How to Get All the Class Names of a DOM Element in JavaScript
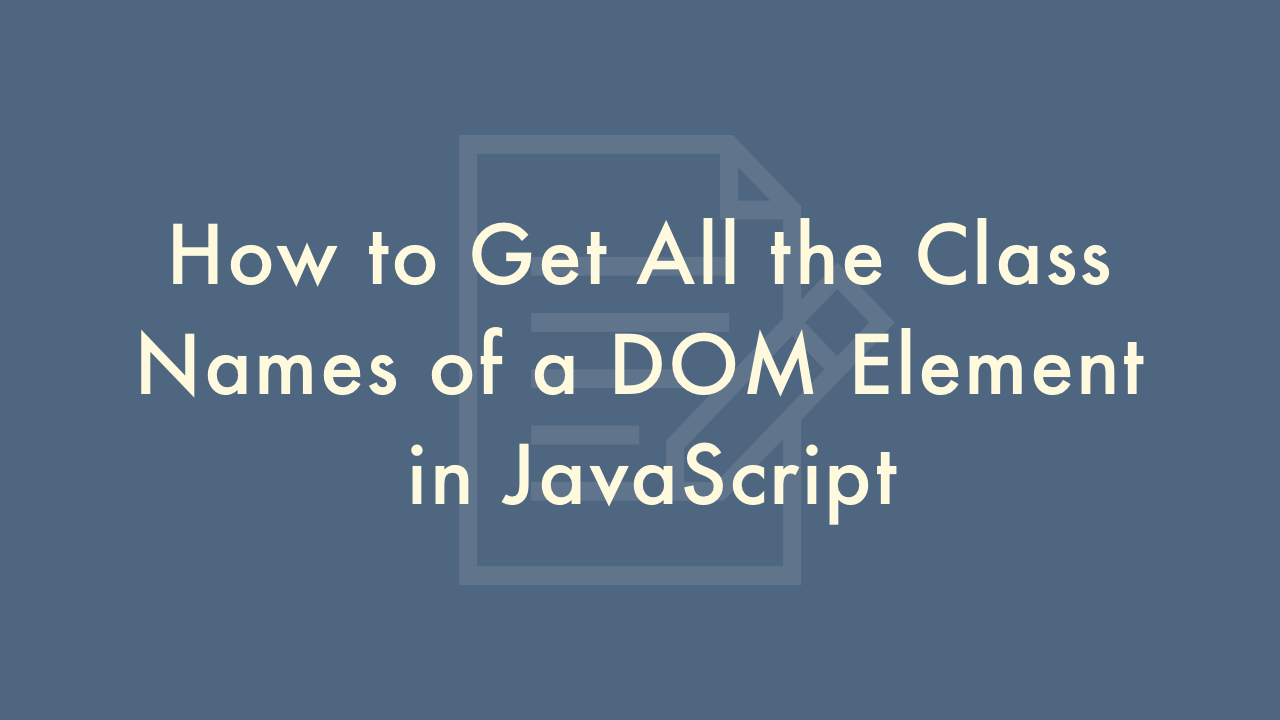
Contents
In this article, you will learn how to get all the class names of a DOM element in JavaScript.
Getting all the class names of a DOM element in JavaScript
In JavaScript, you can get all the class names of a DOM element using various methods. Here are some of them:
Using the className property
This property returns a string containing all the class names of an element. You can split the string to get an array of class names.
Example
const element = document.getElementById('myElement');
const classNames = element.className.split(' ');
console.log(classNames); // Output: an array of class names
Using the classList property
This property returns a DOMTokenList object that contains all the class names of an element. You can convert this object to an array using the Array.from() method or the spread operator.
Example
const element = document.getElementById('myElement');
const classList = Array.from(element.classList);
console.log(classList); // Output: an array of class names
or
const element = document.getElementById('myElement');
const classList = [...element.classList];
console.log(classList); // Output: an array of class names
Using the getAttribute() method
This method returns the value of the specified attribute of an element. You can use it to get the value of the class attribute and split it to get an array of class names.
Example
const element = document.getElementById('myElement');
const classNames = element.getAttribute('class').split(' ');
console.log(classNames); // Output: an array of class names
Note that the className and classList properties will only work for elements that have a class attribute. If an element does not have a class attribute, these properties will return an empty string or a DOMTokenList object with zero length.