How to Use the Ruby reverse Method
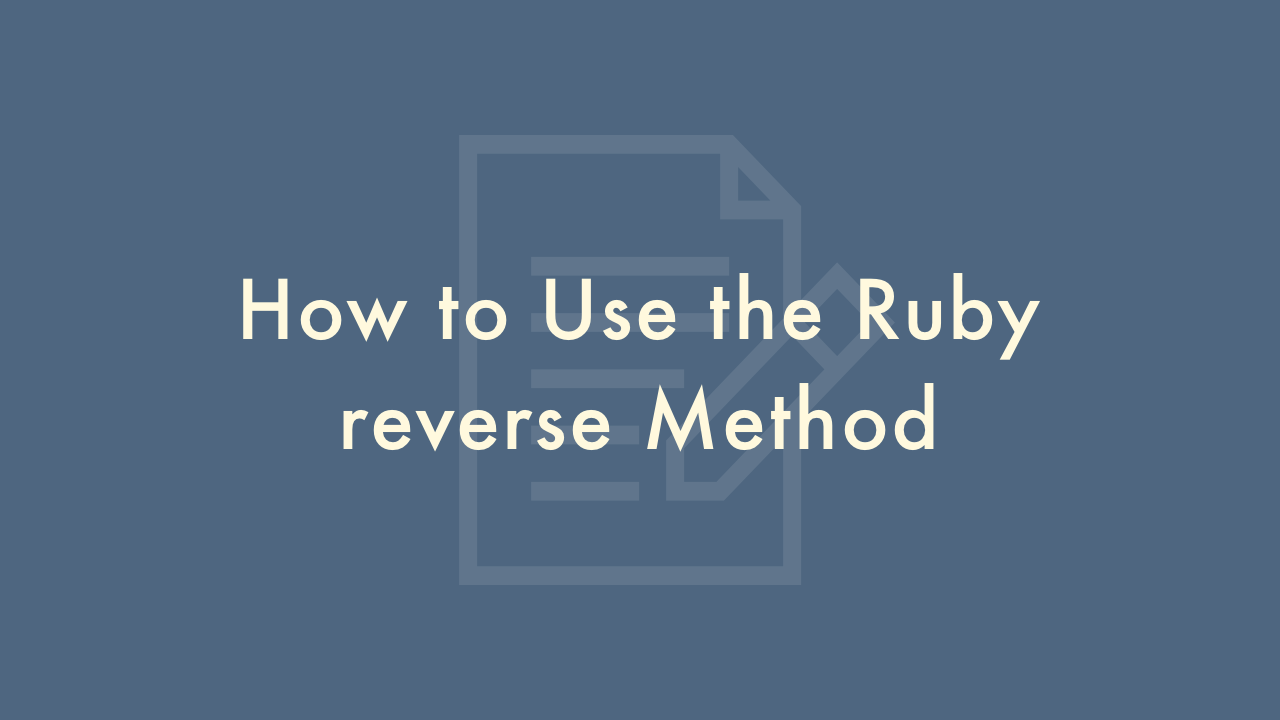
Contents
In this article, you will learn how to use the Ruby reverse method.
Using the reverse method in Ruby
The reverse method is a built-in method in Ruby that can be called on any array, string or range object. It takes no arguments and returns a new object with the elements in reverse order. The original object is not modified by the reverse method.
Here is the basic syntax of using the reverse method:
array.reverse
string.reverse
range.to_a.reverse
As you can see, the method is called on the object that you want to reverse, and the result is returned as a new object.
Using the reverse method with Arrays
In Ruby, you can use the reverse method with arrays to reverse the order of elements in the array. Here is an example:
fruits = ["apple", "banana", "orange", "kiwi"]
reversed_fruits = fruits.reverse
puts reversed_fruits.inspect
In this example, the reverse method is called on the fruits array, and the resulting reversed array is stored in the reversed_fruits variable. The inspect method is used to print the contents of the array to the console. The output of this program would be:
["kiwi", "orange", "banana", "apple"]
As you can see, the reverse method has reversed the order of elements in the fruits array.
Using the reverse method with Strings
You can also use the reverse method with strings in Ruby. Here is an example:
str = "Hello, World!"
reversed_str = str.reverse
puts reversed_str
In this example, the reverse method is called on the str string, and the resulting reversed string is stored in the reversed_str variable. The puts method is used to print the reversed string to the console. The output of this program would be:
!dlroW ,olleH
As you can see, the reverse method has reversed the order of characters in the str string.
Using the reverse method with Ranges
You can also use the reverse method with ranges in Ruby. Here is an example:
numbers = 1..5
reversed_numbers = numbers.to_a.reverse
puts reversed_numbers.inspect
In this example, the reverse method is called on the numbers range, and the resulting reversed array is stored in the reversed_numbers variable. The to_a method is used to convert the range to an array, and the inspect method is used to print the contents of the array to the console. The output of this program would be:
[5, 4, 3, 2, 1]
As you can see, the reverse method has reversed the order of elements in the numbers range.