How to Generate a Unique ID in JavaScript
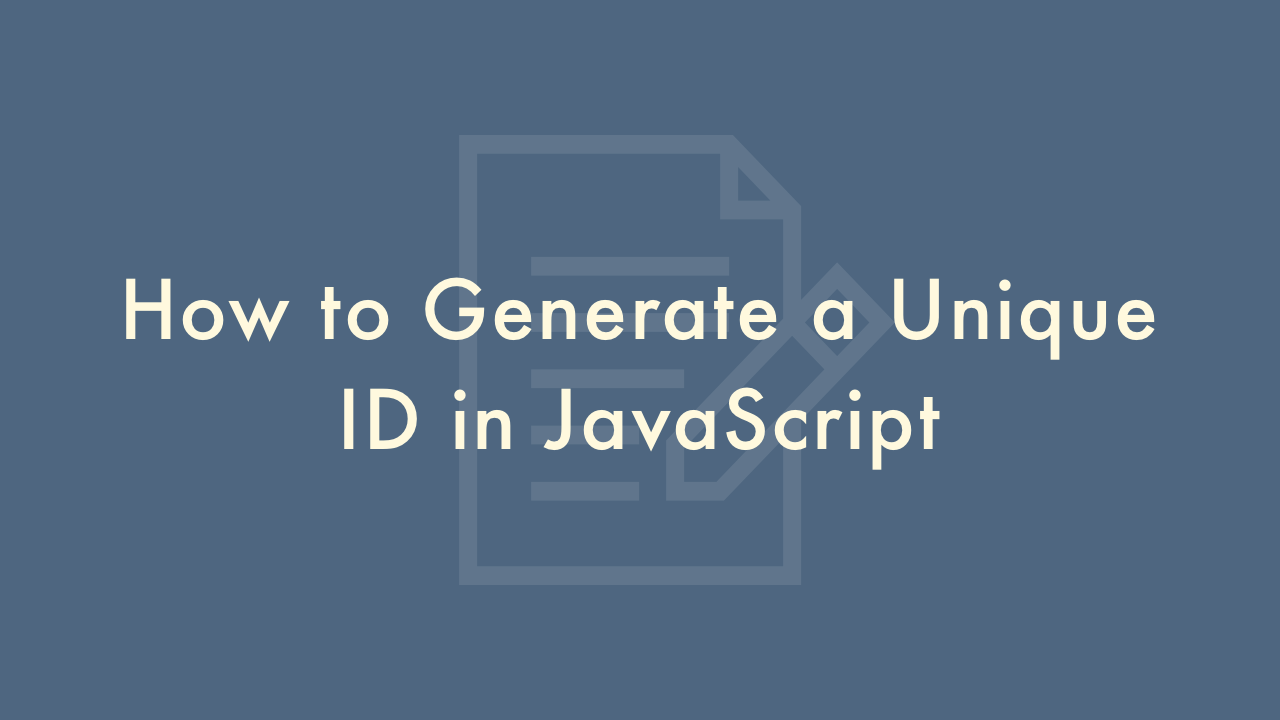
Contents
In this article, you will learn how to generate a unique ID in JavaScript.
Generating a unique ID in JavaScript
Creating a unique ID in JavaScript can be a useful task in many web applications. There are several ways to generate a unique ID in JavaScript, such as using the Date.now() method, generating a random number, or using a library like uuid.
Using Date.now()
The Date.now() method returns the number of milliseconds since January 1, 1970, 00:00:00 UTC. This value can be used as a unique identifier.
Example
const uniqueId = Date.now();
console.log(uniqueId); // output: 1616587501382
Using Math.random()
The Math.random() method returns a random number between 0 and 1. By multiplying this number by a large number and converting it to an integer, we can generate a unique ID.
Example
const uniqueId = Math.floor(Math.random() * 1000000000000);
console.log(uniqueId); // output: 672836583987
Using uuid
uuid is a JavaScript library for generating universally unique identifiers (UUIDs). UUIDs are 128-bit numbers that are unique across space and time. uuid provides several methods for generating UUIDs.
Example
import { v4 as uuidv4 } from 'uuid';
const uniqueId = uuidv4();
console.log(uniqueId); // output: a14322e6-957c-4d2a-8f68-13a47fc4e9fc