How to Get the tBody Elements of a Table in JavaScript
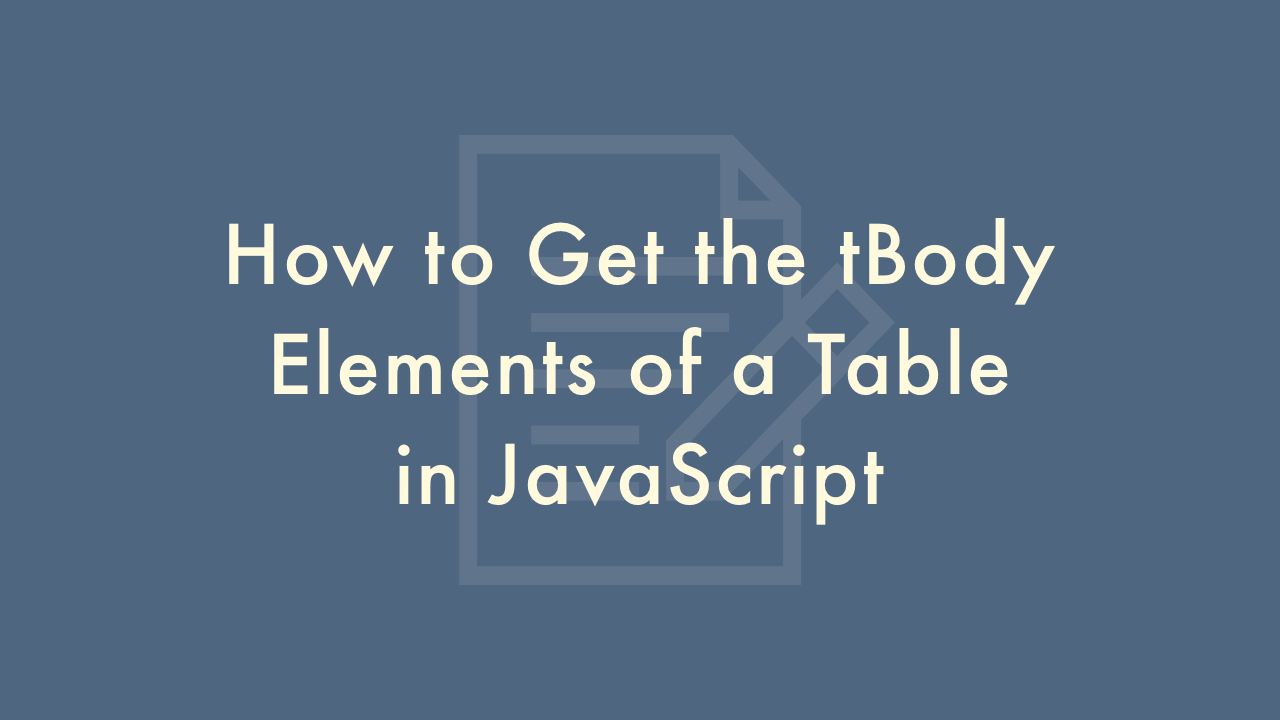
Contents
In this article, you will learn how to get the tbody elements of a table in JavaScript.
Getting the tbody elements of a table in JavaScript
If you want to get the <tbody> elements of a table using JavaScript, you can use several methods. Here are some of the most common approaches.
Using the tBodies property
The HTMLTableElement interface has a tBodies property that returns a collection of <tbody> elements of the table. Here’s an example:
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1, Column 1</td>
<td>Row 1, Column 2</td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
</tr>
</tbody>
<tbody>
<tr>
<td>Row 3, Column 1</td>
<td>Row 3, Column 2</td>
</tr>
<tr>
<td>Row 4, Column 1</td>
<td>Row 4, Column 2</td>
</tr>
</tbody>
</table>
const table = document.querySelector("table");
const tbodies = table.tBodies;
console.log(tbodies); // a collection of <tbody> elements
In this example, we first select the table element using document.querySelector(). We then access the tBodies property of the table element.
Using the getElementsByTagName() method
You can also use the getElementsByTagName() method to get a collection of <tbody> elements. Here’s an example:
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1, Column 1</td>
<td>Row 1, Column 2</td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
</tr>
</tbody>
<tbody>
<tr>
<td>Row 3, Column 1</td>
<td>Row 3, Column 2</td>
</tr>
<tr>
<td>Row 4, Column 1</td>
<td>Row 4, Column 2</td>
</tr>
</tbody>
</table>
const table = document.querySelector("table");
const tbodies = table.getElementsByTagName("tbody");
console.log(tbodies); // a collection of <tbody> elements
In this example, we first select the table element using document.querySelector(). We then use the getElementsByTagName() method to get a collection of <tbody> elements.
Using the querySelectorAll() method
You can also use the querySelectorAll() method to select <tbody> elements directly using a CSS selector. Here’s an example:
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1, Column 1</td>
<td>Row 1, Column 2</td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
</tr>
</tbody>
<tbody>
<tr>
<td>Row 3, Column 1</td>
<td>Row 3, Column 2</td>
</tr>
<tr>
<td>Row 4, Column 1</td>
<td>Row 4, Column 2</td>
</tr>
</tbody>
</table>
const tbodies = document.querySelectorAll("table tbody");
console.log(tbodies); // a collection of <tbody> elements
In this example, we use the querySelectorAll() method to select all <tbody> elements that are descendants of the table element. We then get a collection of <tbody> elements.
Using the rows property
Another approach is to use the rows property of the <tbody> element to get a collection of <tr> elements. Here’s an example:
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1, Column 1</td>
<td>Row 1, Column 2</td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
</tr>
</tbody>
<tbody>
<tr>
<td>Row 3, Column 1</td>
<td>Row 3, Column 2</td>
</tr>
<tr>
<td>Row 4, Column 1</td>
<td>Row 4, Column 2</td>
</tr>
</tbody>
</table>
const tbody = document.getElementsByTagName("tbody")[0];
const rows = tbody.rows;
console.log(rows); // a collection of <tr> elements
In this example, we first select the <tbody> element using document.getElementsByTagName(). We then use the rows property to get a collection of <tr> elements in that <tbody>.
Note that the rows property returns a collection of <tr> elements, not <tbody> elements. To get a collection of <tbody> elements, you can use any of the previous methods.