Convert a String to an Integer with JavaScript parseInt()
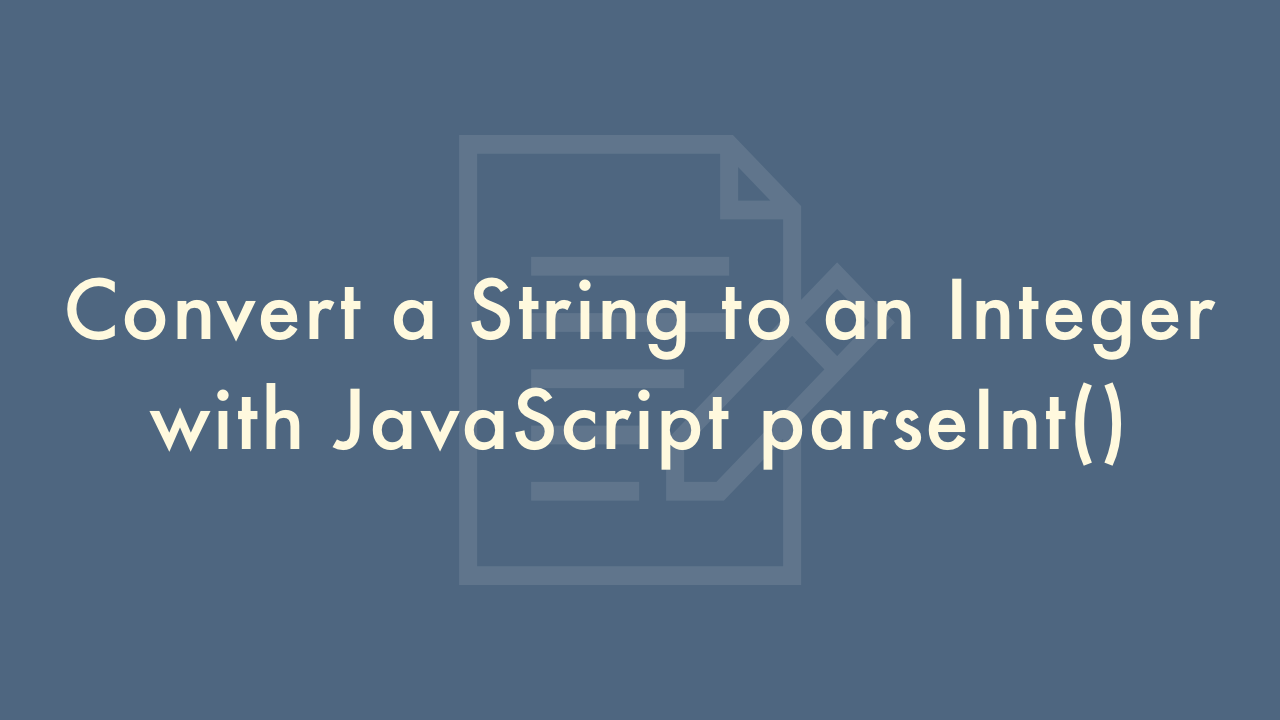
Contents
In this article, you will learn how to convert a string to an integer with JavaScript parseInt().
The parseInt() function
The parseInt() function is a JavaScript global function that converts a string to an integer.
A global function means a function that can be accessed and used from anywhere in a JavaScript program.
JavaScript is a programming language that you can use without worrying about the difference in data types.
A program written in JavaScript captures the contents of functions and operations before and after it, and automatically converts them into appropriate data types.
At first glance it’s great, but on the other hand it causes problems.
Therefore, you can avoid unknown problems by defining the data type firmly instead of having the data type converted automatically.
To do this, we use the parseInt function to convert the string to a variable and define it as an integer.
The syntax is below.
parseInt(str)
parseInt(str, int)
praseInt(str)
The str is an abbreviation for string.
If you write as below, the string 123 will convert the data type to the integer 123.
parseInt("123");
parseInt(str, int)
The int part is the radix.
The radix can be binary, octal, decimal, or hexadecimal.
Below is a sample of converting a string to an integer in each radix.
//Convert 1000 displayed in binary to decimal
parseInt("1000", 2);
//Convert 010 displayed in octal to decimal
parseInt("010", 8);
//Convert 8 displayed in decimal to decimal
parseInt("8", 10);
//Convert 0x8 displayed in hexadecimal to decimal
parseInt("0x8", 16);
All display results will be “8”.
Similar functions to the parseInt() function
Similar functions to the parseInt() function include the parseFloat() function and the Number() function.
The parseFloat() function
It is possible to use floating point literals, unlike the parseInt() function.
Floating point is a decimal notation that displays 1.0 if it is an integer value of 1.
The Number() function
The Number() function can handle floating point like the parseFloat() function.
Unlike the parseInt() function and the parseFloat() function, it cannot handle numbers with mixed strings, but it can parse objects.