StopWatch with HTML, CSS & JavaScript
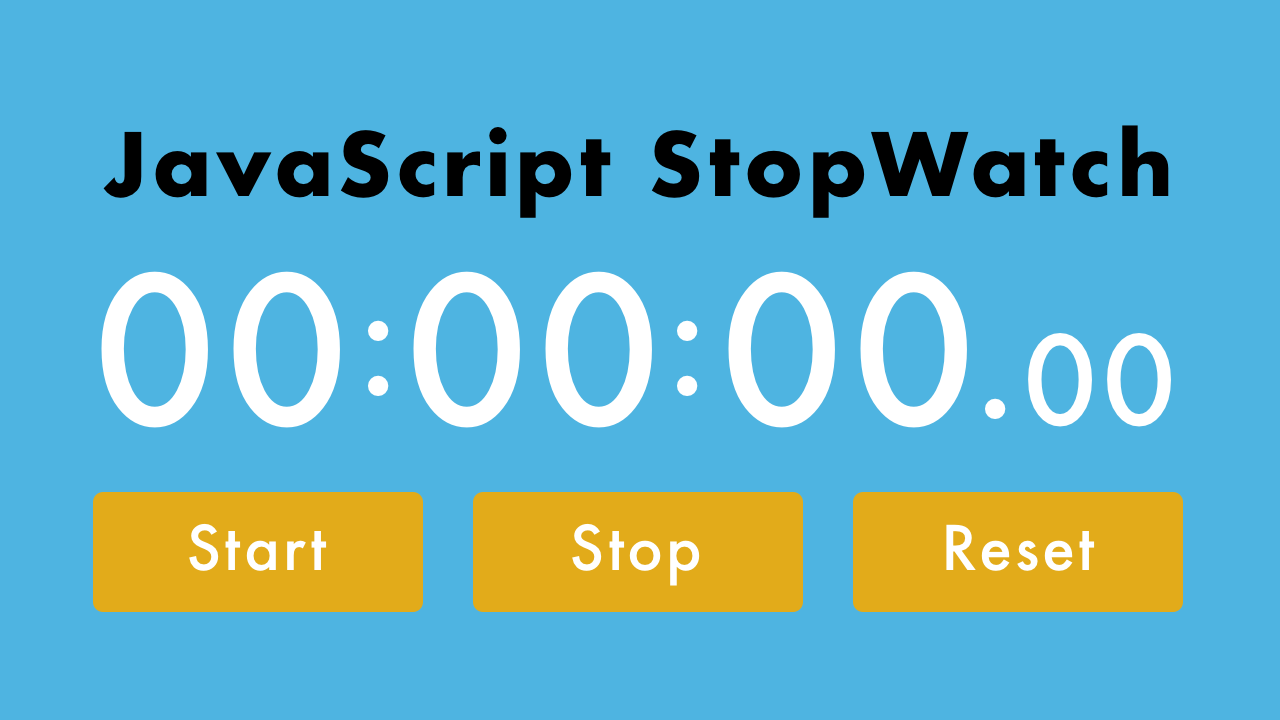
01/16/2021
Contents
Demo
Video
Code
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<title>StopWatch with HTML, CSS & JavaScript</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="box">
<div class="stopwatch">
<span class="hours">00</span>
<span>:</span>
<span class="minutes">00</span>
<span>:</span>
<span class="seconds">00</span>
<span>.</span>
<span class="milliseconds">00</span>
</div>
<div class="buttons">
<button class="btn start" onclick="startTimer()">Start</button>
<button class="btn" onclick="stopTimer()">Stop</button>
<button class="btn" onclick="resetTimer()">Reset</button>
</div>
</div>
<script src="stopwatch.js"></script>
</body>
</html>
CSS
@charset "utf-8";
@import url('https://fonts.googleapis.com/css2?family=Roboto:wght@400&display=swap');
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
html {
font-family: 'Roboto', sans-serif;
font-size: 16px;
}
body {
display: flex;
justify-content: center;
align-items: center;
width: 100%;
height: 100vh;
background-color: #4eb4e1;
}
.box {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
width: 100%;
max-width: 400px;
padding: 10px;
}
.stopwatch {
display: flex;
justify-content: space-between;
align-items: baseline;
width: 100%;
color: #fff;
font-size: 4.5rem;
}
.milliseconds {
font-size: 3rem;
}
.buttons {
display: flex;
justify-content: space-between;
align-items: center;
width: 100%;
margin-top: 10px;
}
.btn {
width: 30%;
padding: 10px 0;
border: none;
border-radius: 4px;
background-color: #e2ab1a;
color: #fff;
font-size: 1.25rem;
font-family: inherit;
outline: none;
cursor: pointer;
}
.btn:disabled {
background-color: #ccc;
cursor: default;
}
@media screen and (max-width: 480px) {
.stopwatch {
font-size: 2.75rem;
}
.milliseconds {
font-size: 2rem;
}
}
JavaScript
let hr = min = sec = ms = 0;
let t;
let startBtn = document.querySelector(".start");
function countUp() {
ms++;
if (ms == 100) {
sec++;
ms = 0;
}
if (sec == 60) {
min++;
sec = 0;
}
if (min == 60) {
hr++;
min = 0;
}
displayTimer();
}
function displayTimer() {
document.querySelector(".hours").innerHTML = ("0" + hr).slice(-2);
document.querySelector(".minutes").innerHTML = ("0" + min).slice(-2);
document.querySelector(".seconds").innerHTML = ("0" + sec).slice(-2);
document.querySelector(".milliseconds").innerHTML = ("0" + ms).slice(-2);
}
function startTimer() {
startBtn.disabled = true;
t = setInterval(countUp, 10);
}
function stopTimer() {
clearInterval(t);
startBtn.disabled = false;
}
function resetTimer() {
clearInterval(t);
hr = min = sec = ms = 0;
displayTimer();
startBtn.disabled = false;
}