The Difference Between Var, Let and Const in JavaScript
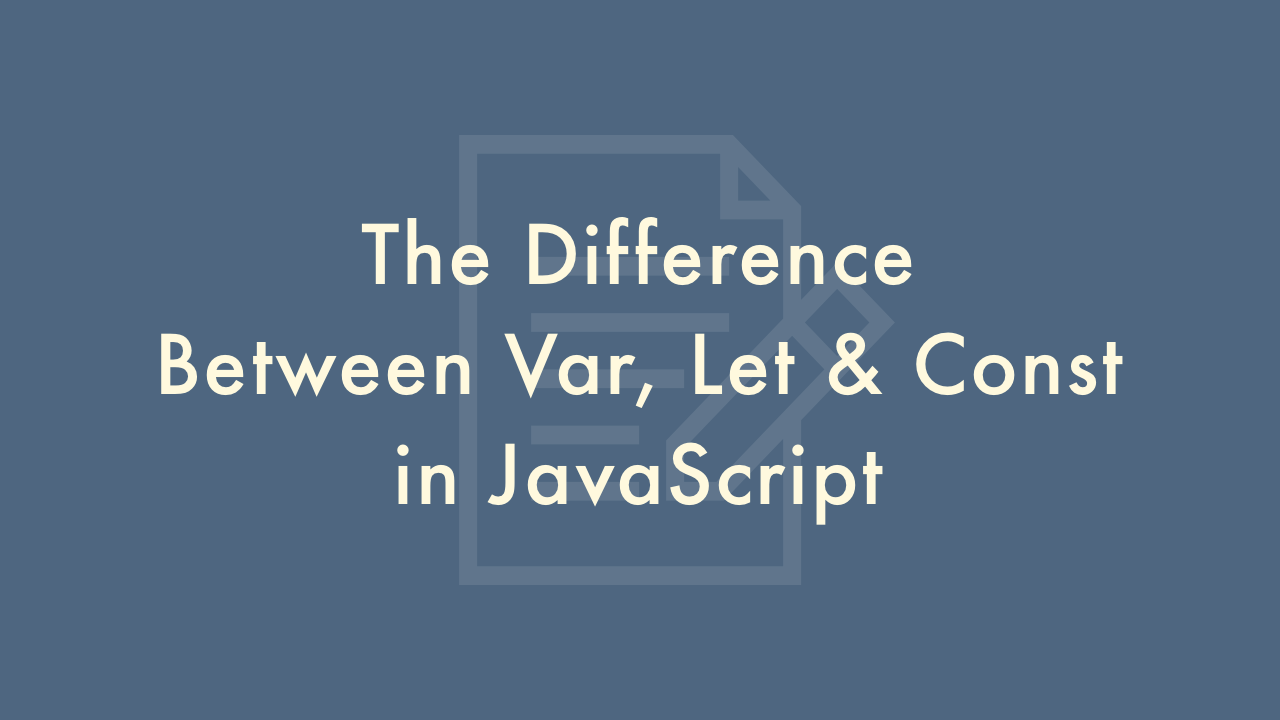
Contents
In this article, you will learn the difference between let
, var
, and const
in JavaScript and how to use them properly.
What are var, let and const?
In JavaScript, it is recommended to declare the variable name when using variables and constants (hereinafter collectively referred to as “variables”).
var
, let
, const
are keywords used when declaring variables in JavaScript.
For example, declare variables by writing:
var name = 'Plantpot';
let name = 'Plantpot';
const name = 'Plantpot';
let
and const
are keywords of the declaration method adopted from ECMAScript 2015 which is a standard specification of JavaScript.
What’s the Difference?
The table below summarizes the difference between var
, let
, and const
.
const | let | var | |
---|---|---|---|
Scope | Block | Block | Function |
Can Be Reassigned? | no | yes | yes |
Can Be Redeclared? | no | no | yes |
Can Be Hoisted? | no | no | yes |
Scope
Declared variables cannot be used anywhere in the code, and this scope defines the range in which they can be used.
var: Function Scope
var
has a wider scope than let
and const
.
Variables declared with var
in a function can be called from anywhere in the function.
function test() {
if () {
// declaration
var a = 1;
}
console.log(a); // expected output: 1
}
let,const: Block Scope
A block is a process enclosed in {}.
Variables declared with let
or const
cannot be called from outside the block, even within the same function.
function test() {
if () {
// declaration
let b = 2;
const c = 3;
}
console.log(b); // undefined
console.log(c); // undefined
};
Basically, it is better to use let
or const
to clarify how the variable is used.
Can Be Reassigned?
Reassignment is to reassign a value to a variable once declared.
var
and let
can be reassigned, but const
cannot.
// var
var a = 1;
a = 2;
console.log(a); // expected output: 2
// let
let b = 1;
b = 2;
console.log(b); // expected output: 2
// const
const c = 1;
c = 2; // Assignment to constant variable.
Use const
as much as possible, because minimizing reassignment will clarify the contents of the variable and implement clear and readable code.
Can Be Redeclared?
Re-declaration is to re-declare the variable name once declared.
Since the declaration is overwritten, re-declaring it will lead to an unexpected bug.
It also reduces the readability of the code and is usually avoided.
Only var
can be redeclared.
// var
var a = 1;
var a = 2;
console.log(a); // expected output: 2
// let
let b = 1;
let b = 2; // SyntaxError: Identifier 'b' has already been declared
// const
const c = 1;
const c = 2; // SyntaxError: Identifier 'c' has already been declared
Can Be Hoisted?
The behavior in which a variable declaration is always made at the beginning of a function is called hoisting (variable hoisting).
Hoisting is possible only for var
.
// var
a = 1;
var a;
console.log(a);
// It is processed as follows.
var a;
a = 1;
console.log(a); //expected output: 1
// let
console.log(b); // ReferenceError: b is not defined
let b = 2;
// const
console.log(c); // ReferenceError: c is not defined
const c = 3;
Proper use of var, let, const
From the conclusion, basically use const
.
In addition to not being able to redeclare or reassign, const
, which has a narrow scope, is the most restrictive, so it is a safe keyword to prevent unintended bugs.
If you can’t use const
, use let
.
For example, use let
when reassignment is required for repetitive processing.
let count = 0;
for () {
count++;
}