How to Read and Write Files in Ruby on Rails
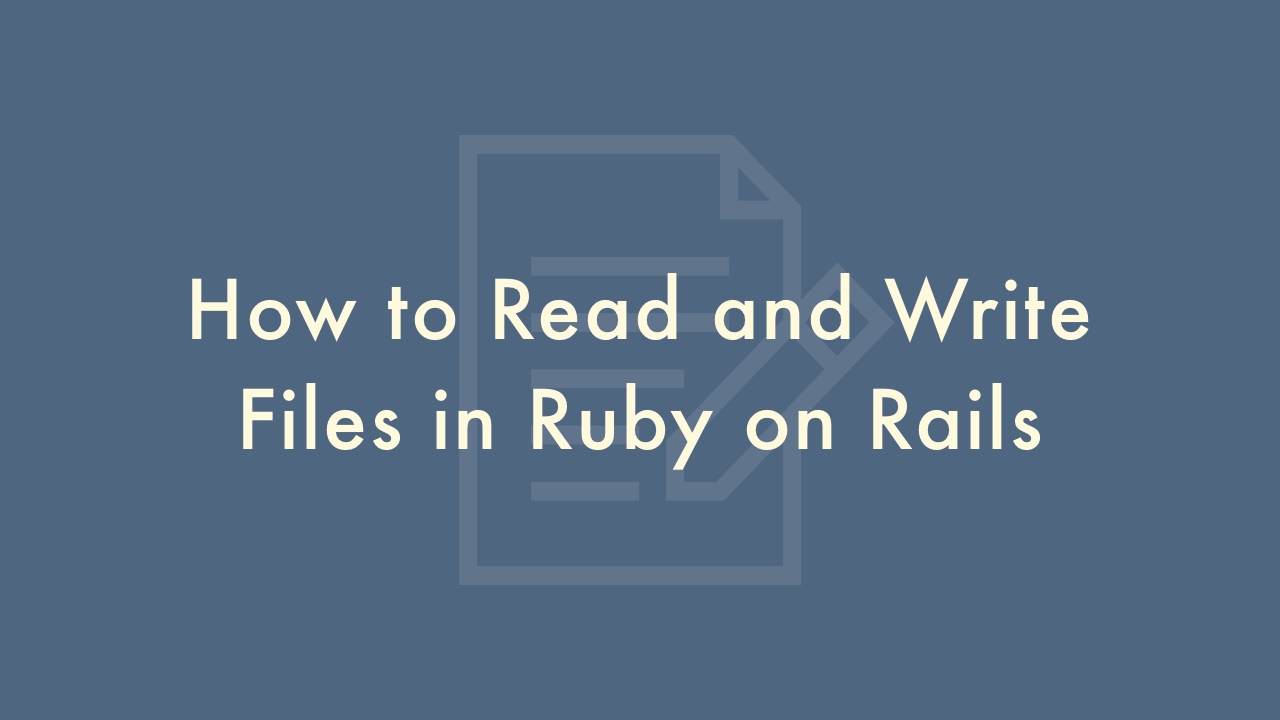
Contents
In this article, you will learn how to read and write files in Ruby on Rails.
Reading and writing files
Reading and writing files in Ruby on Rails is very similar to standard Ruby. Here are some ways to do it:
Reading Files
Reading an Entire File
To read an entire file, you can use the File.read method. Here’s an example:
contents = File.read('/path/to/file')
This will read the entire file at the given path and return its contents as a string.
Reading a File Line by Line
To read a file line by line, you can use the File.foreach method. Here’s an example:
File.foreach('/path/to/file') do |line|
puts line
end
This will read each line of the file at the given path and print it to the console.
Writing Files
Writing to a File
To write to a file, you can use the File.write method. Here’s an example:
File.write('/path/to/file', 'This is some text that will be written to the file.')
This will write the given text to the file at the given path. If the file doesn’t exist, it will be created.
Appending to a File
To append text to a file, you can use the File.open method with the ‘a’ mode. Here’s an example:
File.open('/path/to/file', 'a') do |file|
file.puts 'This text will be appended to the file.'
end
This will open the file at the given path in append mode, write the given text to the file, and then close the file. If the file doesn’t exist, it will be created.
Note that in both cases, you need to specify the full path to the file. You can also use relative paths if the file is in the same directory as your Rails application, or in a subdirectory of your Rails application.