Call PHP Script/Function with JavaScript
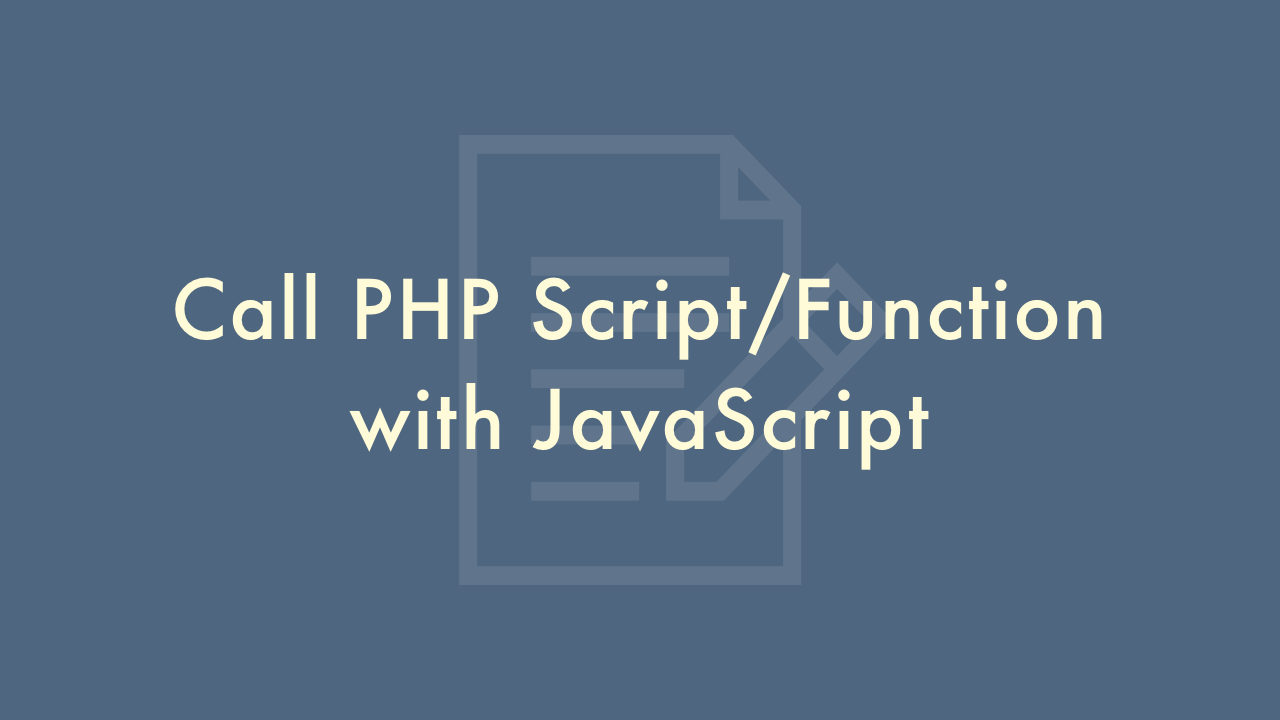
02/02/2022
Contents
In this article, you will learn how to call PHP script/function with JavaScript.
Call PHP script/function from JavaScript
Use the XMLHttpRequest object to call PHP from JavaScript.
For example, to call PHP that returns a JSON object, write as follows.
var request = new XMLHttpRequest();
request.open('GET', URL, true);
request.responseType = 'json';
request.addEventListener('load', function (response) {
// Processing after receiving JSON data
});
request.send();
Call JavaScript script/function from PHP
By writing JavaScript in the PHP file, the result on the PHP side can be reflected in JavaScript.
var data = <?="this.response"?>;
Sample Code
Below is sample code that calls sample1.php from JavaScript in sample2.php using an XMLHttpRequest object.
sample1.php
<?php
$ret = 0;
if(isset($_GET['str'])) {
$str = $_GET['str'];
eval(sprintf('$ret=%s;',$str));
}
$array = array("ans1" => $ret, "ans2" => $ret * $ret);
header("Content-Type: text/javascript; charset=utf-8");
echo json_encode($array);
>
sample2.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>Call PHP Script/Function with JavaScript</title>
</head>
<body>
<div id="ans1"></div>
<div id="ans2"></div>
<script language="javascript" type="text/javascript">
var request = new XMLHttpRequest();
request.open('GET', 'http://plantpot.works/sample1.php?str=100', true);
request.responseType = 'json';
request.addEventListener('load', function (response) {
var data = ="this.response"?>;
console.log("ans1", data.ans1);
document.getElementById("ans1").innerHTML = "ans1=" + data.ans1;
console.log("ans2", data.ans2);
document.getElementById("ans2").innerHTML = "ans2=" + data.ans2;
});
request.send();
</script>
</body>
</html>
You can check the execution result on the console.