Simple Accordion Menu with HTML, CSS & JavaScript
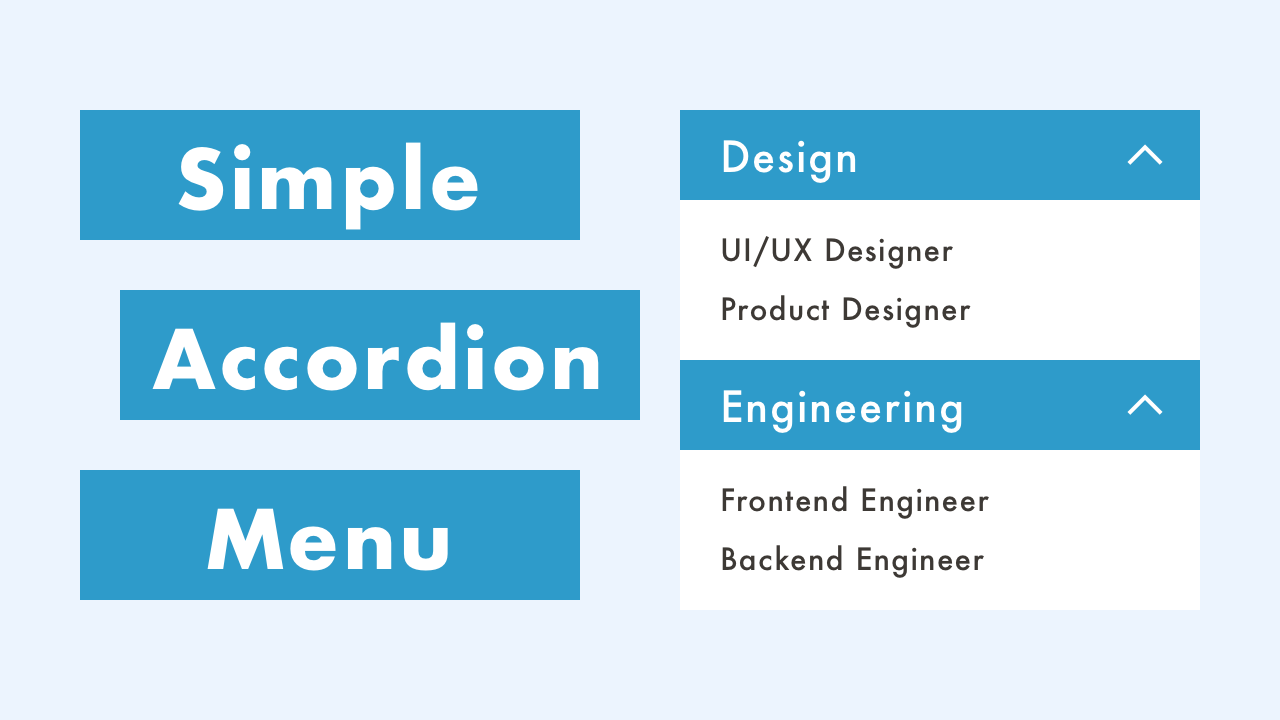
02/28/2021
Contents
Demo
Code
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<title>Accordion Menu</title>
<link rel="stylesheet" type="text/css" href="https://demo.plantpot.works/assets/css/normalize.css">
<link rel="stylesheet" href="https://use.typekit.net/opg3wle.css">
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="container">
<div class="box">
<div class="acd-menu">
<button type="button" class="btn-acd">Design<span></span></button>
<ul>
<li><a href="#">UI/UX Designer</a></li>
<li><a href="#">Product Designer</a></li>
</ul>
</div>
<div class="acd-menu">
<button type="button" class="btn-acd">Engineering<span></span></button>
<ul>
<li><a href="#">Frontend Engineer</a></li>
<li><a href="#">Backend Engineer</a></li>
</ul>
</div>
</div>
</div>
</body>
</html>
CSS
@charset "utf-8";
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
html {
font-size: 16px;
}
body {
background-color: #ecf4fe;
font-family: futura-pt, sans-serif;
-webkit-tap-highlight-color: rgba(0,0,0,0);
}
#container {
display: flex;
justify-content: center;
align-items: center;
position: relative;
width: 100%;
height: 100vh;
}
.box {
display: flex;
flex-direction: column;
justify-content: flex-start;
align-items: center;
position: relative;
width: 240px;
height: 240px;
}
.acd-menu {
width: 100%;
}
.btn-acd {
display: flex;
justify-content: space-between;
align-items: center;
border: none;
border-radius: 0;
width: 100%;
padding: 10px 20px;
border-bottom: 1px solid #fff;
background-color: #2e9bca;
color: #fff;
font-size: 1.25rem;
letter-spacing: 2px;
text-align: left;
outline: none;
cursor: pointer;
}
.btn-acd span {
-webkit-transform: rotate(45deg);
transform: rotate(45deg);
width: 10px;
height: 10px;
margin-bottom: 5px;
border-right: 2px solid #fff;
border-bottom: 2px solid #fff;
}
.btn-acd:hover {
opacity: .9;
}
.acd-menu ul {
display: none;
list-style: none;
padding: 10px 0;
background-color: #fff;
}
.acd-menu ul li {
padding: 5px 20px;
font-size: 1rem;
text-align: left;
cursor: pointer;
}
.acd-menu ul li a {
display: block;
color: #3d3935;
text-decoration: none;
}
.acd-menu ul li:hover a {
color: #2e9bca;
}
.acd-menu.is-open ul {
display: block;
}
.acd-menu.is-open .btn-acd span {
-webkit-transform: rotate(-135deg);
transform: rotate(-135deg);
margin-bottom: 0;
}
JavaScript
var btns = document.querySelectorAll('.btn-acd');
btns.forEach(btn => {
btn.addEventListener('click', () => {
if(!btn.parentNode.classList.contains("is-open")) {
btn.parentNode.classList.add("is-open");
} else {
btn.parentNode.classList.remove("is-open");
}
});
});