How to Get a Character at a Specific Position in a String in JavaScript
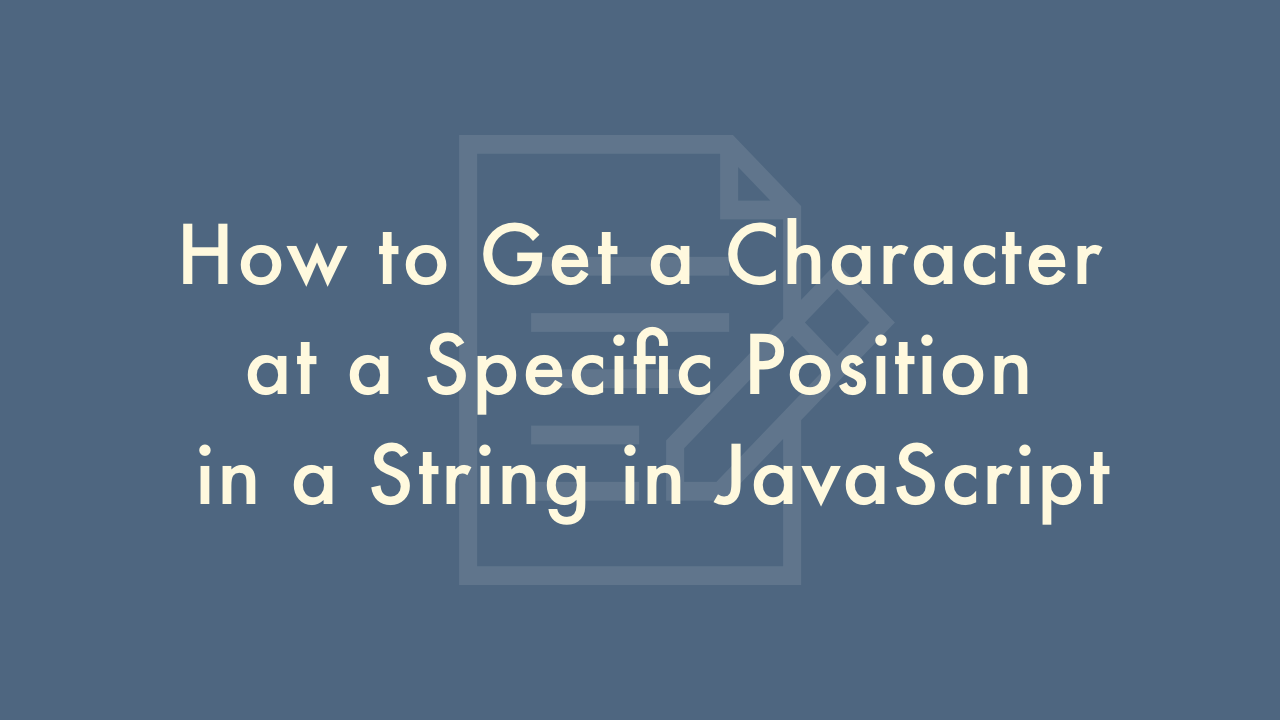
Contents
In this article, you will learn how to get a character at a specific position in a string in JavaScript.
Getting a character at a specific position in a string in JavaScript
In JavaScript, you can get a character at a specific position in a string using the charAt() and [] operators.
Using the charAt() method
The charAt() method returns the character at a specified index in a string. The method takes one argument: index. The index argument specifies the position of the character to be returned. The first character of a string has an index of 0.
Example
const str = "Hello, world!";
const result = str.charAt(7);
console.log(result); // "w"
In this example, we retrieved the character “w” from the original string “Hello, world!” using the charAt() method.
Using the [] operator
In JavaScript, you can use the [] operator to access a character at a specific position in a string. The [] operator takes one argument: index. The index argument specifies the position of the character to be returned. The first character of a string has an index of 0.
Example
const str = "Hello, world!";
const result = str[7];
console.log(result); // "w"
In this example, we retrieved the character “w” from the original string “Hello, world!” using the [] operator.