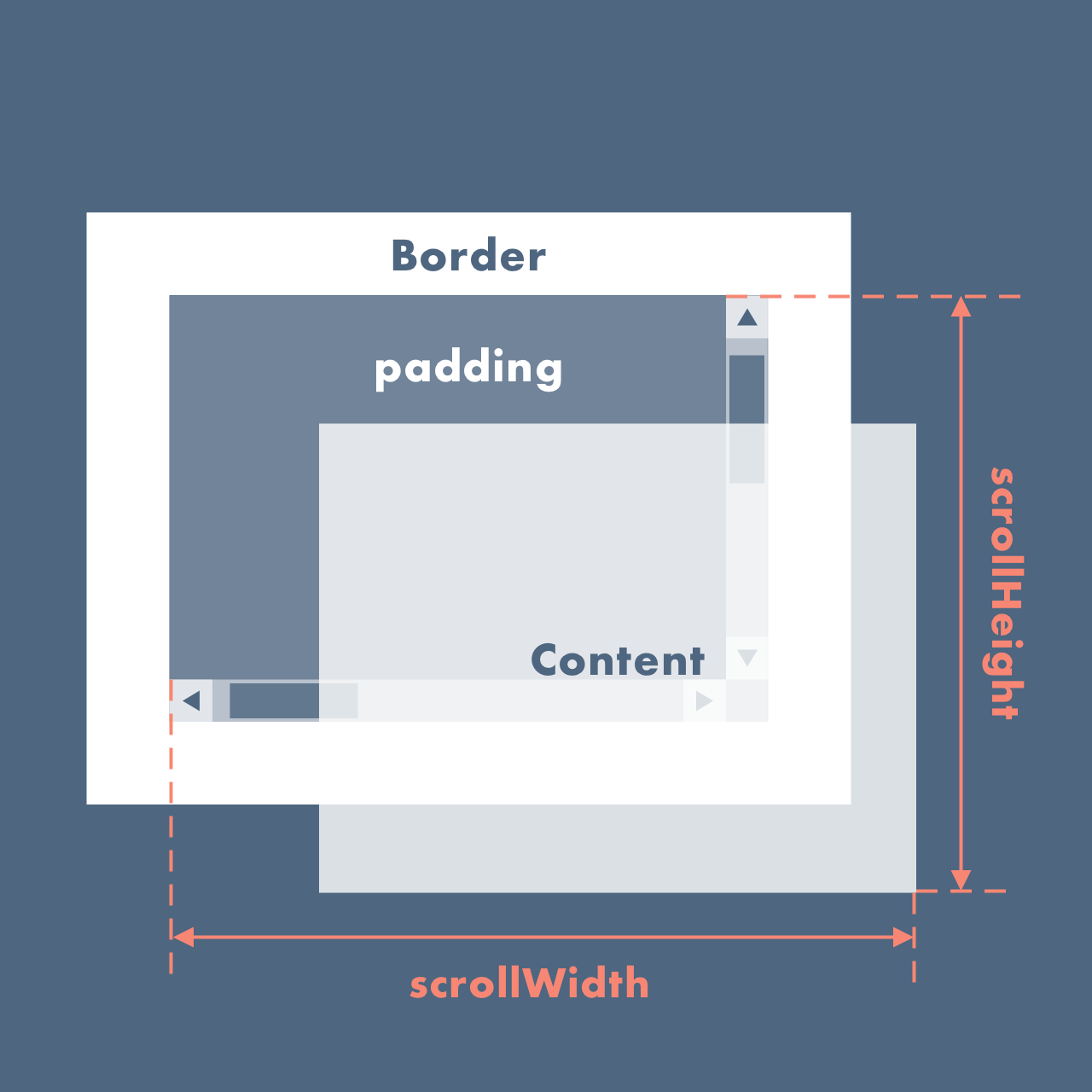
Demo
Code
HTML
<div id="el">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.<br>
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
</p>
</div>
<p class="size">
scrollWidth = <span id="scrollWidth"></span><br>
scrollHeight = <span id="scrollHeight"></span><br>
</p>
CSS
#el {
width: 100px;
height: 50px;
margin: 10px;
padding: 10px;
border: 10px solid #333;
}
#el p {
width: 200px;
height: 200px;
}
JavaScript
const element = document.getElementById('el');
const sWidth = document.getElementById('scrollWidth');
const sHeight = document.getElementById('scrollHeight');
sWidth.innerHTML = element.scrollWidth;
sHeight.innerHTML = element.scrollHeight;