How to Get IP Address in Python
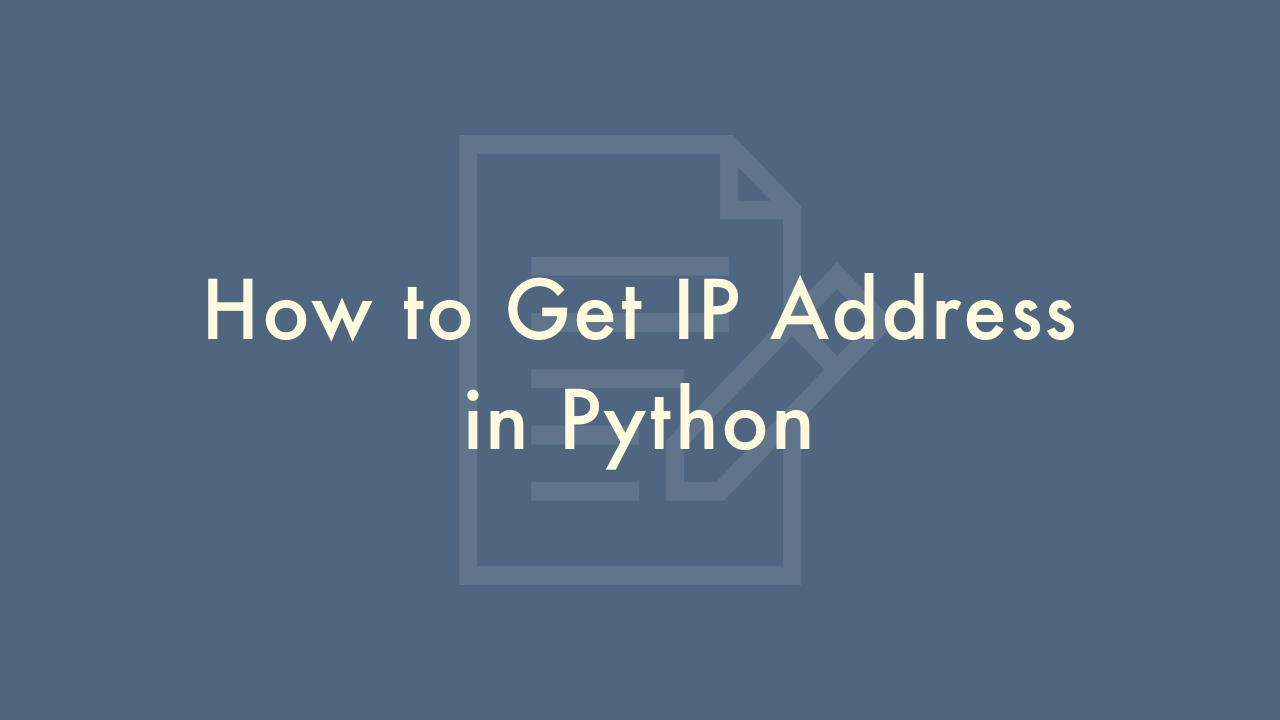
Contents
In this article, you will learn how to Get IP address in Python.
Get IP Address in Python
In Python, you can retrieve your own IP address or the IP address of a remote server using the built-in socket library. Here’s an example of how to do it:
import socket
# To get the IP address of the current machine:
ip_address = socket.gethostbyname(socket.gethostname())
print("IP Address:", ip_address)
# To get the IP address of a remote server:
remote_host = "www.google.com"
ip_address = socket.gethostbyname(remote_host)
print("IP Address of", remote_host, "is:", ip_address)
In the first example, we’re using the gethostname function to get the name of the current machine, and then passing that to the gethostbyname function to get the IP address.
In the second example, we’re specifying the hostname of a remote server (in this case, Google’s website), and using the gethostbyname function to get the IP address of that server.
Here’s some additional information about working with IP addresses in Python:
socket.getfqdn()
This function returns the fully qualified domain name (FQDN) of the current machine. The FQDN includes both the hostname and the domain name, separated by dots.
socket.gethostbyaddr(ip_address)
This function returns a tuple containing the hostname, alias list, and IP address corresponding to the given IP address. If the IP address is not resolvable, the function raises a socket.herror exception.
socket.gethostbyname_ex(hostname)
This function returns a tuple containing the canonical hostname, alias list, and IP address list corresponding to the given hostname. If the hostname is not resolvable, the function raises a socket.herror exception.
socket.inet_aton(ip_address) and socket.inet_ntoa(packed_ip_address)
These functions convert between a 32-bit packed binary format and the standard “dotted-quad” IP address format. inet_aton converts an IP address string to a packed binary format, and inet_ntoa converts a packed binary format to an IP address string.
socket.create_connection(address, timeout=None, source_address=None)
This function creates a TCP/IP socket connection to the specified address. The address argument should be a tuple containing the IP address and port number to connect to.
socket.timeout
This is an exception class that is raised when a socket operation times out. You can catch this exception to handle network timeouts in your code.
These are just a few of the many functions and features available in the socket library for working with IP addresses and network connections in Python.