How to Convert Radians to Degrees in JavaScript
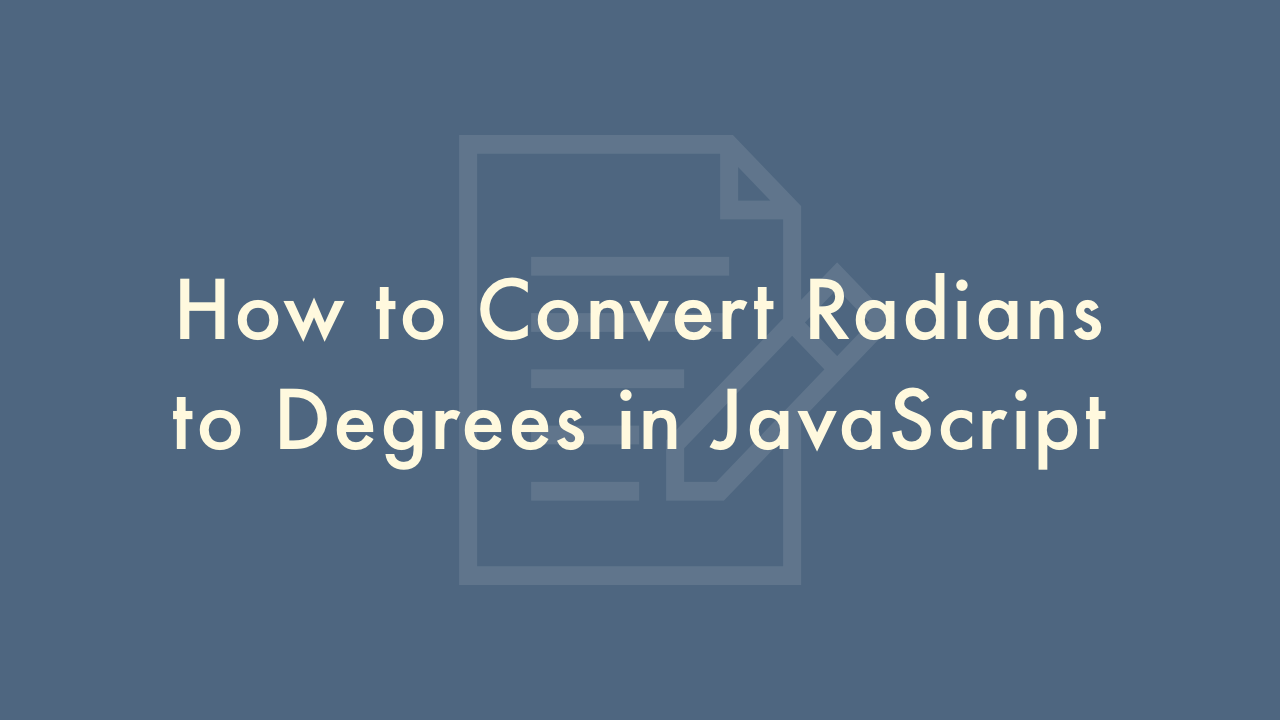
Contents
In this article, you will learn how to convert radians to degrees in JavaScript.
Converting radians to degrees in JavaScript
Radians and degrees are two ways to measure angles. Radians are a more mathematically convenient way to measure angles, but degrees are more commonly used in everyday life. In JavaScript, we can convert radians to degrees using a simple formula.
The formula to convert radians to degrees is:
degrees = radians * (180 / Math.PI)
where radians is the angle measurement in radians and Math.PI is the mathematical constant for pi, which is approximately equal to 3.14159.
Examples
Convert 0.7854 radians to degrees
const radians = 0.7854;
const degrees = radians * (180 / Math.PI);
console.log(degrees); // 45
In this example, we first define the angle measurement radians to be 0.7854. We then use the formula to convert radians to degrees and store the result in the variable degrees. The output is the value of degrees, which is approximately equal to 45 degrees.
Convert 1.5708 radians to degrees
const radians = 1.5708;
const degrees = radians * (180 / Math.PI);
console.log(degrees); // 90
In this example, we define the angle measurement radians to be 1.5708. We then use the formula to convert radians to degrees and store the result in the variable degrees. The output is the value of degrees, which is approximately equal to 90 degrees.
Convert 3.1416 radians to degrees
const radians = 3.1416;
const degrees = radians * (180 / Math.PI);
console.log(degrees); // 180
In this example, we define the angle measurement radians to be 3.1416. We then use the formula to convert radians to degrees and store the result in the variable degrees. The output is the value of degrees, which is approximately equal to 180 degrees.