How to Generate a Random String in Ruby
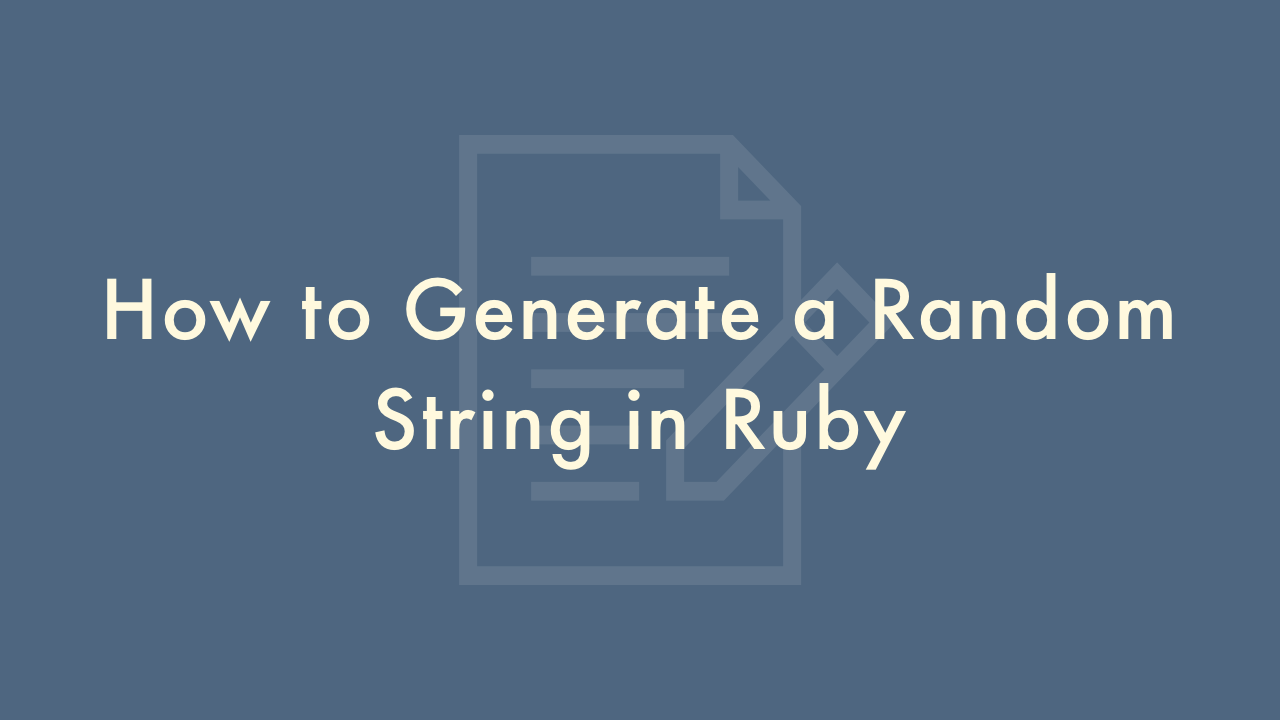
Contents
In this article, you will learn how to generate a random string in Ruby.
Generating a random string
Generating a random string in Ruby can be accomplished in many ways, here are a few methods:
Using SecureRandom
One of the simplest and most reliable ways to generate a random string in Ruby is to use the SecureRandom library. Here’s an example code:
require 'securerandom'
random_string = SecureRandom.hex(10)
This will generate a random string of 10 characters using hexadecimal encoding.
Using Array of Characters
Another way to generate a random string is by creating an array of characters, and then using the sample method to select a random element from the array:
characters = ('a'..'z').to_a + ('A'..'Z').to_a + (0..9).to_a
random_string = (0...10).map { characters.sample }.join
This will generate a random string of 10 characters using a combination of lowercase and uppercase letters, as well as digits.
Using rand and ASCII Values
A third way to generate a random string is by using the rand method with ASCII values to generate a random sequence of characters:
random_string = (0...10).map { (rand(65..90)).chr }.join
This will generate a random string of 10 uppercase letters using ASCII values.
Using Base64 Encoding
Another approach to generating a random string is to use Base64 encoding. Here’s an example code:
require 'base64'
random_string = Base64.urlsafe_encode64(SecureRandom.random_bytes(10))
This will generate a random string of 10 bytes and encode it using Base64, resulting in a string of approximately 14 characters in length.
Using UUID
Finally, if you need to generate a unique identifier, you can use the uuidtools gem to generate a UUID:
require 'uuidtools'
random_string = UUIDTools::UUID.random_create.to_s
This will generate a random UUID string, which is a unique identifier that is 36 characters long.