How to Use the if…else Statement in JavaScript
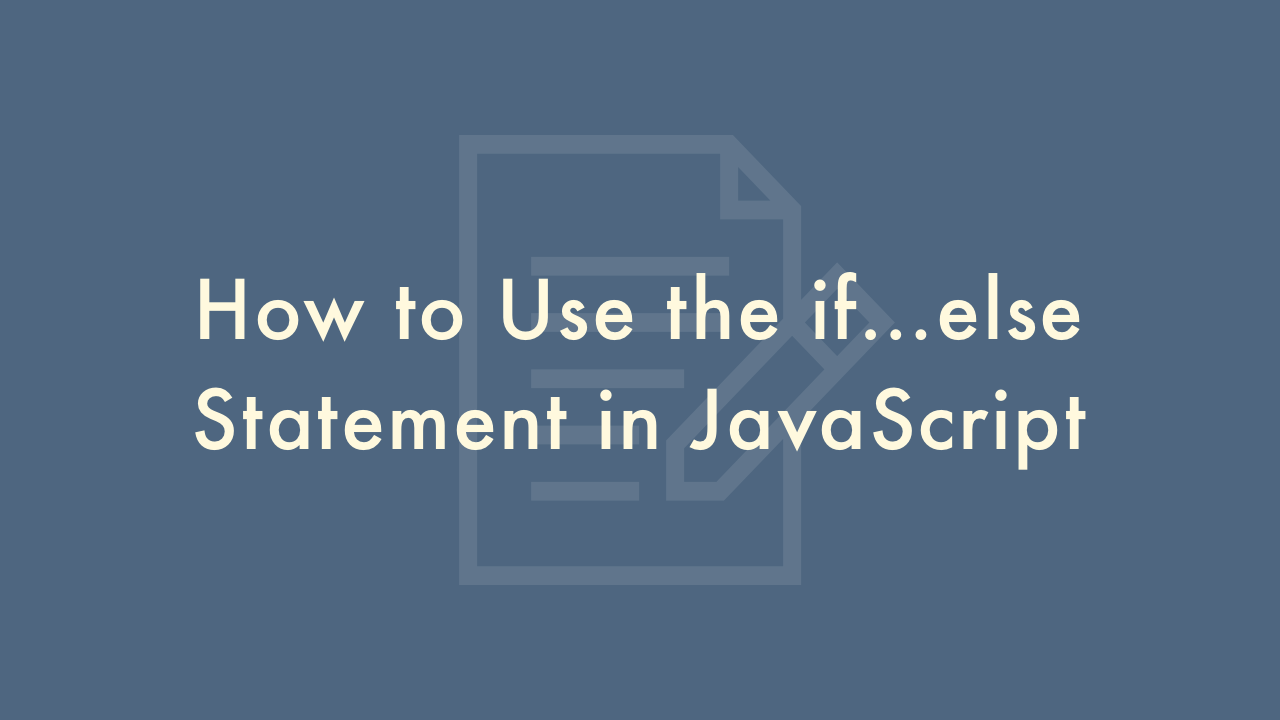
Contents
In this article, you will learn how to use the if…else statement in JavaScript.
Using the if…else statement in JavaScript
The if…else statement is a control structure in JavaScript that allows you to execute different blocks of code depending on whether a condition is true or false. This is a fundamental building block of programming, as it allows you to write code that can make decisions based on input or other factors.
Syntax
Here is the basic syntax for an if…else statement:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
Example
Here is an example of an if…else statement that checks whether a number is even or odd:
let num = 4;
if (num % 2 === 0) {
console.log(num + " is even");
} else {
console.log(num + " is odd");
}
In this example, the if…else statement checks whether num is evenly divisible by 2. If it is, the first block of code will be executed, which prints out that the number is even. If it is not, the second block of code will be executed, which prints out that the number is odd.
Tips for working with if…else statements
Here are some tips for working with if…else statements in JavaScript:
- Be sure to use double equal signs (==) or triple equal signs (===) to check for equality in your conditions. Double equal signs will perform type coercion, which can lead to unexpected results, so it is generally recommended to use triple equal signs instead.
- You can use multiple else if statements to check for multiple conditions.
- Be sure to consider all possible cases when writing your conditions. For example, if you are checking whether a number is positive, you should also check whether it is zero or negative.
- You can nest if…else statements to create more complex logic.