How to Use the isFinite() Function in JavaScript
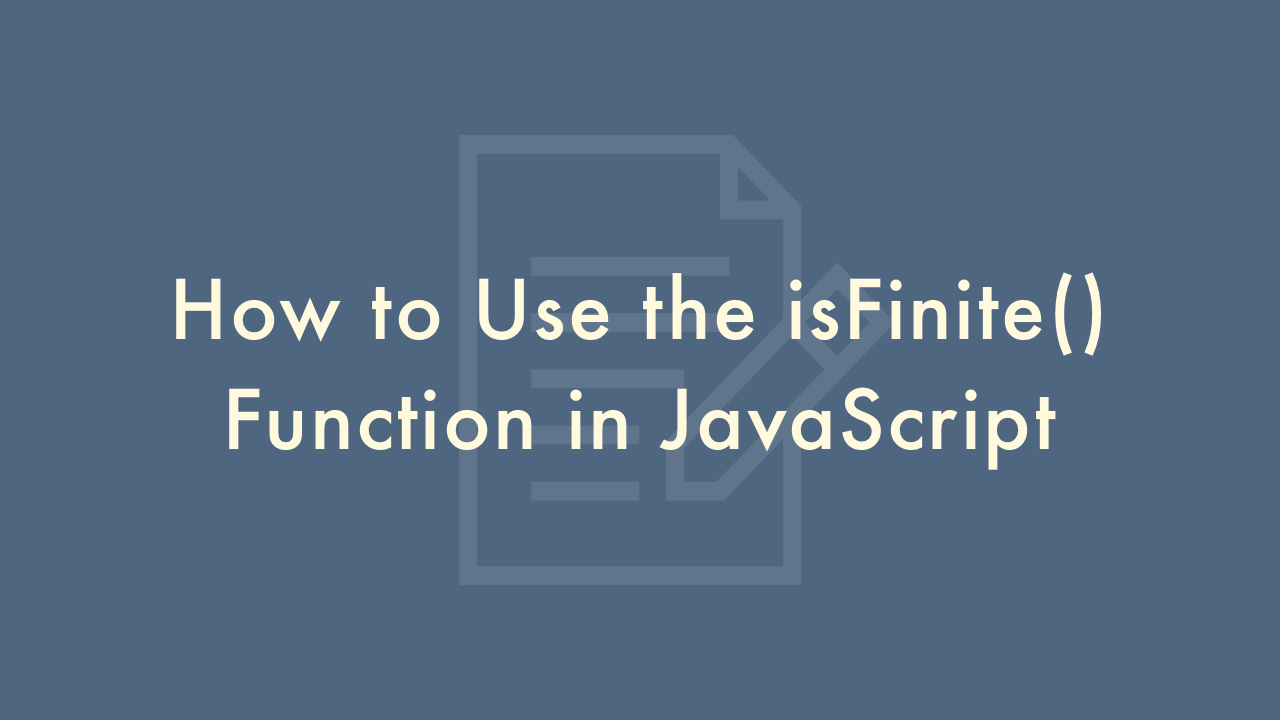
Contents
In this article, you will learn how to use the isFinite() function in JavaScript.
The isFinite() Function
The isFinite() function in JavaScript is a built-in method that allows you to determine if a given value is a finite number or not. It returns true if the argument passed to it is a finite number, and false otherwise. The function works with both numeric literals and variables, and it is particularly useful when working with user input or when performing mathematical operations.
Here’s how to use the isFinite() function in JavaScript:
Syntax
The syntax for the isFinite() function is as follows:
isFinite(value)
The value parameter can be any number or numeric expression that you want to test for finiteness.
Example
Let’s say you want to test whether a user’s input is a finite number. You can use the isFinite() function to check this. Here’s an example:
let userInput = prompt("Enter a number:");
if (isFinite(userInput)) {
console.log("The input is a finite number.");
} else {
console.log("The input is not a finite number.");
}
In this example, the prompt() method is used to display a dialog box where the user can enter a number. The isFinite() function is then used to check whether the input is a finite number. If the input is finite, the message “The input is a finite number.” is displayed in the console. Otherwise, the message “The input is not a finite number.” is displayed.
Limitations
It’s important to note that the isFinite() function has some limitations. For example, it cannot be used to test for NaN (Not-a-Number) or Infinity values. To test for these values, you can use the isNaN() and isFinite() functions, respectively.
let x = NaN;
let y = Infinity;
console.log(isNaN(x)); // true
console.log(isNaN(y)); // false
console.log(isFinite(x)); // false
console.log(isFinite(y)); // false
In this example, the isNaN() function is used to test whether x is NaN (which returns true). The isFinite() function is used to test whether x and y are finite numbers (which returns false for both).