Background Animation with Anime.js
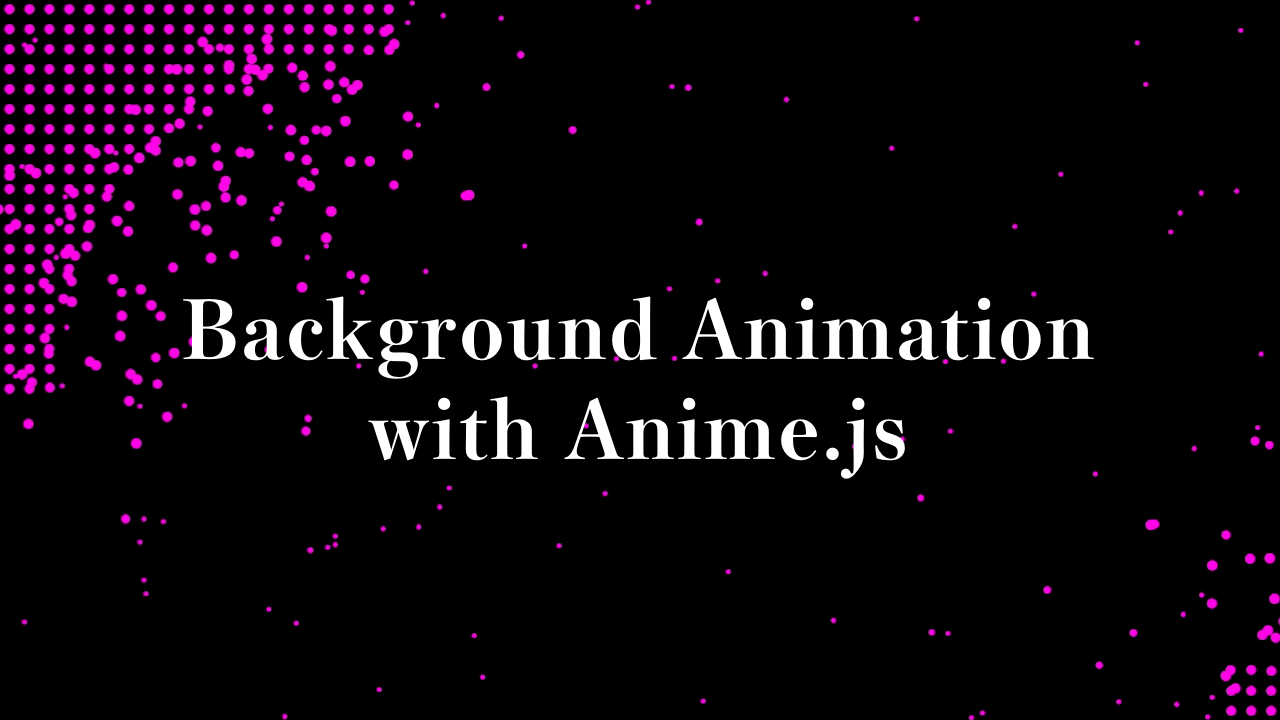
09/23/2021
Contents
Demo
Video
Code
HTML
<h1 class="title">Background<br>Animation<br>with Anime.js</h1>
<div class="bg"></div>
CSS
.title {
position: absolute;
top: 50%;
left: 50%;
opacity: 0;
z-index: 10;
transform: translate(-50%, -50%);
margin: 0;
color: #000;
font-size: 1.25rem;
line-height: 1.8;
text-align: center;
}
.bg {
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
width: 200px;
height: 200px;
}
.el {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #000;
}
JavaScript
const bg = document.querySelector('.bg');
const title = document.querySelector('.title');
const fragment = document.createDocumentFragment();
const grid = [20, 20];
const col = grid[0];
const row = grid[1];
const field = col * row;
for (let i = 0; i < field; i++) {
const div = document.createElement('div');
fragment.appendChild(div);
div.className = 'el';
}
bg.appendChild(fragment);
const BackgroundAnimation = anime.timeline({
targets: '.el',
duration: 1000,
delay: anime.stagger(0, {grid: grid, from: 'first'}),
loop: true,
easing: 'easeInBack',
})
.add({
scale: .5,
backgroundColor: '#ff08e8',
delay: anime.stagger(100, {grid: grid, from: 'last'}),
})
.add({
translateX: () => anime.random(-1000, 1000),
translateY: () => anime.random(-1000, 1000),
scale: .25,
delay: anime.stagger(150, {grid: grid, from: 'last'}),
})
.add({
targets: title,
opacity: 1,
duration: 10,
})
.add({
translateX: 0,
translateY: 0,
scale: 1,
borderRadius: 0,
backgroundColor: '#fff',
duration: 2500,
delay: anime.stagger(150, {grid: grid, from: 'first'}),
endDelay: 1500,
});
BackgroundAnimation.play();