How to Use the Ruby inject Method
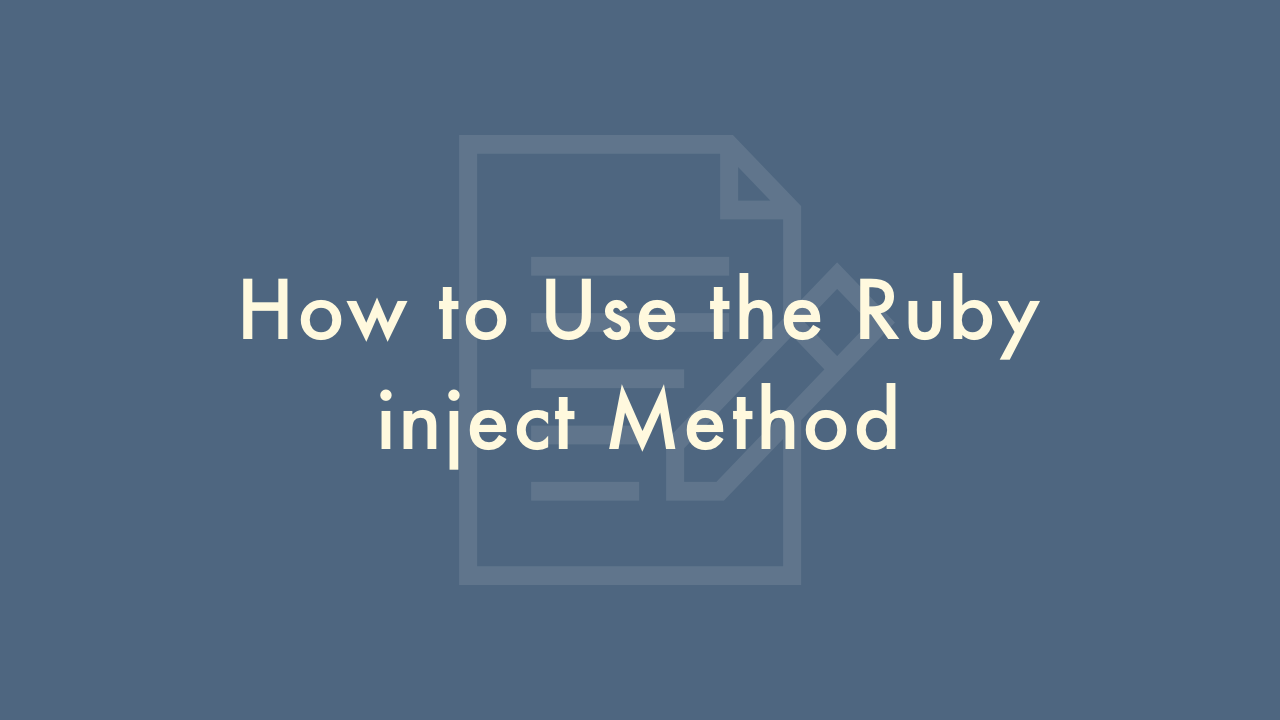
Contents
In this article, you will learn how to use the Ruby inject method.
Using the inject method
The Ruby inject method (also known as reduce) is a powerful way to iterate over an enumerable collection and accumulate a single result. It can be used with a block or a symbol that represents a method name, and it’s often used for summing or multiplying values, finding the maximum or minimum, or concatenating strings.
Syntax
The basic syntax of the inject method is:
collection.inject(initial_value) { |accumulator, element| block }
Parameters
collection
: The enumerable object that you want to iterate over.initial_value
: The initial value of the accumulator (optional).block
: The code that you want to execute on each element of the collection.accumulator
: The variable holds the result of the previous iteration.element
: The current element of the collection.
Examples
Here’s an example of using inject to sum an array of numbers:
numbers = [1, 2, 3, 4, 5]
sum = numbers.inject(0) { |result, n| result + n }
puts sum # => 15
In this example, we start with an initial value of 0 for the accumulator (result), and we add each element of the numbers array to it. The final value of the accumulator is the sum of all the numbers in the array.
You can also use a symbol that represents a method name instead of a block. This can make the code shorter and more readable. For example:
numbers = [1, 2, 3, 4, 5]
sum = numbers.inject(:+)
puts sum # => 15
Here, we’re using the :+ symbol, which represents the + method. This is equivalent to using a block that adds the elements together.
Another common use case for inject is finding the maximum or minimum value in a collection:
numbers = [1, 2, 3, 4, 5]
max = numbers.inject { |a, b| a > b ? a : b }
puts max # => 5
In this example, we’re comparing each element of the array to the previous maximum value (a), and returning the larger of the two. The final value of a is the maximum value in the array.
You can also use inject to concatenate strings:
words = ["foo", "bar", "baz"]
result = words.inject("") { |sentence, word| sentence + word + " " }
puts result.strip # => "foo bar baz"
In this example, we’re starting with an empty string as the initial value of the accumulator, and adding each word in the array to it, separated by a space. The strip method removes the trailing space from the result.